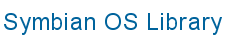
![]() |
![]() |
|
Location:
BasicAnimation.h
Link against: animation.lib
class CBasicAnimation : public CAnimation, public MAnimationDrawer, public MAnimationDataProviderObserver;
Implementation of CAnimation
for purely client side animations.
A basic animation accepts a data provider during construction, loads the appropriate animator plugin, and implements the interface
defined in CAnimation
.
A basic animation must be associated with an RWindow
. Redraw events will be received by the client application whenever the animation needs to draw a new frame, and it is the
applications responsibility to call Draw()
while handling these events. It is also the client applications responsibility to handle visibility events and place the
animation on hold when it isn't visible. This saves on CPU usage and ultimately prolongs battery life.
MAnimationDataProviderObserver
- Interface from a data provider to an animation
MAnimationDrawer
- Interface used by an animator to during the rendering process
CBase
- Base class for all classes to be instantiated on the heap
CAnimation
- Pure virtual base class for animations
CBasicAnimation
- Implementation of
Defined in CBasicAnimation
:
AnimatorDataType()
, AnimatorDraw()
, AnimatorInitialisedL()
, AnimatorResetL()
, AnimatorTicker()
, CBasicAnimation_Reserved1()
, CBasicAnimation_Reserved2()
, DataProvider()
, DataProviderEventL()
, Draw()
, Freeze()
, Hold()
, NewL()
, NewL()
, NewL()
, NewL()
, Pause()
, Position()
, Resume()
, SetHostL()
, SetPosition()
, Size()
, Start()
, Stop()
, Unfreeze()
, Unhold()
, ~CBasicAnimation()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from MAnimationDataProviderObserver
:
MAnimationDataProviderObserver_Reserved1()
,
MAnimationDataProviderObserver_Reserved2()
Inherited from MAnimationDrawer
:
MAnimationDrawer_Reserved1()
,
MAnimationDrawer_Reserved2()
static IMPORT_C CBasicAnimation *NewL(CAnimationDataProvider *aDataProvider, const TPoint &aPoint, RWsSession &aWs, RWindow
&aWindow, MAnimationObserver *aObserver=0);
Two stage constructor.
This creates and returns a new basic animation.
|
|
static IMPORT_C CBasicAnimation *NewL(CAnimationDataProvider *aDataProvider, const TPoint &aPoint, RWsSession &aWs, RWindow
&aWindow, const TDesC8 &aDataType, MAnimationObserver *aObserver=0);
Two stage constructor.
This is identical to the other NewL except that it allows an alternative data type to be specified. Unless you are trying to use a custom animator class the other form of constructor should be used.
|
|
static IMPORT_C CBasicAnimation *NewL(CAnimationDataProvider *aDataProvider, const TPoint &aPoint, MAnimationObserver *aObserver,
const CCoeControl *aHost);
Two stage constructor. This creates and returns a new basic animation.
|
|
static IMPORT_C CBasicAnimation *NewL(CAnimationDataProvider *aDataProvider, const TPoint &aPoint, const TDesC8 &aDataType,
MAnimationObserver *aObserver, const CCoeControl *aHost);
Two stage constructor.
This is identical to the other NewL except that it allows an alternative data type to be specified. Unless you are trying to use a custom animator class the other form of constructor should be used.
|
|
IMPORT_C void Draw(CWindowGc &aGc) const;
This function should be called with a valid graphics context whenever a redraw event is received for the window this animation resides in.
The graphics context attributes will not be modified, which means effects such as opacity can be applied to it before the call to Draw.
|
inline CAnimationDataProvider *DataProvider();
Provides an CAnimationDataProvider
interface to the client application.
|
inline const TPoint &Position() const;
Returns the current drawing position.
|
inline const TSize &Size() const;
Gets the size of the smallest bounding rectangle that will be required to render the animation.
This function is called when the animator is ready to begin animating. The animator cannot be started until it has called this function.
|
virtual void Start(const TAnimationConfig &aConfig);
Implements CAnimation::Start
.
|
virtual void SetPosition(const TPoint &aPoint);
Implements CAnimation::SetPosition
.
|
IMPORT_C void SetHostL(const CCoeControl *aHost);
This function lets you set the host at a later stage if you haven't got access to it during construction, or if you for some reason need to change it in run time.
|
private: virtual void DataProviderEventL(TInt aEvent, TAny *aData, TInt aDataSize);
Receives an event from a data provider.
|
private: virtual void AnimatorDraw();
Called by the animator when it needs to draw a new frame
private: virtual void AnimatorInitialisedL(const TSize &aSize);
Called by the animator when it is ready to begin running.
|
private: virtual void AnimatorResetL();
Called by the animator when it is no longer ready, usually in response to TAnimationEvent::EAnimationDataChanged
private: virtual const TPtrC8 AnimatorDataType() const;
Called by the animator plugin loading routine to determine the type of data for which an animator is required.
|
private: virtual CAnimationTicker &AnimatorTicker();
Called by the animator to obtain an AnimatorTicker, to which it will add itself whenever it is running.
|