csParticleSystem Class Reference
[Common Plugin Classes]
This class represents a particle system.
More...
#include <csplugincommon/particlesys/partgen.h>
Inheritance diagram for csParticleSystem:
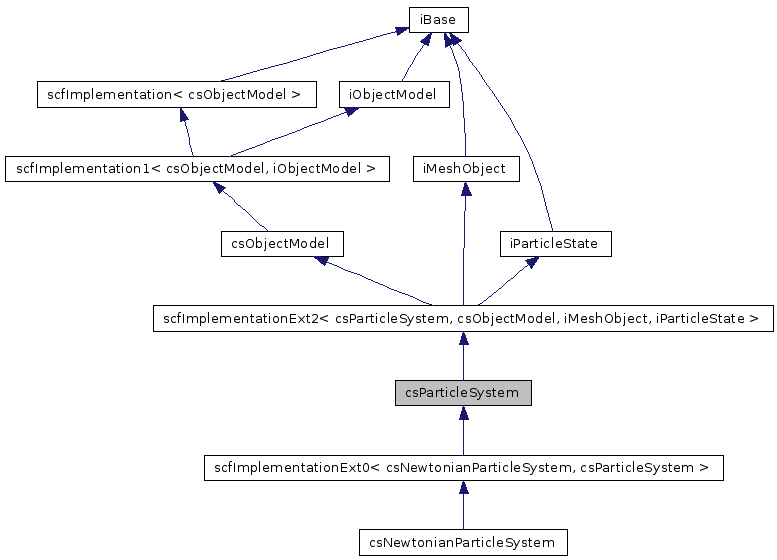
Public Member Functions | |
virtual void | AddColor (const csColor &col) |
Add particle colors, convenience function. | |
void | AppendParticle (iMeshObject *mesh, iParticle *part, iSprite2DState *spr2d) |
Add a new particle, increases num_particles. Do a DecRef yourself. | |
void | AppendRectSprite (float width, float height, iMaterialWrapper *mat, bool lighted) |
Add an rectangle shaped sprite2d particle. | |
void | AppendRegularSprite (int n, float radius, iMaterialWrapper *mat, bool lighted) |
Add a sprite2d n-gon with material, and given radius. | |
virtual csPtr< iMeshObject > | Clone () |
Creates a copy of this object and returns the clone. | |
csParticleSystem (iObjectRegistry *object_reg, iMeshObjectFactory *factory) | |
Make a new system. | |
float | GetAlpha () const |
Get the probable alpha of the particles. | |
const csBox3 & | GetBoundingBox () const |
Get the bounding box for this particle system. | |
bool | GetChangeAlpha (float &factor) const |
see if change alpha is enabled, and get the value if so. | |
bool | GetChangeColor (csColor &col) const |
see if change color is enabled, and get a copy if so. | |
bool | GetChangeRotation (float &angle) const |
see if change rotation is enabled, and get the angle if so. | |
bool | GetChangeSize (float &factor) const |
see if change size is enabled, and get the value if so. | |
virtual bool | GetColor (csColor &col) const |
Get the base color of the mesh. | |
virtual iMeshObjectFactory * | GetFactory () const |
Get the reference to the factory that created this mesh object. | |
virtual csFlags & | GetFlags () |
Get flags for this object. | |
virtual iMaterialWrapper * | GetMaterialWrapper () const |
Get the material of the mesh. | |
virtual iMeshWrapper * | GetMeshWrapper () const |
Get the logical parent for this mesh object. | |
virtual uint | GetMixMode () const |
Get mix mode. | |
size_t | GetNumParticles () const |
How many particles the system currently has. | |
virtual const csBox3 & | GetObjectBoundingBox () |
Get the bounding box in object space for this mesh object. | |
virtual void | GetObjectBoundingBox (csBox3 &bbox) |
Get the bounding box in object space for this mesh object. | |
virtual iObjectModel * | GetObjectModel () |
Get the generic interface describing the geometry of this mesh. | |
iParticle * | GetParticle (size_t idx) const |
Get a particle. | |
size_t | GetParticleCount () const |
Get the number of particles. | |
virtual void | GetRadius (float &rad, csVector3 ¢) |
Get the radius and center of this object in object space. | |
virtual csRenderMesh ** | GetRenderMeshes (int &n, iRenderView *rview, iMovable *movable, uint32 frustum_mask) |
Returns the set of render meshes. | |
bool | GetSelfDestruct () const |
returns whether the system will self destruct | |
virtual iTerraFormer * | GetTerraFormerColldet () |
Get a terra former representing the geometry of the object. | |
csTicks | GetTimeToLive () const |
if the system will self destruct, returns the time to live in msec. | |
virtual iMeshObjectDrawCallback * | GetVisibleCallback () const |
Get the current visible callback. | |
virtual int | HitBeamBBox (const csVector3 &, const csVector3 &, csVector3 &, float *) |
virtual bool | HitBeamObject (const csVector3 &start, const csVector3 &end, csVector3 &isect, float *pr, int *polygon_idx=0, iMaterialWrapper **material=0) |
Check if this mesh is hit by this object space vector. | |
virtual bool | HitBeamOutline (const csVector3 &, const csVector3 &, csVector3 &, float *) |
Check if this mesh is hit by this object space vector. | |
virtual void | InvalidateMaterialHandles () |
Material changed. | |
virtual void | NextFrame (csTicks current_time, const csVector3 &, uint) |
Control animation of this object. | |
virtual void | PositionChild (iMeshObject *, csTicks) |
see imesh/object.h for specification. | |
void | RemoveParticles () |
Remove all particles. | |
virtual void | Rotate (float angle) |
Rotate all particles. | |
virtual void | ScaleBy (float factor) |
Scale all particles. | |
void | SetAlpha (float alpha) |
Set the alpha of particles. | |
void | SetChangeAlpha (float factor) |
Change alpha of all particles, by factor per second. | |
void | SetChangeColor (const csColor &col) |
Change color of all particles, by col per second. | |
void | SetChangeRotation (float angle) |
Change rotation of all particles, by angle in radians per second. | |
void | SetChangeSize (float factor) |
Change size of all particles, by factor per second. | |
virtual bool | SetColor (const csColor &col) |
Set the base color of the mesh. | |
virtual bool | SetMaterialWrapper (iMaterialWrapper *mat) |
Set the material of the mesh. | |
virtual void | SetMeshWrapper (iMeshWrapper *lp) |
Set a reference to the mesh wrapper holding the mesh objects. | |
virtual void | SetMixMode (uint mode) |
Set mix mode. Note that not all meshes may support this. | |
virtual void | SetObjectBoundingBox (const csBox3 &bbox) |
Override the bounding box of this mesh object in object space. | |
virtual void | SetParticleCount (size_t num) |
Set the number of particles to use. | |
void | SetSelfDestruct (csTicks t) |
Set selfdestruct mode on, and msec to live. | |
virtual void | SetupColor () |
Set particle colors, convenience function. | |
virtual void | SetupMixMode () |
Set particle mixmodes, convenience function. | |
virtual void | SetVisibleCallback (iMeshObjectDrawCallback *cb) |
Register a callback to the mesh object which will be called from within Draw() if the mesh object thinks that the object is really visible. | |
void | UnsetChangeAlpha () |
Stop change of alpha. | |
void | UnsetChangeColor () |
Stop change of color. | |
void | UnsetChangeRotation () |
Stop change of rotation. | |
void | UnsetChangeSize () |
Stop change of size. | |
void | UnSetSelfDestruct () |
system will no longer self destruct | |
virtual void | Update (csTicks elapsed_time) |
Update the state of the particles as time has passed. | |
virtual | ~csParticleSystem () |
Destroy particle system, and all particles. | |
Protected Member Functions | |
void | ChangeObject () |
csVector3 | GetRandomDirection (csVector3 const &magnitude, csVector3 const &offset) |
Return vector with -1..+1 members. Varying length. | |
csVector3 | GetRandomDirection () |
Return vector with -1..+1 members. Varying length. | |
csVector3 | GetRandomPosition (csBox3 const &box) |
Return vector with random position within box. | |
void | SetupBuffers (size_t part_sides) |
Setup the buffers for the particles. | |
virtual void | SetupObject () |
Setup this object. | |
Protected Attributes | |
float | alpha_now |
float | alphapersecond |
float | anglepersecond |
csBox3 | bbox |
bounding box in 3d of all particles in this system. | |
bool | change_alpha |
Alpha change. | |
bool | change_color |
Color change. | |
bool | change_rotation |
Rotate particles, angle in radians. | |
bool | change_size |
Size change. | |
csColor | color |
Color of all particles. | |
csColor | colorpersecond |
uint32 | current_features |
float | current_lod |
iEngine * | engine |
iMeshObjectFactory * | factory |
csFlags | flags |
csRef< iGraphics3D > | g3d |
csRef< iRenderBuffer > | index_buffer |
bool | initialized |
csRef< iLightManager > | light_mgr |
iMeshWrapper * | logparent |
csRef< iMaterialWrapper > | mat |
Material for all particles. | |
uint | MixMode |
MixMode for all particles. | |
size_t | number |
iObjectRegistry * | object_reg |
csVector3 * | part_pos |
particle position | |
size_t | part_sides |
csRefArray< iParticle > | particles |
csRefArray< iMeshObject > | partmeshes |
iParticle ptrs to the particles. | |
csFrameDataHolder< PerFrameData > | perFrameHolder |
csTicks | prev_time |
Previous time. | |
float | radius |
Object space radius. | |
csRenderMeshHolder | rmHolder |
float | scalepersecond |
bool | self_destruct |
Self destruct and when. | |
csRef< iMeshObjectFactory > | spr_factory |
Pointer to a mesh object factory for 2D sprites. | |
csRefArray< iSprite2DState > | sprite2ds |
csTicks | time_to_live |
size_t | TriangleCount |
size_t | VertexCount |
iMeshObjectDrawCallback * | vis_cb |
Classes | |
struct | PerFrameData |
Detailed Description
This class represents a particle system.It is a set of iParticles. Subclasses of this class may be of more interest to users. More specialised particle systems can be found below.
Definition at line 63 of file partgen.h.
Constructor & Destructor Documentation
csParticleSystem::csParticleSystem | ( | iObjectRegistry * | object_reg, | |
iMeshObjectFactory * | factory | |||
) |
Make a new system.
Also adds the particle system to the list of the current engine.
virtual csParticleSystem::~csParticleSystem | ( | ) | [virtual] |
Destroy particle system, and all particles.
Member Function Documentation
virtual void csParticleSystem::AddColor | ( | const csColor & | col | ) | [virtual] |
Add particle colors, convenience function.
void csParticleSystem::AppendParticle | ( | iMeshObject * | mesh, | |
iParticle * | part, | |||
iSprite2DState * | spr2d | |||
) | [inline] |
void csParticleSystem::AppendRectSprite | ( | float | width, | |
float | height, | |||
iMaterialWrapper * | mat, | |||
bool | lighted | |||
) |
Add an rectangle shaped sprite2d particle.
Pass along half w and h. adds sprite to engine list.
void csParticleSystem::AppendRegularSprite | ( | int | n, | |
float | radius, | |||
iMaterialWrapper * | mat, | |||
bool | lighted | |||
) |
Add a sprite2d n-gon with material, and given radius.
adds sprite to engine list.
virtual csPtr<iMeshObject> csParticleSystem::Clone | ( | ) | [inline, virtual] |
float csParticleSystem::GetAlpha | ( | ) | const [inline, virtual] |
const csBox3& csParticleSystem::GetBoundingBox | ( | ) | const [inline] |
bool csParticleSystem::GetChangeAlpha | ( | float & | factor | ) | const [inline, virtual] |
bool csParticleSystem::GetChangeColor | ( | csColor & | col | ) | const [inline, virtual] |
bool csParticleSystem::GetChangeRotation | ( | float & | angle | ) | const [inline, virtual] |
bool csParticleSystem::GetChangeSize | ( | float & | factor | ) | const [inline, virtual] |
virtual bool csParticleSystem::GetColor | ( | csColor & | col | ) | const [inline, virtual] |
virtual iMeshObjectFactory* csParticleSystem::GetFactory | ( | ) | const [inline, virtual] |
virtual csFlags& csParticleSystem::GetFlags | ( | ) | [inline, virtual] |
Get flags for this object.
The following flags are at least supported:
- CS_MESH_STATICPOS: mesh will never move.
- CS_MESH_STATICSHAPE: mesh will never animate.
Implements iMeshObject.
virtual iMaterialWrapper* csParticleSystem::GetMaterialWrapper | ( | ) | const [inline, virtual] |
virtual iMeshWrapper* csParticleSystem::GetMeshWrapper | ( | ) | const [inline, virtual] |
Get the logical parent for this mesh object.
See SetMeshWrapper() for more information.
Implements iMeshObject.
virtual uint csParticleSystem::GetMixMode | ( | ) | const [inline, virtual] |
size_t csParticleSystem::GetNumParticles | ( | ) | const [inline] |
virtual const csBox3& csParticleSystem::GetObjectBoundingBox | ( | ) | [inline, virtual] |
virtual void csParticleSystem::GetObjectBoundingBox | ( | csBox3 & | bbox | ) | [inline, virtual] |
Get the bounding box in object space for this mesh object.
- Deprecated:
- Use GetObjectBoundingBox() (without parameters) instead.
Implements iObjectModel.
Definition at line 288 of file partgen.h.
References bbox.
virtual iObjectModel* csParticleSystem::GetObjectModel | ( | ) | [inline, virtual] |
Get the generic interface describing the geometry of this mesh.
If the factory supports this you should preferably use the object model from the factory instead.
Implements iMeshObject.
iParticle* csParticleSystem::GetParticle | ( | size_t | idx | ) | const [inline] |
size_t csParticleSystem::GetParticleCount | ( | ) | const [inline] |
virtual void csParticleSystem::GetRadius | ( | float & | rad, | |
csVector3 & | cent | |||
) | [inline, virtual] |
csVector3 csParticleSystem::GetRandomDirection | ( | csVector3 const & | magnitude, | |
csVector3 const & | offset | |||
) | [protected] |
Return vector with -1..+1 members. Varying length.
csVector3 csParticleSystem::GetRandomDirection | ( | ) | [protected] |
Return vector with -1..+1 members. Varying length.
Return vector with random position within box.
virtual csRenderMesh** csParticleSystem::GetRenderMeshes | ( | int & | n, | |
iRenderView * | rview, | |||
iMovable * | movable, | |||
uint32 | frustum_mask | |||
) | [virtual] |
Returns the set of render meshes.
The frustum_mask is given by the culler and contains a mask with all relevant planes for the given object. These planes correspond with the clip planes kept by iRenderView.
Implements iMeshObject.
bool csParticleSystem::GetSelfDestruct | ( | ) | const [inline] |
virtual iTerraFormer* csParticleSystem::GetTerraFormerColldet | ( | ) | [inline, virtual] |
Get a terra former representing the geometry of the object.
This class is useful for collision detection. Can return 0 if this object model doesn't support that.
Reimplemented from csObjectModel.
csTicks csParticleSystem::GetTimeToLive | ( | ) | const [inline] |
virtual iMeshObjectDrawCallback* csParticleSystem::GetVisibleCallback | ( | ) | const [inline, virtual] |
virtual bool csParticleSystem::HitBeamObject | ( | const csVector3 & | start, | |
const csVector3 & | end, | |||
csVector3 & | isect, | |||
float * | pr, | |||
int * | polygon_idx = 0 , |
|||
iMaterialWrapper ** | material = 0 | |||
) | [inline, virtual] |
Check if this mesh is hit by this object space vector.
Return the collision point in object space coordinates. This is the most detailed version (and also the slowest). The returned hit will be guaranteed to be the point closest to the 'start' of the beam. If the object supports this then an index of the hit polygon will be returned (or -1 if not supported or no hit).
- Parameters:
-
start Start of the beam to trace. end End of the beam to trace. isect Returns the point of the hit. pr Returns the position of the hit as a value between 0 and 1, where 0 means start and 1 means end. polygon_idx Index of the polygon hit on the mesh (or -1 if not supported). material If not 0 then the hit material will be put here. Or 0 in case this mesh object doesn't support material selection.
Implements iMeshObject.
virtual bool csParticleSystem::HitBeamOutline | ( | const csVector3 & | , | |
const csVector3 & | , | |||
csVector3 & | , | |||
float * | ||||
) | [inline, virtual] |
Check if this mesh is hit by this object space vector.
This will do a test based on the outline of the object. This means that it is more accurate than HitBeamBBox(). Note that this routine will typically be faster than HitBeamObject(). The hit may be on the front or the back of the object, but will indicate that it iterrupts the beam.
Implements iMeshObject.
virtual void csParticleSystem::InvalidateMaterialHandles | ( | ) | [inline, virtual] |
Material changed.
This is an 'event' that the engine (or another party managing materials) will send out as soon as the material handles are changed in some way which requires the mesh object to fetch it again (i.e. to call materialwrapper->GetMaterialHandle()) again.
Implements iMeshObject.
virtual void csParticleSystem::PositionChild | ( | iMeshObject * | , | |
csTicks | ||||
) | [inline, virtual] |
see imesh/object.h for specification.
The default implementation does nothing.
Implements iMeshObject.
void csParticleSystem::RemoveParticles | ( | ) |
Remove all particles.
virtual void csParticleSystem::Rotate | ( | float | angle | ) | [virtual] |
Rotate all particles.
virtual void csParticleSystem::ScaleBy | ( | float | factor | ) | [virtual] |
Scale all particles.
void csParticleSystem::SetAlpha | ( | float | alpha | ) | [inline, virtual] |
Set the alpha of particles.
Implements iParticleState.
Definition at line 244 of file partgen.h.
References CS_FX_SETALPHA.
void csParticleSystem::SetChangeAlpha | ( | float | factor | ) | [inline, virtual] |
void csParticleSystem::SetChangeColor | ( | const csColor & | col | ) | [inline, virtual] |
void csParticleSystem::SetChangeRotation | ( | float | angle | ) | [inline, virtual] |
void csParticleSystem::SetChangeSize | ( | float | factor | ) | [inline, virtual] |
virtual bool csParticleSystem::SetColor | ( | const csColor & | col | ) | [inline, virtual] |
Set the base color of the mesh.
This color will be added to whatever color is set for lighting. Not all meshes need to support this. This function will return true if it worked.
Implements iMeshObject.
virtual bool csParticleSystem::SetMaterialWrapper | ( | iMaterialWrapper * | mat | ) | [inline, virtual] |
Set the material of the mesh.
This only works for single-material meshes. If not supported this function will return false.
Implements iMeshObject.
Definition at line 367 of file partgen.h.
References mat.
virtual void csParticleSystem::SetMeshWrapper | ( | iMeshWrapper * | lp | ) | [inline, virtual] |
Set a reference to the mesh wrapper holding the mesh objects.
Note that this function should NOT increase the ref-count of the given logical parent because this would cause a circular reference (since the logical parent already holds a reference to this mesh object).
Implements iMeshObject.
virtual void csParticleSystem::SetMixMode | ( | uint | mode | ) | [inline, virtual] |
virtual void csParticleSystem::SetObjectBoundingBox | ( | const csBox3 & | bbox | ) | [inline, virtual] |
Override the bounding box of this mesh object in object space.
Note that some mesh objects don't have a bounding box on their own and may delegate this call to their factory (like genmesh).
Implements iObjectModel.
Definition at line 298 of file partgen.h.
References bbox.
virtual void csParticleSystem::SetParticleCount | ( | size_t | num | ) | [inline, virtual] |
void csParticleSystem::SetSelfDestruct | ( | csTicks | t | ) | [inline, virtual] |
void csParticleSystem::SetupBuffers | ( | size_t | part_sides | ) | [protected] |
Setup the buffers for the particles.
virtual void csParticleSystem::SetupColor | ( | ) | [virtual] |
Set particle colors, convenience function.
virtual void csParticleSystem::SetupMixMode | ( | ) | [virtual] |
Set particle mixmodes, convenience function.
virtual void csParticleSystem::SetupObject | ( | ) | [protected, virtual] |
Setup this object.
virtual void csParticleSystem::SetVisibleCallback | ( | iMeshObjectDrawCallback * | cb | ) | [inline, virtual] |
Register a callback to the mesh object which will be called from within Draw() if the mesh object thinks that the object is really visible.
Depending on the type of mesh object this can be very accurate or not accurate at all. But in all cases it will certainly be called if the object is visible.
Implements iMeshObject.
Definition at line 318 of file partgen.h.
References iBase::IncRef().
void csParticleSystem::UnsetChangeAlpha | ( | ) | [inline, virtual] |
void csParticleSystem::UnsetChangeColor | ( | ) | [inline, virtual] |
void csParticleSystem::UnsetChangeRotation | ( | ) | [inline, virtual] |
void csParticleSystem::UnsetChangeSize | ( | ) | [inline, virtual] |
void csParticleSystem::UnSetSelfDestruct | ( | ) | [inline, virtual] |
virtual void csParticleSystem::Update | ( | csTicks | elapsed_time | ) | [virtual] |
Update the state of the particles as time has passed.
i.e. move the particles, retexture, recolor ... this member function will set to_delete if self_destruct is enabled and time is up.
Reimplemented in csNewtonianParticleSystem.
Member Data Documentation
csBox3 csParticleSystem::bbox [protected] |
bounding box in 3d of all particles in this system.
the particle system subclass has to give this a reasonable value. no particle may exceed the bbox.
Definition at line 101 of file partgen.h.
Referenced by GetObjectBoundingBox(), and SetObjectBoundingBox().
bool csParticleSystem::change_alpha [protected] |
bool csParticleSystem::change_color [protected] |
bool csParticleSystem::change_rotation [protected] |
bool csParticleSystem::change_size [protected] |
csColor csParticleSystem::color [protected] |
csRef<iMaterialWrapper> csParticleSystem::mat [protected] |
Material for all particles.
Definition at line 85 of file partgen.h.
Referenced by SetMaterialWrapper().
uint csParticleSystem::MixMode [protected] |
csVector3* csParticleSystem::part_pos [protected] |
csRefArray<iMeshObject> csParticleSystem::partmeshes [protected] |
csTicks csParticleSystem::prev_time [protected] |
float csParticleSystem::radius [protected] |
bool csParticleSystem::self_destruct [protected] |
csRef<iMeshObjectFactory> csParticleSystem::spr_factory [protected] |
The documentation for this class was generated from the following file:
- csplugincommon/particlesys/partgen.h
Generated for Crystal Space by doxygen 1.4.7