csObjectModel Class Reference
[Geometry utilities]
Helper class to make it easier to implement iObjectModel in mesh objects.
More...
#include <cstool/objmodel.h>
Inheritance diagram for csObjectModel:
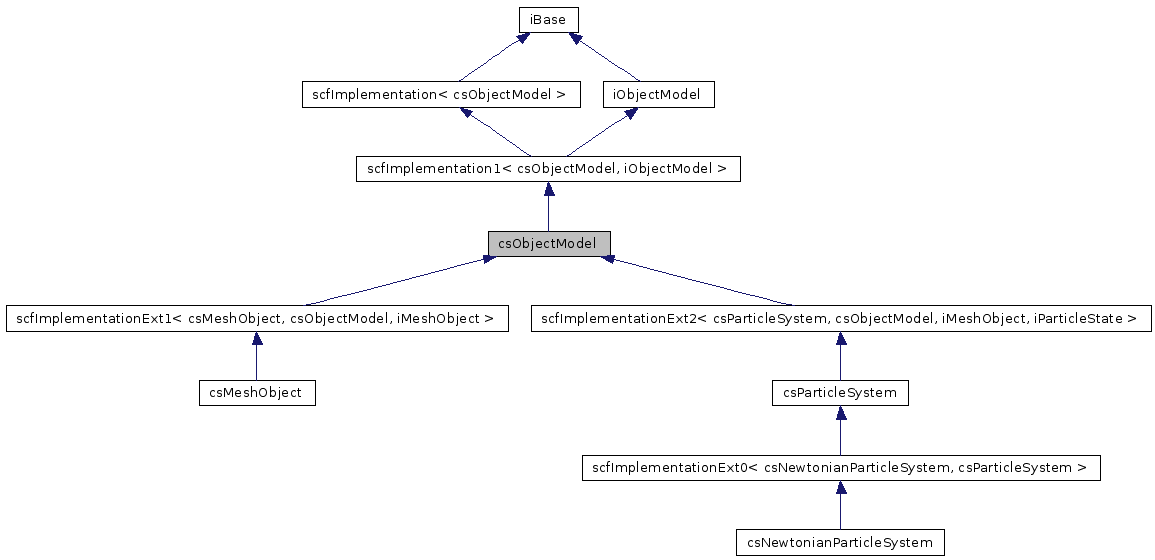
Public Member Functions | |
virtual void | AddListener (iObjectModelListener *listener) |
Add a listener to this object model. | |
virtual csPtr< iPolygonMesh > | CreateLowerDetailPolygonMesh (float) |
Create a polygon mesh representing a lower detail version of the object but without the restrictions of GetPolygonMeshViscull(). | |
csObjectModel (iBase *parent=0) | |
Construct a new csObjectModel. | |
void | FireListeners () |
Fire all listeners. | |
virtual iPolygonMesh * | GetPolygonMeshBase () |
Get a polygon mesh representing the basic geometry of the object. | |
virtual iPolygonMesh * | GetPolygonMeshColldet () |
Get a polygon mesh representing the geometry of the object. | |
virtual iPolygonMesh * | GetPolygonMeshShadows () |
Get a polygon mesh specifically for shadow casting (to be used by the shadow manager). | |
virtual iPolygonMesh * | GetPolygonMeshViscull () |
Get a polygon mesh specifically for visibility culling (to be used as an occluder). | |
virtual long | GetShapeNumber () const |
Returns a number that will change whenever the shape of this object changes. | |
virtual iTerraFormer * | GetTerraFormerColldet () |
Get a terra former representing the geometry of the object. | |
virtual void | RemoveListener (iObjectModelListener *listener) |
Remove a listener from this object model. | |
void | SetPolygonMeshBase (iPolygonMesh *base) |
Set the pointer to the base polygon mesh. | |
virtual void | SetPolygonMeshColldet (iPolygonMesh *polymesh) |
Set a polygon mesh representing the geometry of the object. | |
virtual void | SetPolygonMeshShadows (iPolygonMesh *polymesh) |
Set a polygon mesh representing the geometry of the object. | |
virtual void | SetPolygonMeshViscull (iPolygonMesh *polymesh) |
Set a polygon mesh representing the geometry of the object. | |
void | SetShapeNumber (long n) |
Set the shape number manually (should not be needed in most cases). | |
void | ShapeChanged () |
Increase the shape number and also fire all listeners. | |
virtual | ~csObjectModel () |
Detailed Description
Helper class to make it easier to implement iObjectModel in mesh objects.This class does not implement the bounding box and radius functions.
Definition at line 45 of file objmodel.h.
Constructor & Destructor Documentation
csObjectModel::csObjectModel | ( | iBase * | parent = 0 |
) | [inline] |
Construct a new csObjectModel.
Don't forget to call SetPolygonMesh<xxx>()!
Definition at line 60 of file objmodel.h.
Member Function Documentation
virtual void csObjectModel::AddListener | ( | iObjectModelListener * | listener | ) | [inline, virtual] |
Add a listener to this object model.
This listener will be called whenever the object model changes or right before it is destroyed.
Implements iObjectModel.
Definition at line 123 of file objmodel.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Push(), and RemoveListener().
virtual csPtr<iPolygonMesh> csObjectModel::CreateLowerDetailPolygonMesh | ( | float | ) | [inline, virtual] |
Create a polygon mesh representing a lower detail version of the object but without the restrictions of GetPolygonMeshViscull().
The floating point input number is 0 for minimum detail and 1 for highest detail. This function may return the same polygon mesh as GetPolygonMeshColldet() (but with ref count incremented by one). Can return 0 if this object model doesn't support that.
Implements iObjectModel.
Definition at line 119 of file objmodel.h.
void csObjectModel::FireListeners | ( | ) | [inline] |
Fire all listeners.
Definition at line 95 of file objmodel.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Length().
Referenced by ShapeChanged().
virtual iPolygonMesh* csObjectModel::GetPolygonMeshBase | ( | ) | [inline, virtual] |
Get a polygon mesh representing the basic geometry of the object.
Can return 0 if this object model doesn't support that.
Implements iObjectModel.
Definition at line 103 of file objmodel.h.
virtual iPolygonMesh* csObjectModel::GetPolygonMeshColldet | ( | ) | [inline, virtual] |
Get a polygon mesh representing the geometry of the object.
This mesh is useful for collision detection. Can return 0 if this object model doesn't support that.
Implements iObjectModel.
Definition at line 104 of file objmodel.h.
virtual iPolygonMesh* csObjectModel::GetPolygonMeshShadows | ( | ) | [inline, virtual] |
Get a polygon mesh specifically for shadow casting (to be used by the shadow manager).
This polygon mesh is guaranteed to be smaller or equal to the real object. In other words: if you would render the original mesh in red and this one in blue you should not see any blue anywhere. Can return 0 if this object model doesn't support that. In that case the object will not be used for shadow casting.
Implements iObjectModel.
Definition at line 114 of file objmodel.h.
virtual iPolygonMesh* csObjectModel::GetPolygonMeshViscull | ( | ) | [inline, virtual] |
Get a polygon mesh specifically for visibility culling (to be used as an occluder).
This polygon mesh is guaranteed to be smaller or equal to the real object. In other words: if you would render the original mesh in red and this one in blue you should not see any blue anywhere. This kind of lower detail version can be used for occlusion writing in a visibility culling system. Can return 0 if this object model doesn't support that. In that case the object will not be used for visibility culling.
Implements iObjectModel.
Definition at line 109 of file objmodel.h.
virtual long csObjectModel::GetShapeNumber | ( | ) | const [inline, virtual] |
Returns a number that will change whenever the shape of this object changes.
If that happens then the data in all the returned polygon meshes and bounding volumes will be invalid.
Implements iObjectModel.
Definition at line 102 of file objmodel.h.
virtual iTerraFormer* csObjectModel::GetTerraFormerColldet | ( | ) | [inline, virtual] |
Get a terra former representing the geometry of the object.
This class is useful for collision detection. Can return 0 if this object model doesn't support that.
Implements iObjectModel.
Reimplemented in csParticleSystem, and csMeshObject.
Definition at line 132 of file objmodel.h.
virtual void csObjectModel::RemoveListener | ( | iObjectModelListener * | listener | ) | [inline, virtual] |
Remove a listener from this object model.
Implements iObjectModel.
Definition at line 128 of file objmodel.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Delete().
Referenced by AddListener().
void csObjectModel::SetPolygonMeshBase | ( | iPolygonMesh * | base | ) | [inline] |
virtual void csObjectModel::SetPolygonMeshColldet | ( | iPolygonMesh * | polymesh | ) | [inline, virtual] |
Set a polygon mesh representing the geometry of the object.
This mesh is useful for collision detection. This can be used to replace the default polygon mesh returned by GetPolygonMeshColldet() with one that has less detail or even to support polygon mesh for mesh objects that otherwise don't support it. The object model will keep a reference to the given polymesh.
Implements iObjectModel.
Definition at line 105 of file objmodel.h.
virtual void csObjectModel::SetPolygonMeshShadows | ( | iPolygonMesh * | polymesh | ) | [inline, virtual] |
Set a polygon mesh representing the geometry of the object.
This mesh is useful for shadow casting. This can be used to replace the default polygon mesh returned by GetPolygonMeshShadows() with one that has less detail or even to support polygon mesh for mesh objects that otherwise don't support it. The object model will keep a reference to the given polymesh.
Implements iObjectModel.
Definition at line 115 of file objmodel.h.
virtual void csObjectModel::SetPolygonMeshViscull | ( | iPolygonMesh * | polymesh | ) | [inline, virtual] |
Set a polygon mesh representing the geometry of the object.
This mesh is useful for visibility culling. This can be used to replace the default polygon mesh returned by GetPolygonMeshViscull() with one that has less detail or even to support polygon mesh for mesh objects that otherwise don't support it. The object model will keep a reference to the given polymesh.
Implements iObjectModel.
Definition at line 110 of file objmodel.h.
void csObjectModel::SetShapeNumber | ( | long | n | ) | [inline] |
Set the shape number manually (should not be needed in most cases).
Definition at line 87 of file objmodel.h.
void csObjectModel::ShapeChanged | ( | ) | [inline] |
Increase the shape number and also fire all listeners.
Definition at line 78 of file objmodel.h.
References FireListeners().
The documentation for this class was generated from the following file:
- cstool/objmodel.h
Generated for Crystal Space by doxygen 1.4.7