iObjectModel Struct Reference
[Geometry utilities]
This interface represents data related to some geometry in object space.
More...
#include <imesh/objmodel.h>
Inheritance diagram for iObjectModel:
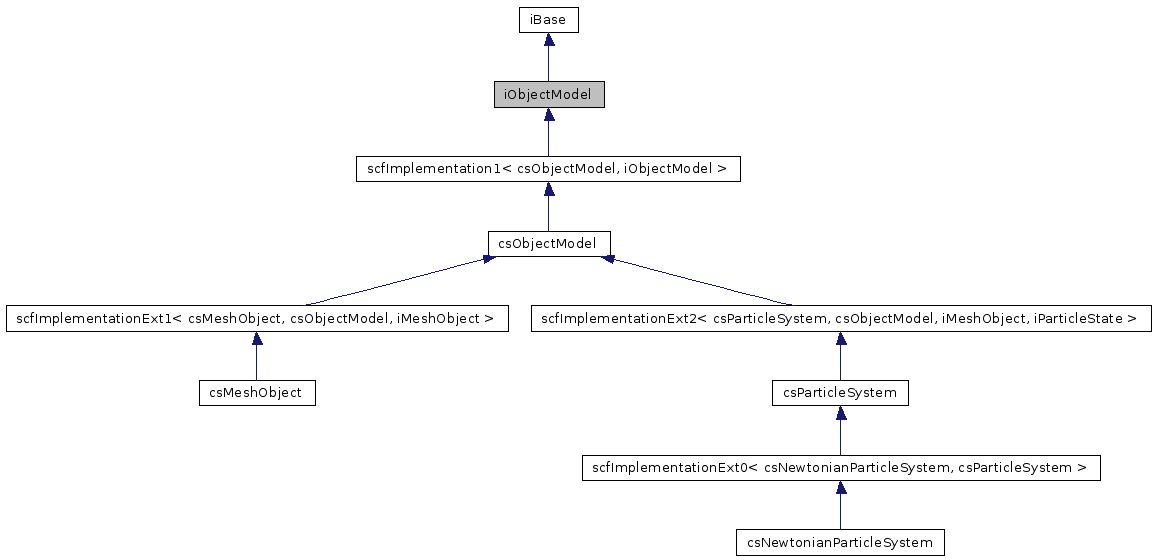
Public Member Functions | |
virtual void | AddListener (iObjectModelListener *listener)=0 |
Add a listener to this object model. | |
virtual csPtr< iPolygonMesh > | CreateLowerDetailPolygonMesh (float detail)=0 |
Create a polygon mesh representing a lower detail version of the object but without the restrictions of GetPolygonMeshViscull(). | |
virtual const csBox3 & | GetObjectBoundingBox ()=0 |
Get the bounding box in object space for this mesh object. | |
virtual void | GetObjectBoundingBox (csBox3 &bbox)=0 |
Get the bounding box in object space for this mesh object. | |
virtual iPolygonMesh * | GetPolygonMeshBase ()=0 |
Get a polygon mesh representing the basic geometry of the object. | |
virtual iPolygonMesh * | GetPolygonMeshColldet ()=0 |
Get a polygon mesh representing the geometry of the object. | |
virtual iPolygonMesh * | GetPolygonMeshShadows ()=0 |
Get a polygon mesh specifically for shadow casting (to be used by the shadow manager). | |
virtual iPolygonMesh * | GetPolygonMeshViscull ()=0 |
Get a polygon mesh specifically for visibility culling (to be used as an occluder). | |
virtual void | GetRadius (float &radius, csVector3 ¢er)=0 |
Get the radius and center of this object in object space. | |
virtual long | GetShapeNumber () const =0 |
Returns a number that will change whenever the shape of this object changes. | |
virtual iTerraFormer * | GetTerraFormerColldet ()=0 |
Get a terra former representing the geometry of the object. | |
virtual void | RemoveListener (iObjectModelListener *listener)=0 |
Remove a listener from this object model. | |
virtual void | SetObjectBoundingBox (const csBox3 &bbox)=0 |
Override the bounding box of this mesh object in object space. | |
virtual void | SetPolygonMeshColldet (iPolygonMesh *polymesh)=0 |
Set a polygon mesh representing the geometry of the object. | |
virtual void | SetPolygonMeshShadows (iPolygonMesh *polymesh)=0 |
Set a polygon mesh representing the geometry of the object. | |
virtual void | SetPolygonMeshViscull (iPolygonMesh *polymesh)=0 |
Set a polygon mesh representing the geometry of the object. |
Detailed Description
This interface represents data related to some geometry in object space.It is a generic way to describe meshes in the engine. By using this interface you can make sure your code works on all engine geometry. The data returned by this class is in local object space.
Main creators of instances implementing this interface:
- All mesh objects implement this interface.
Main ways to get pointers to this interface:
Definition at line 65 of file objmodel.h.
Member Function Documentation
virtual void iObjectModel::AddListener | ( | iObjectModelListener * | listener | ) | [pure virtual] |
Add a listener to this object model.
This listener will be called whenever the object model changes or right before it is destroyed.
Implemented in csObjectModel.
virtual csPtr<iPolygonMesh> iObjectModel::CreateLowerDetailPolygonMesh | ( | float | detail | ) | [pure virtual] |
Create a polygon mesh representing a lower detail version of the object but without the restrictions of GetPolygonMeshViscull().
The floating point input number is 0 for minimum detail and 1 for highest detail. This function may return the same polygon mesh as GetPolygonMeshColldet() (but with ref count incremented by one). Can return 0 if this object model doesn't support that.
Implemented in csObjectModel.
virtual const csBox3& iObjectModel::GetObjectBoundingBox | ( | ) | [pure virtual] |
Get the bounding box in object space for this mesh object.
Implemented in csParticleSystem, and csMeshObject.
virtual void iObjectModel::GetObjectBoundingBox | ( | csBox3 & | bbox | ) | [pure virtual] |
Get the bounding box in object space for this mesh object.
- Deprecated:
- Use GetObjectBoundingBox() (without parameters) instead.
Implemented in csParticleSystem, and csMeshObject.
virtual iPolygonMesh* iObjectModel::GetPolygonMeshBase | ( | ) | [pure virtual] |
Get a polygon mesh representing the basic geometry of the object.
Can return 0 if this object model doesn't support that.
Implemented in csObjectModel.
virtual iPolygonMesh* iObjectModel::GetPolygonMeshColldet | ( | ) | [pure virtual] |
Get a polygon mesh representing the geometry of the object.
This mesh is useful for collision detection. Can return 0 if this object model doesn't support that.
Implemented in csObjectModel.
virtual iPolygonMesh* iObjectModel::GetPolygonMeshShadows | ( | ) | [pure virtual] |
Get a polygon mesh specifically for shadow casting (to be used by the shadow manager).
This polygon mesh is guaranteed to be smaller or equal to the real object. In other words: if you would render the original mesh in red and this one in blue you should not see any blue anywhere. Can return 0 if this object model doesn't support that. In that case the object will not be used for shadow casting.
Implemented in csObjectModel.
virtual iPolygonMesh* iObjectModel::GetPolygonMeshViscull | ( | ) | [pure virtual] |
Get a polygon mesh specifically for visibility culling (to be used as an occluder).
This polygon mesh is guaranteed to be smaller or equal to the real object. In other words: if you would render the original mesh in red and this one in blue you should not see any blue anywhere. This kind of lower detail version can be used for occlusion writing in a visibility culling system. Can return 0 if this object model doesn't support that. In that case the object will not be used for visibility culling.
Implemented in csObjectModel.
virtual void iObjectModel::GetRadius | ( | float & | radius, | |
csVector3 & | center | |||
) | [pure virtual] |
Get the radius and center of this object in object space.
Implemented in csParticleSystem, and csMeshObject.
virtual long iObjectModel::GetShapeNumber | ( | ) | const [pure virtual] |
Returns a number that will change whenever the shape of this object changes.
If that happens then the data in all the returned polygon meshes and bounding volumes will be invalid.
Implemented in csObjectModel.
virtual iTerraFormer* iObjectModel::GetTerraFormerColldet | ( | ) | [pure virtual] |
Get a terra former representing the geometry of the object.
This class is useful for collision detection. Can return 0 if this object model doesn't support that.
Implemented in csParticleSystem, csMeshObject, and csObjectModel.
virtual void iObjectModel::RemoveListener | ( | iObjectModelListener * | listener | ) | [pure virtual] |
virtual void iObjectModel::SetObjectBoundingBox | ( | const csBox3 & | bbox | ) | [pure virtual] |
Override the bounding box of this mesh object in object space.
Note that some mesh objects don't have a bounding box on their own and may delegate this call to their factory (like genmesh).
Implemented in csParticleSystem, and csMeshObject.
virtual void iObjectModel::SetPolygonMeshColldet | ( | iPolygonMesh * | polymesh | ) | [pure virtual] |
Set a polygon mesh representing the geometry of the object.
This mesh is useful for collision detection. This can be used to replace the default polygon mesh returned by GetPolygonMeshColldet() with one that has less detail or even to support polygon mesh for mesh objects that otherwise don't support it. The object model will keep a reference to the given polymesh.
Implemented in csObjectModel.
virtual void iObjectModel::SetPolygonMeshShadows | ( | iPolygonMesh * | polymesh | ) | [pure virtual] |
Set a polygon mesh representing the geometry of the object.
This mesh is useful for shadow casting. This can be used to replace the default polygon mesh returned by GetPolygonMeshShadows() with one that has less detail or even to support polygon mesh for mesh objects that otherwise don't support it. The object model will keep a reference to the given polymesh.
Implemented in csObjectModel.
virtual void iObjectModel::SetPolygonMeshViscull | ( | iPolygonMesh * | polymesh | ) | [pure virtual] |
Set a polygon mesh representing the geometry of the object.
This mesh is useful for visibility culling. This can be used to replace the default polygon mesh returned by GetPolygonMeshViscull() with one that has less detail or even to support polygon mesh for mesh objects that otherwise don't support it. The object model will keep a reference to the given polymesh.
Implemented in csObjectModel.
The documentation for this struct was generated from the following file:
- imesh/objmodel.h
Generated for Crystal Space by doxygen 1.4.7