iDynamicsSystemCollider Struct Reference
This is the interface for a dynamics system collider. More...
#include <ivaria/dynamics.h>
Inheritance diagram for iDynamicsSystemCollider:
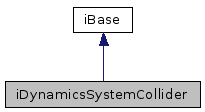
Public Member Functions | |
virtual bool | CreateBoxGeometry (const csVector3 &box_size)=0 |
Create Collider Geometry with given box (given by its size). | |
virtual bool | CreateCCylinderGeometry (float length, float radius)=0 |
Create Collider Geometry with Cylinder (given by its length and radius). | |
virtual bool | CreateMeshGeometry (iMeshWrapper *mesh)=0 |
Create Collider Geometry with given mesh geometry. | |
virtual bool | CreatePlaneGeometry (const csPlane3 &plane)=0 |
Create Collider Geometry with given plane. | |
virtual bool | CreateSphereGeometry (const csSphere &sphere)=0 |
Create Collider Geometry with given sphere. | |
virtual void | FillWithColliderGeometry (csRef< iGeneralFactoryState > genmesh_fact)=0 |
Fills given General Mesh factory with collider geometry. | |
virtual bool | GetBoxGeometry (csVector3 &size)=0 |
If collider has box geometry method will return true and size of box, otherwise it will return false. | |
virtual bool | GetCylinderGeometry (float &length, float &radius)=0 |
If collider has plane geometry method will return true and cylinder's length and radius, otherwise it will return false. | |
virtual float | GetDensity ()=0 |
Get bodys density. | |
virtual float | GetElasticity ()=0 |
Get collider surface elasticity. | |
virtual float | GetFriction ()=0 |
Get collider surface friction. | |
virtual csColliderGeometryType | GetGeometryType ()=0 |
Get geometry type. | |
virtual csOrthoTransform | GetLocalTransform ()=0 |
Get collider transform (when collider is attached to body transform will be in body space). | |
virtual bool | GetPlaneGeometry (csPlane3 &plane)=0 |
If collider has plane geometry method will return true and plane, otherwise it will return false. | |
virtual float | GetSoftness ()=0 |
Get collider surface friction. | |
virtual bool | GetSphereGeometry (csSphere &sphere)=0 |
If collider has sphere geometry method will return true and sphere, otherwise it will return false. | |
virtual csOrthoTransform | GetTransform ()=0 |
Get collider transform (it will be alwas in world cooridnates). | |
virtual bool | IsStatic ()=0 |
Check if collider is static. | |
virtual void | MakeDynamic ()=0 |
Make collider dynamic. | |
virtual void | MakeStatic ()=0 |
Make collider static. | |
virtual void | SetDensity (float density)=0 |
Set body density. | |
virtual void | SetElasticity (float elasticity)=0 |
Set softness of collider elasticity. | |
virtual void | SetFriction (float friction)=0 |
Set friction of collider surface. | |
virtual void | SetSoftness (float softness)=0 |
Set softness of collider surface. | |
virtual void | SetTransform (const csOrthoTransform &trans)=0 |
Set Collider transform. |
Detailed Description
This is the interface for a dynamics system collider.It keeps all properties that system uses for collision detection and after collision behaviour (like surface properties, collider geometry). It can be placed into dynamic system (then this will be "static" collider by default) or attached to body (then it will be "dynamic").
Main creators of instances implementing this interface:
Main ways to get pointers to this interface:
Main users of this interface:
Definition at line 680 of file dynamics.h.
Member Function Documentation
virtual bool iDynamicsSystemCollider::CreateBoxGeometry | ( | const csVector3 & | box_size | ) | [pure virtual] |
Create Collider Geometry with given box (given by its size).
virtual bool iDynamicsSystemCollider::CreateCCylinderGeometry | ( | float | length, | |
float | radius | |||
) | [pure virtual] |
Create Collider Geometry with Cylinder (given by its length and radius).
virtual bool iDynamicsSystemCollider::CreateMeshGeometry | ( | iMeshWrapper * | mesh | ) | [pure virtual] |
Create Collider Geometry with given mesh geometry.
virtual bool iDynamicsSystemCollider::CreatePlaneGeometry | ( | const csPlane3 & | plane | ) | [pure virtual] |
Create Collider Geometry with given plane.
virtual bool iDynamicsSystemCollider::CreateSphereGeometry | ( | const csSphere & | sphere | ) | [pure virtual] |
Create Collider Geometry with given sphere.
virtual void iDynamicsSystemCollider::FillWithColliderGeometry | ( | csRef< iGeneralFactoryState > | genmesh_fact | ) | [pure virtual] |
Fills given General Mesh factory with collider geometry.
virtual bool iDynamicsSystemCollider::GetBoxGeometry | ( | csVector3 & | size | ) | [pure virtual] |
If collider has box geometry method will return true and size of box, otherwise it will return false.
virtual bool iDynamicsSystemCollider::GetCylinderGeometry | ( | float & | length, | |
float & | radius | |||
) | [pure virtual] |
If collider has plane geometry method will return true and cylinder's length and radius, otherwise it will return false.
virtual float iDynamicsSystemCollider::GetDensity | ( | ) | [pure virtual] |
Get bodys density.
virtual float iDynamicsSystemCollider::GetElasticity | ( | ) | [pure virtual] |
Get collider surface elasticity.
virtual float iDynamicsSystemCollider::GetFriction | ( | ) | [pure virtual] |
Get collider surface friction.
virtual csColliderGeometryType iDynamicsSystemCollider::GetGeometryType | ( | ) | [pure virtual] |
Get geometry type.
virtual csOrthoTransform iDynamicsSystemCollider::GetLocalTransform | ( | ) | [pure virtual] |
Get collider transform (when collider is attached to body transform will be in body space).
virtual bool iDynamicsSystemCollider::GetPlaneGeometry | ( | csPlane3 & | plane | ) | [pure virtual] |
If collider has plane geometry method will return true and plane, otherwise it will return false.
virtual float iDynamicsSystemCollider::GetSoftness | ( | ) | [pure virtual] |
Get collider surface friction.
virtual bool iDynamicsSystemCollider::GetSphereGeometry | ( | csSphere & | sphere | ) | [pure virtual] |
If collider has sphere geometry method will return true and sphere, otherwise it will return false.
virtual csOrthoTransform iDynamicsSystemCollider::GetTransform | ( | ) | [pure virtual] |
Get collider transform (it will be alwas in world cooridnates).
virtual bool iDynamicsSystemCollider::IsStatic | ( | ) | [pure virtual] |
Check if collider is static.
virtual void iDynamicsSystemCollider::MakeDynamic | ( | ) | [pure virtual] |
Make collider dynamic.
Dynamic colliders acts (it collision is checked, and collision callbacks called) on every other collider and body.
virtual void iDynamicsSystemCollider::MakeStatic | ( | ) | [pure virtual] |
Make collider static.
Static collider acts on dynamic colliders and bodies, but ignores other static colliders (it won't do precise collision detection in that case).
virtual void iDynamicsSystemCollider::SetDensity | ( | float | density | ) | [pure virtual] |
Set body density.
This could look strange that collider needs to know this parameter, but it is used for reseting mass of conected body (it should depend on collider geometry)
virtual void iDynamicsSystemCollider::SetElasticity | ( | float | elasticity | ) | [pure virtual] |
Set softness of collider elasticity.
virtual void iDynamicsSystemCollider::SetFriction | ( | float | friction | ) | [pure virtual] |
Set friction of collider surface.
virtual void iDynamicsSystemCollider::SetSoftness | ( | float | softness | ) | [pure virtual] |
Set softness of collider surface.
virtual void iDynamicsSystemCollider::SetTransform | ( | const csOrthoTransform & | trans | ) | [pure virtual] |
Set Collider transform.
If this is "static" collider then given transform will be in world space, otherwise it will be in attached rigid body space.
The documentation for this struct was generated from the following file:
- ivaria/dynamics.h
Generated for Crystal Space by doxygen 1.4.7