iGraphics3D Struct Reference
This is the standard 3D graphics interface. More...
#include <ivideo/graph3d.h>
Inheritance diagram for iGraphics3D:
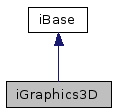
Public Member Functions | |
virtual bool | ActivateBuffers (csVertexAttrib *attribs, iRenderBuffer **buffers, unsigned int count)=0 |
Activate all given buffers. | |
virtual bool | ActivateBuffers (csRenderBufferHolder *holder, csRenderBufferName mapping[CS_VATTRIB_SPECIFIC_LAST+1])=0 |
Activate the buffers in the default buffer holder. | |
virtual bool | BeginDraw (int DrawFlags)=0 |
Start a new frame (see CSDRAW_XXX bit flags). | |
virtual void | Close ()=0 |
Close the 3D graphics display. | |
virtual void | ClosePortal ()=0 |
Close a portal previously opened with OpenPortal(). | |
virtual iHalo * | CreateHalo (float iR, float iG, float iB, unsigned char *iAlpha, int iWidth, int iHeight)=0 |
Create a halo of the specified color and return a handle. | |
virtual void | DeactivateBuffers (csVertexAttrib *attribs, unsigned int count)=0 |
Deactivate all given buffers. | |
virtual void | DisableZOffset ()=0 |
Disables offsetting of Z values. | |
virtual void | DrawLine (const csVector3 &v1, const csVector3 &v2, float fov, int color)=0 |
Draw a line in camera space. | |
virtual void | DrawMesh (const csCoreRenderMesh *mymesh, const csRenderMeshModes &modes, const iShaderVarStack *stacks)=0 |
Drawroutine. Only way to draw stuff. | |
virtual void | DrawPixmap (iTextureHandle *hTex, int sx, int sy, int sw, int sh, int tx, int ty, int tw, int th, uint8 Alpha=0)=0 |
Draw a pixmap using a rectangle from given texture. | |
virtual void | DrawSimpleMesh (const csSimpleRenderMesh &mesh, uint flags=0)=0 |
Draw a csSimpleRenderMesh on the screen. | |
virtual void | EnableZOffset ()=0 |
Enables offsetting of Z values. | |
virtual void | FinishDraw ()=0 |
End the frame and do a page swap. | |
virtual const csGraphics3DCaps * | GetCaps () const =0 |
Get the current driver's capabilities. | |
virtual iClipper2D * | GetClipper ()=0 |
Get clipper that was used. | |
virtual int | GetClipType () const =0 |
Return type of clipper. | |
virtual iGraphics2D * | GetDriver2D ()=0 |
Retrieve the associated canvas. | |
virtual int | GetHeight () const =0 |
Get drawing buffer height. | |
virtual const csPlane3 & | GetNearPlane () const =0 |
Get near clip plane. | |
virtual float | GetPerspectiveAspect () const =0 |
Get aspect ratio. | |
virtual void | GetPerspectiveCenter (int &x, int &y) const =0 |
Get perspective center. | |
virtual long | GetRenderState (G3D_RENDERSTATEOPTION op) const =0 |
Get a renderstate value. | |
virtual iTextureHandle * | GetRenderTarget () const =0 |
Get the current render target (0 for screen). | |
virtual iTextureManager * | GetTextureManager ()=0 |
Retrieve the texture manager. | |
virtual int | GetWidth () const =0 |
Get drawing buffer width. | |
virtual const csReversibleTransform & | GetWorldToCamera ()=0 |
Get the current world to camera transform. | |
virtual void | GetWriteMask (bool &red, bool &green, bool &blue, bool &alpha) const =0 |
Get the masking of color and/or alpha values to framebuffer. | |
virtual float | GetZBuffValue (int x, int y)=0 |
Get Z-buffer value at given X,Y position. | |
virtual csZBufMode | GetZMode ()=0 |
Get the z buffer write/test mode. | |
virtual bool | HasNearPlane () const =0 |
Return true if we have a near plane. | |
virtual bool | Open ()=0 |
Open the 3D graphics display. | |
virtual void | OpenPortal (size_t numVertices, const csVector2 *vertices, const csPlane3 &normal, csFlags flags)=0 |
Enter a new portal. | |
virtual bool | PerformExtension (char const *command,...)=0 |
Perform a system specific exension. | |
virtual bool | PerformExtensionV (char const *command, va_list)=0 |
Perform a system specific exension. | |
virtual void | Print (csRect const *area)=0 |
Print the image in backbuffer. | |
virtual void | RemoveFromCache (iRendererLightmap *rlm)=0 |
Remove some polygon from the cache. | |
virtual void | ResetNearPlane ()=0 |
Reset near clip plane (i.e. | |
virtual void | SetClipper (iClipper2D *clipper, int cliptype)=0 |
Set optional clipper to use. | |
virtual void | SetDimensions (int width, int height)=0 |
Change the dimensions of the display. | |
virtual void | SetNearPlane (const csPlane3 &pl)=0 |
Set near clip plane. | |
virtual bool | SetOption (const char *, const char *)=0 |
Set a renderer specific option. | |
virtual void | SetPerspectiveAspect (float aspect)=0 |
Set aspect ratio for perspective projection. | |
virtual void | SetPerspectiveCenter (int x, int y)=0 |
Set center of projection for perspective projection. | |
virtual bool | SetRenderState (G3D_RENDERSTATEOPTION op, long val)=0 |
Set a renderstate value. | |
virtual void | SetRenderTarget (iTextureHandle *handle, bool persistent=false, int subtexture=0)=0 |
Set the target of rendering. | |
virtual void | SetShadowState (int state)=0 |
Controls shadow drawing. | |
virtual void | SetTextureState (int *units, iTextureHandle **textures, int count)=0 |
Activate or deactivate all given textures depending on the value of 'textures' for that unit (i.e. | |
virtual void | SetWorldToCamera (const csReversibleTransform &w2c)=0 |
Set the world to camera transform. | |
virtual void | SetWriteMask (bool red, bool green, bool blue, bool alpha)=0 |
Set the masking of color and/or alpha values to framebuffer. | |
virtual void | SetZMode (csZBufMode mode)=0 |
Set the z buffer write/test mode. |
Detailed Description
This is the standard 3D graphics interface.All 3D graphics rasterizer servers for Crystal Space should implement this interface, as well as the iGraphics2D interface. The standard implementation is csGraphics3DSoftware.
Main creators of instances implementing this interface:
- OpenGL Renderer plugin (crystalspace.graphics3d.opengl)
- Software Renderer plugin (crystalspace.graphics3d.software)
- Null 3D Renderer plugin (crystalspace.graphics3d.null)
Main ways to get pointers to this interface:
Definition at line 701 of file graph3d.h.
Member Function Documentation
virtual bool iGraphics3D::ActivateBuffers | ( | csVertexAttrib * | attribs, | |
iRenderBuffer ** | buffers, | |||
unsigned int | count | |||
) | [pure virtual] |
Activate all given buffers.
virtual bool iGraphics3D::ActivateBuffers | ( | csRenderBufferHolder * | holder, | |
csRenderBufferName | mapping[CS_VATTRIB_SPECIFIC_LAST+1] | |||
) | [pure virtual] |
Activate the buffers in the default buffer holder.
virtual bool iGraphics3D::BeginDraw | ( | int | DrawFlags | ) | [pure virtual] |
Start a new frame (see CSDRAW_XXX bit flags).
virtual void iGraphics3D::Close | ( | ) | [pure virtual] |
Close the 3D graphics display.
virtual void iGraphics3D::ClosePortal | ( | ) | [pure virtual] |
Close a portal previously opened with OpenPortal().
If 'zfill_portal' then the portal area will be zfilled.
virtual iHalo* iGraphics3D::CreateHalo | ( | float | iR, | |
float | iG, | |||
float | iB, | |||
unsigned char * | iAlpha, | |||
int | iWidth, | |||
int | iHeight | |||
) | [pure virtual] |
Create a halo of the specified color and return a handle.
virtual void iGraphics3D::DeactivateBuffers | ( | csVertexAttrib * | attribs, | |
unsigned int | count | |||
) | [pure virtual] |
Deactivate all given buffers.
If attribs is 0, all buffers are deactivated;
virtual void iGraphics3D::DisableZOffset | ( | ) | [pure virtual] |
Disables offsetting of Z values.
virtual void iGraphics3D::DrawLine | ( | const csVector3 & | v1, | |
const csVector3 & | v2, | |||
float | fov, | |||
int | color | |||
) | [pure virtual] |
Draw a line in camera space.
Warning! This is a 2D operation and must be called while in BeginDraw(CSDRAW_2DGRAPHICS)!
virtual void iGraphics3D::DrawMesh | ( | const csCoreRenderMesh * | mymesh, | |
const csRenderMeshModes & | modes, | |||
const iShaderVarStack * | stacks | |||
) | [pure virtual] |
Drawroutine. Only way to draw stuff.
virtual void iGraphics3D::DrawPixmap | ( | iTextureHandle * | hTex, | |
int | sx, | |||
int | sy, | |||
int | sw, | |||
int | sh, | |||
int | tx, | |||
int | ty, | |||
int | tw, | |||
int | th, | |||
uint8 | Alpha = 0 | |||
) | [pure virtual] |
Draw a pixmap using a rectangle from given texture.
The sx,sy(sw,sh) rectangle defines the screen rectangle within which the drawing is performed (clipping rectangle is also taken into account). The tx,ty(tw,th) rectangle defines a subrectangle from texture which should be painted. If the subrectangle exceeds the actual texture size, texture coordinates are wrapped around (e.g. the texture is tiled). The Alpha parameter defines the transparency of the drawing operation, 0 means opaque, 255 means fully transparent.
WARNING: Tiling works only with textures that have power-of-two sizes! That is, both width and height should be a power-of-two, although not neccessarily equal.
virtual void iGraphics3D::DrawSimpleMesh | ( | const csSimpleRenderMesh & | mesh, | |
uint | flags = 0 | |||
) | [pure virtual] |
Draw a csSimpleRenderMesh on the screen.
Simple render meshes are intended for cases where setting up a render mesh and juggling with render buffers would be too much effort - e.g. when you want to draw a single polygon on the screen.
DrawSimpleMesh () hides the complexity of csRenderMesh, it cares about setting up render buffers, activating the texture etc. Note that you can still provide shaders and shader variables, but those are optional.
- Parameters:
-
mesh The mesh to draw. flags Drawing flags, a combination of csSimpleMeshFlags values.
- Remarks:
- This operation can also be called from 2D mode. In this case, the csSimpleMeshScreenspace flag should be specified. If this is not the case, you are responsible for the mess that is likely created.
virtual void iGraphics3D::EnableZOffset | ( | ) | [pure virtual] |
Enables offsetting of Z values.
virtual void iGraphics3D::FinishDraw | ( | ) | [pure virtual] |
End the frame and do a page swap.
virtual const csGraphics3DCaps* iGraphics3D::GetCaps | ( | ) | const [pure virtual] |
Get the current driver's capabilities.
Each driver implements their own function.
virtual iClipper2D* iGraphics3D::GetClipper | ( | ) | [pure virtual] |
Get clipper that was used.
virtual int iGraphics3D::GetClipType | ( | ) | const [pure virtual] |
Return type of clipper.
virtual iGraphics2D* iGraphics3D::GetDriver2D | ( | ) | [pure virtual] |
Retrieve the associated canvas.
- Remarks:
- This will return a valid canvas only after csInitializer::OpenApplication() has been invoked (and if the canvas plugin loaded and initialized successfully); otherwise it will return null.
virtual int iGraphics3D::GetHeight | ( | ) | const [pure virtual] |
Get drawing buffer height.
virtual const csPlane3& iGraphics3D::GetNearPlane | ( | ) | const [pure virtual] |
Get near clip plane.
virtual float iGraphics3D::GetPerspectiveAspect | ( | ) | const [pure virtual] |
Get aspect ratio.
virtual void iGraphics3D::GetPerspectiveCenter | ( | int & | x, | |
int & | y | |||
) | const [pure virtual] |
Get perspective center.
- Remarks:
- The coordinates are vertically mirrored in comparison to screen space, i.e. y=0 is at the bottom of the viewport, y=GetHeight() at the top.
virtual long iGraphics3D::GetRenderState | ( | G3D_RENDERSTATEOPTION | op | ) | const [pure virtual] |
Get a renderstate value.
virtual iTextureHandle* iGraphics3D::GetRenderTarget | ( | ) | const [pure virtual] |
Get the current render target (0 for screen).
virtual iTextureManager* iGraphics3D::GetTextureManager | ( | ) | [pure virtual] |
Retrieve the texture manager.
- Remarks:
- This will return a valid texture manager only after csInitializer::OpenApplication() has been invoked; otherwise it will return null.
virtual int iGraphics3D::GetWidth | ( | ) | const [pure virtual] |
Get drawing buffer width.
virtual const csReversibleTransform& iGraphics3D::GetWorldToCamera | ( | ) | [pure virtual] |
Get the current world to camera transform.
- Remarks:
- 'this' space is world space, 'other' space is camera space
virtual void iGraphics3D::GetWriteMask | ( | bool & | red, | |
bool & | green, | |||
bool & | blue, | |||
bool & | alpha | |||
) | const [pure virtual] |
Get the masking of color and/or alpha values to framebuffer.
virtual float iGraphics3D::GetZBuffValue | ( | int | x, | |
int | y | |||
) | [pure virtual] |
Get Z-buffer value at given X,Y position.
virtual csZBufMode iGraphics3D::GetZMode | ( | ) | [pure virtual] |
Get the z buffer write/test mode.
virtual bool iGraphics3D::HasNearPlane | ( | ) | const [pure virtual] |
Return true if we have a near plane.
virtual bool iGraphics3D::Open | ( | ) | [pure virtual] |
Open the 3D graphics display.
virtual void iGraphics3D::OpenPortal | ( | size_t | numVertices, | |
const csVector2 * | vertices, | |||
const csPlane3 & | normal, | |||
csFlags | flags | |||
) | [pure virtual] |
Enter a new portal.
If 'flags' contains CS_PORTAL_FLOAT then this routine will restrict all further drawing to the given 2D area and it will also respect the current contents of the Z-buffer so that geometry will only render where the Z-buffer allows it (even if zfill or znone is used). Remember to close a portal later using ClosePortal(). Basically this represents a stacked layer of portals. Each subsequent portal must be fully contained in the previous ones.
virtual bool iGraphics3D::PerformExtension | ( | char const * | command, | |
... | ||||
) | [pure virtual] |
Perform a system specific exension.
The command is a string; any arguments may follow. There is no way to guarantee the uniquiness of commands, so please try to use descriptive command names rather than "a", "b" and so on...
virtual bool iGraphics3D::PerformExtensionV | ( | char const * | command, | |
va_list | ||||
) | [pure virtual] |
Perform a system specific exension.
Just like PerformExtension() except that the command arguments are passed as a `va_list'.
virtual void iGraphics3D::Print | ( | csRect const * | area | ) | [pure virtual] |
Print the image in backbuffer.
The area parameter is only a hint to the renderer. Changes outside the rectangle may or may not be printed as well.
virtual void iGraphics3D::RemoveFromCache | ( | iRendererLightmap * | rlm | ) | [pure virtual] |
Remove some polygon from the cache.
You have to call this function before deleting a polygon (csPolygon3D destructor will do that).
virtual void iGraphics3D::ResetNearPlane | ( | ) | [pure virtual] |
Reset near clip plane (i.e.
disable it).
virtual void iGraphics3D::SetClipper | ( | iClipper2D * | clipper, | |
int | cliptype | |||
) | [pure virtual] |
Set optional clipper to use.
If clipper == null then there is no clipper. Currently only used by DrawTriangleMesh.
virtual void iGraphics3D::SetDimensions | ( | int | width, | |
int | height | |||
) | [pure virtual] |
Change the dimensions of the display.
virtual void iGraphics3D::SetNearPlane | ( | const csPlane3 & | pl | ) | [pure virtual] |
Set near clip plane.
The plane is in camera space.
virtual bool iGraphics3D::SetOption | ( | const char * | , | |
const char * | ||||
) | [pure virtual] |
Set a renderer specific option.
Returns false if renderer doesn't support that option.
virtual void iGraphics3D::SetPerspectiveAspect | ( | float | aspect | ) | [pure virtual] |
Set aspect ratio for perspective projection.
virtual void iGraphics3D::SetPerspectiveCenter | ( | int | x, | |
int | y | |||
) | [pure virtual] |
Set center of projection for perspective projection.
- Remarks:
- The coordinates are vertically mirrored in comparison to screen space, i.e. y=0 is at the bottom of the viewport, y=GetHeight() at the top.
virtual bool iGraphics3D::SetRenderState | ( | G3D_RENDERSTATEOPTION | op, | |
long | val | |||
) | [pure virtual] |
Set a renderstate value.
virtual void iGraphics3D::SetRenderTarget | ( | iTextureHandle * | handle, | |
bool | persistent = false , |
|||
int | subtexture = 0 | |||
) | [pure virtual] |
Set the target of rendering.
If this is 0 then the target will be the main screen. Otherwise it is a texture. After calling g3d->FinishDraw() the target will automatically be reset to 0 (main screen). Note that on some implementions rendering on a texture will overwrite the screen. So you should only do this BEFORE you start rendering your frame.
- Parameters:
-
persistent If this is true then the current contents of the texture will be copied on screen before drawing occurs (in the first call to BeginDraw). Otherwise it is assumed that you fully render the texture. subtexture this specifies the subtexture index if the texture is a cubemap. It is in the range 0 to 5.
virtual void iGraphics3D::SetShadowState | ( | int | state | ) | [pure virtual] |
Controls shadow drawing.
virtual void iGraphics3D::SetTextureState | ( | int * | units, | |
iTextureHandle ** | textures, | |||
int | count | |||
) | [pure virtual] |
Activate or deactivate all given textures depending on the value of 'textures' for that unit (i.e.
deactivate if 0).
virtual void iGraphics3D::SetWorldToCamera | ( | const csReversibleTransform & | w2c | ) | [pure virtual] |
Set the world to camera transform.
This affects rendering in DrawMesh and DrawSimpleMesh.
- Remarks:
- 'this' space is world space, 'other' space is camera space
virtual void iGraphics3D::SetWriteMask | ( | bool | red, | |
bool | green, | |||
bool | blue, | |||
bool | alpha | |||
) | [pure virtual] |
Set the masking of color and/or alpha values to framebuffer.
virtual void iGraphics3D::SetZMode | ( | csZBufMode | mode | ) | [pure virtual] |
Set the z buffer write/test mode.
The documentation for this struct was generated from the following file:
- ivideo/graph3d.h
Generated for Crystal Space by doxygen 1.4.7