iDynamicSystem Struct Reference
This is the interface for the dynamics core. More...
#include <ivaria/dynamics.h>
Inheritance diagram for iDynamicSystem:
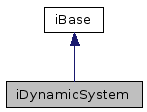
Public Member Functions | |
virtual void | AttachCollider (iDynamicsSystemCollider *collider)=0 |
Attach collider to dynamic system. | |
virtual bool | AttachColliderBox (const csVector3 &size, const csOrthoTransform &trans, float friction, float elasticity, float softness=0.01f)=0 |
Attaches a static collider box to world. | |
virtual bool | AttachColliderCylinder (float length, float radius, const csOrthoTransform &trans, float friction, float elasticity, float softness=0.01f)=0 |
Attaches a static collider cylinder to world (oriented along it's Z axis). | |
virtual bool | AttachColliderMesh (iMeshWrapper *mesh, const csOrthoTransform &trans, float friction, float elasticity, float softness=0.01f)=0 |
Attaches a static collider mesh to world. | |
virtual bool | AttachColliderPlane (const csPlane3 &plane, float friction, float elasticity, float softness=0.01f)=0 |
Attaches a static collider plane to world. | |
virtual bool | AttachColliderSphere (float radius, const csVector3 &offset, float friction, float elasticity, float softness=0.01f)=0 |
Attaches a static collider sphere to world. | |
virtual csPtr< iRigidBody > | CreateBody ()=0 |
Create a rigid body and add it to the simulation. | |
virtual csRef< iDynamicsSystemCollider > | CreateCollider ()=0 |
Create static collider and put it into simulation. | |
virtual csPtr< iBodyGroup > | CreateGroup ()=0 |
Create a body group. Bodies in a group don't collide with each other. | |
virtual csPtr< iJoint > | CreateJoint ()=0 |
Create a joint and add it to the simulation. | |
virtual void | DestroyCollider (iDynamicsSystemCollider *collider)=0 |
Destroy static collider. | |
virtual void | DestroyColliders ()=0 |
Destroy all static colliders. | |
virtual void | EnableAutoDisable (bool enable)=0 |
Turn on/off AutoDisable functionality. | |
virtual iRigidBody * | FindBody (const char *name)=0 |
Finds a body within a system. | |
virtual iRigidBody * | GetBody (unsigned int index)=0 |
Get Rigid Body by its index. | |
virtual int | GetBodysCount ()=0 |
Get rigid bodys count. | |
virtual csRef< iDynamicsSystemCollider > | GetCollider (unsigned int index)=0 |
Get static collider. | |
virtual int | GetColliderCount ()=0 |
Get static colliders count. | |
virtual iDynamicsMoveCallback * | GetDefaultMoveCallback ()=0 |
Get the default move callback. | |
virtual const csVector3 | GetGravity () const =0 |
Get the global gravity. | |
virtual float | GetLinearDampener () const =0 |
Get the global linear dampener setting. | |
virtual float | GetRollingDampener () const =0 |
Get the global rolling dampener setting. | |
virtual iObject * | QueryObject (void)=0 |
returns the underlying object | |
virtual void | RemoveBody (iRigidBody *body)=0 |
Create a rigid body and add it to the simulation. | |
virtual void | RemoveGroup (iBodyGroup *group)=0 |
Remove a group from a simulation. Those bodies now collide. | |
virtual void | RemoveJoint (iJoint *joint)=0 |
Remove a joint from the simulation. | |
virtual void | SetAutoDisableParams (float linear, float angular, int steps, float time)=0 |
Set the parameters for AutoDisable. | |
virtual void | SetGravity (const csVector3 &v)=0 |
Set the global gravity. | |
virtual void | SetLinearDampener (float d)=0 |
Set the global linear dampener. | |
virtual void | SetRollingDampener (float d)=0 |
Set the global rolling dampener. | |
virtual void | Step (float stepsize)=0 |
Step the simulation forward by stepsize. |
Detailed Description
This is the interface for the dynamics core.It handles all bookkeeping for rigid bodies and joints. It also handles collision response. Collision detection is done in another plugin.
Main creators of instances implementing this interface:
- iDynamicSystem::CreateSystem()
Main ways to get pointers to this interface:
- iDynamicSystem::FindSystem()
Definition at line 94 of file dynamics.h.
Member Function Documentation
virtual void iDynamicSystem::AttachCollider | ( | iDynamicsSystemCollider * | collider | ) | [pure virtual] |
Attach collider to dynamic system.
virtual bool iDynamicSystem::AttachColliderBox | ( | const csVector3 & | size, | |
const csOrthoTransform & | trans, | |||
float | friction, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Attaches a static collider box to world.
- Parameters:
-
size the box size along each axis trans a hard transform to apply to the mesh friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual bool iDynamicSystem::AttachColliderCylinder | ( | float | length, | |
float | radius, | |||
const csOrthoTransform & | trans, | |||
float | friction, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Attaches a static collider cylinder to world (oriented along it's Z axis).
- Parameters:
-
length the cylinder length along the axis radius the cylinder radius trans a hard transform to apply to the mesh friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual bool iDynamicSystem::AttachColliderMesh | ( | iMeshWrapper * | mesh, | |
const csOrthoTransform & | trans, | |||
float | friction, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Attaches a static collider mesh to world.
- Parameters:
-
mesh the mesh to use for collision detection trans a hard transform to apply to the mesh friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual bool iDynamicSystem::AttachColliderPlane | ( | const csPlane3 & | plane, | |
float | friction, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Attaches a static collider plane to world.
- Parameters:
-
plane describes the plane to added friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual bool iDynamicSystem::AttachColliderSphere | ( | float | radius, | |
const csVector3 & | offset, | |||
float | friction, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Attaches a static collider sphere to world.
- Parameters:
-
radius the radius of the sphere offset a translation of the sphere's center from the default (0,0,0) friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual csPtr<iRigidBody> iDynamicSystem::CreateBody | ( | ) | [pure virtual] |
Create a rigid body and add it to the simulation.
virtual csRef<iDynamicsSystemCollider> iDynamicSystem::CreateCollider | ( | ) | [pure virtual] |
Create static collider and put it into simulation.
After collision it will remain in the same place, but it will affect collided dynamic colliders (to make it dynamic, just attach it to the rigid body).
virtual csPtr<iBodyGroup> iDynamicSystem::CreateGroup | ( | ) | [pure virtual] |
Create a body group. Bodies in a group don't collide with each other.
Create a joint and add it to the simulation.
virtual void iDynamicSystem::DestroyCollider | ( | iDynamicsSystemCollider * | collider | ) | [pure virtual] |
Destroy static collider.
virtual void iDynamicSystem::DestroyColliders | ( | ) | [pure virtual] |
Destroy all static colliders.
virtual void iDynamicSystem::EnableAutoDisable | ( | bool | enable | ) | [pure virtual] |
Turn on/off AutoDisable functionality.
AutoDisable will stop moving objects if they are stable in order to save processing time. By default this is enabled.
virtual iRigidBody* iDynamicSystem::FindBody | ( | const char * | name | ) | [pure virtual] |
Finds a body within a system.
virtual iRigidBody* iDynamicSystem::GetBody | ( | unsigned int | index | ) | [pure virtual] |
Get Rigid Body by its index.
virtual int iDynamicSystem::GetBodysCount | ( | ) | [pure virtual] |
Get rigid bodys count.
virtual csRef<iDynamicsSystemCollider> iDynamicSystem::GetCollider | ( | unsigned int | index | ) | [pure virtual] |
Get static collider.
virtual int iDynamicSystem::GetColliderCount | ( | ) | [pure virtual] |
Get static colliders count.
virtual iDynamicsMoveCallback* iDynamicSystem::GetDefaultMoveCallback | ( | ) | [pure virtual] |
Get the default move callback.
virtual const csVector3 iDynamicSystem::GetGravity | ( | ) | const [pure virtual] |
Get the global gravity.
virtual float iDynamicSystem::GetLinearDampener | ( | ) | const [pure virtual] |
Get the global linear dampener setting.
virtual float iDynamicSystem::GetRollingDampener | ( | ) | const [pure virtual] |
Get the global rolling dampener setting.
virtual iObject* iDynamicSystem::QueryObject | ( | void | ) | [pure virtual] |
returns the underlying object
virtual void iDynamicSystem::RemoveBody | ( | iRigidBody * | body | ) | [pure virtual] |
Create a rigid body and add it to the simulation.
virtual void iDynamicSystem::RemoveGroup | ( | iBodyGroup * | group | ) | [pure virtual] |
Remove a group from a simulation. Those bodies now collide.
virtual void iDynamicSystem::RemoveJoint | ( | iJoint * | joint | ) | [pure virtual] |
Remove a joint from the simulation.
virtual void iDynamicSystem::SetAutoDisableParams | ( | float | linear, | |
float | angular, | |||
int | steps, | |||
float | time | |||
) | [pure virtual] |
Set the parameters for AutoDisable.
- Parameters:
-
linear Maximum linear movement to disable a body angular Maximum angular movement to disable a body steps Minimum number of steps the body meets linear and angular requirements before it is disabled. time Minimum time the body needs to meet linear and angular movement requirements before it is disabled.
virtual void iDynamicSystem::SetGravity | ( | const csVector3 & | v | ) | [pure virtual] |
Set the global gravity.
virtual void iDynamicSystem::SetLinearDampener | ( | float | d | ) | [pure virtual] |
Set the global linear dampener.
virtual void iDynamicSystem::SetRollingDampener | ( | float | d | ) | [pure virtual] |
Set the global rolling dampener.
virtual void iDynamicSystem::Step | ( | float | stepsize | ) | [pure virtual] |
Step the simulation forward by stepsize.
The documentation for this struct was generated from the following file:
- ivaria/dynamics.h
Generated for Crystal Space by doxygen 1.4.7