csMeshObject Class Reference
This is an abstract implementation of iMeshObject. More...
#include <cstool/meshobjtmpl.h>
Inheritance diagram for csMeshObject:
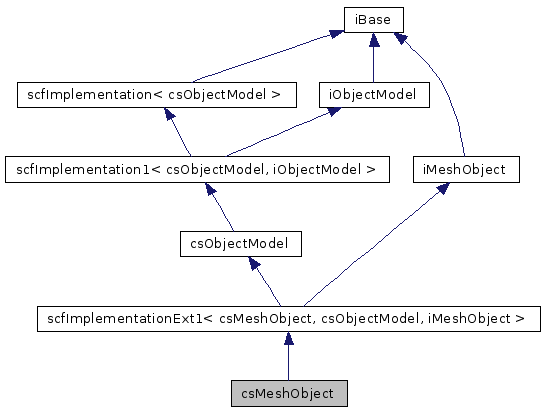
Public Member Functions | |
virtual csPtr< iMeshObject > | Clone () |
See imesh/object.h for specification. | |
csMeshObject (iEngine *engine) | |
Constructor. | |
virtual bool | GetColor (csColor &color) const |
See imesh/object.h for specification. | |
virtual iMeshObjectFactory * | GetFactory () const =0 |
See imesh/object.h for specification. | |
virtual csFlags & | GetFlags () |
See imesh/object.h for specification. | |
virtual iMaterialWrapper * | GetMaterialWrapper () const |
See imesh/object.h for specification. | |
virtual iMeshWrapper * | GetMeshWrapper () const |
See imesh/object.h for specification. | |
virtual uint | GetMixMode () const |
Get mix mode. | |
virtual const csBox3 & | GetObjectBoundingBox () |
See imesh/objmodel.h for specification. | |
virtual void | GetObjectBoundingBox (csBox3 &bbox) |
See imesh/objmodel.h for specification. | |
virtual iObjectModel * | GetObjectModel () |
See imesh/object.h for specification. | |
virtual void | GetRadius (float &radius, csVector3 ¢er) |
See imesh/objmodel.h for specification. | |
virtual csRenderMesh ** | GetRenderMeshes (int &num, iRenderView *, iMovable *, uint32) |
See imesh/object.h for specification. | |
virtual iTerraFormer * | GetTerraFormerColldet () |
See imesh/objmodel.h for specification. | |
virtual iMeshObjectDrawCallback * | GetVisibleCallback () const |
See imesh/object.h for specification. | |
virtual void | HardTransform (const csReversibleTransform &t) |
See imesh/object.h for specification. | |
virtual bool | HitBeamObject (const csVector3 &start, const csVector3 &end, csVector3 &isect, float *pr, int *polygon_idx=0, iMaterialWrapper **=0) |
See imesh/object.h for specification. | |
virtual bool | HitBeamOutline (const csVector3 &start, const csVector3 &end, csVector3 &isect, float *pr) |
See imesh/object.h for specification. | |
virtual void | InvalidateMaterialHandles () |
see imesh/object.h for specification. | |
virtual void | NextFrame (csTicks current_time, const csVector3 &pos, uint currentFrame) |
See imesh/object.h for specification. | |
virtual void | PositionChild (iMeshObject *, csTicks) |
see imesh/object.h for specification. | |
virtual bool | SetColor (const csColor &color) |
See imesh/object.h for specification. | |
virtual bool | SetMaterialWrapper (iMaterialWrapper *material) |
See imesh/object.h for specification. | |
virtual void | SetMeshWrapper (iMeshWrapper *logparent) |
See imesh/object.h for specification. | |
virtual void | SetMixMode (uint) |
Set mix mode. Default implementation doesn't do anything. | |
virtual void | SetObjectBoundingBox (const csBox3 &bbox) |
See imesh/objmodel.h for specification. | |
virtual void | SetVisibleCallback (iMeshObjectDrawCallback *cb) |
See imesh/object.h for specification. | |
virtual bool | SupportsHardTransform () const |
See imesh/object.h for specification. | |
virtual | ~csMeshObject () |
Destructor. | |
Protected Member Functions | |
void | WantToDie () |
Tell the engine that this object wants to be deleted. | |
Protected Attributes | |
csBox3 | boundingbox |
The bounding box. | |
iEngine * | Engine |
pointer to the engine if available. | |
csFlags | flags |
Flags. | |
iMeshWrapper * | LogParent |
logical parent (usually the wrapper object from the engine) | |
csRef< iMeshObjectDrawCallback > | VisCallback |
the drawing callback |
Detailed Description
This is an abstract implementation of iMeshObject.It can be used to write custom mesh object implementations more easily. Currently it supports the following common functions of mesh objects:
- Implementation of iMeshObject
- Implementation of iObjectModel
- Storing a "visible callback"
- Storing a logical parent
- Storing object model properties
- Default implementation of most methods
Definition at line 75 of file meshobjtmpl.h.
Constructor & Destructor Documentation
csMeshObject::csMeshObject | ( | iEngine * | engine | ) |
Constructor.
virtual csMeshObject::~csMeshObject | ( | ) | [virtual] |
Destructor.
Member Function Documentation
virtual csPtr<iMeshObject> csMeshObject::Clone | ( | ) | [inline, virtual] |
See imesh/object.h for specification.
The default implementation does nothing and returns 0.
Implements iMeshObject.
Definition at line 115 of file meshobjtmpl.h.
virtual bool csMeshObject::GetColor | ( | csColor & | color | ) | const [virtual] |
See imesh/object.h for specification.
The default implementation does not support a base color.
Implements iMeshObject.
virtual iMeshObjectFactory* csMeshObject::GetFactory | ( | ) | const [pure virtual] |
See imesh/object.h for specification.
There is no default implementation for this method.
Implements iMeshObject.
virtual csFlags& csMeshObject::GetFlags | ( | ) | [inline, virtual] |
See imesh/object.h for specification.
Implements iMeshObject.
Definition at line 120 of file meshobjtmpl.h.
virtual iMaterialWrapper* csMeshObject::GetMaterialWrapper | ( | ) | const [virtual] |
See imesh/object.h for specification.
The default implementation does not support a material.
Implements iMeshObject.
virtual iMeshWrapper* csMeshObject::GetMeshWrapper | ( | ) | const [virtual] |
See imesh/object.h for specification.
This function is handled completely in csMeshObject.
Implements iMeshObject.
virtual uint csMeshObject::GetMixMode | ( | ) | const [inline, virtual] |
Get mix mode.
Implements iMeshObject.
Definition at line 224 of file meshobjtmpl.h.
References CS_FX_COPY.
virtual const csBox3& csMeshObject::GetObjectBoundingBox | ( | ) | [virtual] |
See imesh/objmodel.h for specification.
The default implementation returns an infinite bounding box.
Implements iObjectModel.
virtual void csMeshObject::GetObjectBoundingBox | ( | csBox3 & | bbox | ) | [virtual] |
See imesh/objmodel.h for specification.
The default implementation returns an infinite bounding box.
Implements iObjectModel.
virtual iObjectModel* csMeshObject::GetObjectModel | ( | ) | [inline, virtual] |
See imesh/object.h for specification.
Implements iMeshObject.
Definition at line 195 of file meshobjtmpl.h.
virtual void csMeshObject::GetRadius | ( | float & | radius, | |
csVector3 & | center | |||
) | [virtual] |
See imesh/objmodel.h for specification.
The default implementation returns an infinite radius.
Implements iObjectModel.
virtual csRenderMesh** csMeshObject::GetRenderMeshes | ( | int & | num, | |
iRenderView * | , | |||
iMovable * | , | |||
uint32 | ||||
) | [inline, virtual] |
See imesh/object.h for specification.
The default implementation does nothing and always returns 0.
Implements iMeshObject.
Definition at line 126 of file meshobjtmpl.h.
virtual iTerraFormer* csMeshObject::GetTerraFormerColldet | ( | ) | [inline, virtual] |
See imesh/objmodel.h for specification.
The default implementation returns 0.
Reimplemented from csObjectModel.
Definition at line 266 of file meshobjtmpl.h.
virtual iMeshObjectDrawCallback* csMeshObject::GetVisibleCallback | ( | ) | const [virtual] |
See imesh/object.h for specification.
This function is handled completely in csMeshObject.
Implements iMeshObject.
virtual void csMeshObject::HardTransform | ( | const csReversibleTransform & | t | ) | [virtual] |
See imesh/object.h for specification.
The default implementation does nothing.
Implements iMeshObject.
virtual bool csMeshObject::HitBeamObject | ( | const csVector3 & | start, | |
const csVector3 & | end, | |||
csVector3 & | isect, | |||
float * | pr, | |||
int * | polygon_idx = 0 , |
|||
iMaterialWrapper ** | = 0 | |||
) | [virtual] |
See imesh/object.h for specification.
The default implementation will always return a miss.
Implements iMeshObject.
virtual bool csMeshObject::HitBeamOutline | ( | const csVector3 & | start, | |
const csVector3 & | end, | |||
csVector3 & | isect, | |||
float * | pr | |||
) | [virtual] |
See imesh/object.h for specification.
The default implementation will always return a miss.
Implements iMeshObject.
virtual void csMeshObject::InvalidateMaterialHandles | ( | ) | [inline, virtual] |
see imesh/object.h for specification.
The default implementation does nothing.
Implements iMeshObject.
Definition at line 230 of file meshobjtmpl.h.
virtual void csMeshObject::NextFrame | ( | csTicks | current_time, | |
const csVector3 & | pos, | |||
uint | currentFrame | |||
) | [virtual] |
See imesh/object.h for specification.
The default implementation does nothing.
Implements iMeshObject.
virtual void csMeshObject::PositionChild | ( | iMeshObject * | , | |
csTicks | ||||
) | [inline, virtual] |
see imesh/object.h for specification.
The default implementation does nothing.
Implements iMeshObject.
Definition at line 236 of file meshobjtmpl.h.
virtual bool csMeshObject::SetColor | ( | const csColor & | color | ) | [virtual] |
See imesh/object.h for specification.
The default implementation does not support a base color.
Implements iMeshObject.
virtual bool csMeshObject::SetMaterialWrapper | ( | iMaterialWrapper * | material | ) | [virtual] |
See imesh/object.h for specification.
The default implementation does not support a material.
Implements iMeshObject.
virtual void csMeshObject::SetMeshWrapper | ( | iMeshWrapper * | logparent | ) | [virtual] |
See imesh/object.h for specification.
This function is handled completely in csMeshObject.
Implements iMeshObject.
virtual void csMeshObject::SetMixMode | ( | uint | ) | [inline, virtual] |
Set mix mode. Default implementation doesn't do anything.
Implements iMeshObject.
Definition at line 222 of file meshobjtmpl.h.
virtual void csMeshObject::SetObjectBoundingBox | ( | const csBox3 & | bbox | ) | [virtual] |
See imesh/objmodel.h for specification.
Overrides the default bounding box.
Implements iObjectModel.
virtual void csMeshObject::SetVisibleCallback | ( | iMeshObjectDrawCallback * | cb | ) | [virtual] |
See imesh/object.h for specification.
This function is handled completely in csMeshObject. The actual implementation just has to use the VisCallback variable to perform the callback.
Implements iMeshObject.
virtual bool csMeshObject::SupportsHardTransform | ( | ) | const [virtual] |
See imesh/object.h for specification.
The default implementation returns false.
Implements iMeshObject.
void csMeshObject::WantToDie | ( | ) | [protected] |
Tell the engine that this object wants to be deleted.
Member Data Documentation
csBox3 csMeshObject::boundingbox [protected] |
iEngine* csMeshObject::Engine [protected] |
csFlags csMeshObject::flags [protected] |
iMeshWrapper* csMeshObject::LogParent [protected] |
logical parent (usually the wrapper object from the engine)
Definition at line 83 of file meshobjtmpl.h.
csRef<iMeshObjectDrawCallback> csMeshObject::VisCallback [protected] |
The documentation for this class was generated from the following file:
- cstool/meshobjtmpl.h
Generated for Crystal Space by doxygen 1.4.7