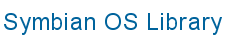
![]() |
![]() |
|
Location:
freespacechecker.h
Link against: serversocket.lib
namespace conn
the connect namespace
Defined in conn
:
CClientSocket
, CCommand
, CMessage
, CServerSocket
, DeserialiseL()
, DeserialiseL()
, EBig
, ELittle
, IsFreeSpaceAvailableL()
, IsFreeSpaceAvailableL()
, MCommand
, MFactory
, MHeader
, MMessage
, MServerSocketObserver
, Pack()
, PackImpl()
, PackImpl()
, RServiceBrokerClient
, Serialise()
, Serialise()
, TEndian
, TFreeSpaceCache
, TServerInfo
, UnpackImplL()
, UnpackImplL()
, UnpackL()
IMPORT_C void Serialise(const TAny *aType, TInt aSize, TDes8 &aBuffer, const TEndian &aEndian);
Appends data to the buffer using either BIG or LITTLE endian ordering.
|
|
IMPORT_C void DeserialiseL(TAny *aType, TInt aSize, TDes8 &aBuffer, const TEndian &aEndian);
Extracts data from the buffer into the address specified, using either BIG or SMALL endian ordering. The data extracted is removed from the buffer.
|
|
IMPORT_C TBool IsFreeSpaceAvailableL(RFs &aFileServ, TInt aDrive, TInt aBytesRequired);
This method provides an example implementation of the IsFreeSpaceAvailable operation which is used by the connectivity subsystem to prevent connect operations filling the disk/flash below a certain safety threshold.
You should update this method for your specific device and hardware requirements. It will be called before any connectivity operation which writes to the file system.
|
|
|
IMPORT_C void Serialise(const TDesC &aString, TDes8 &aBuffer, const TEndian &aEndian);
Stores the string into the buffer using either BIG or SMALL endian ordering. The length of the string is stored in the first two bytes of the buffer.
|
|
IMPORT_C void DeserialiseL(TDes &aString, TDes8 &aBuffer, const TEndian &aEndian);
Extracts the string from the buffer.
|
|
IMPORT_C TBool IsFreeSpaceAvailableL(RFs &aFileServ, TInt aDrive, TInt aBytesRequired, TFreeSpaceCache *aCache);
This method provides an example implementation of the IsFreeSpaceAvailable operation which is used by the connectivity subsystem to prevent connect operations filling the disk/flash below a certain safety threshold.
You should update this method for your specific device and hardware requirements. It will be called before any connectivity operation which writes to the file system.
If desired, a more complex implementation may be inserted here so that the call to RFs::Volume
does not have to be executed every time this method is called, based on the data stored in the TFreeSpaceCache
object.
This object must also be updated here. The following is a simple example which is NOT guaranteed to prevent the disk filling up, merely to illustrate the concept
|
|
|
inline void PackImpl(const TDesC &aType, TDes8 &aBuffer, const TEndian &aEndian, Int2Type< true >);
Calls the serialise method for strings
|
inline void UnpackImplL(TDes &aType, TDes8 &aBuffer, const TEndian &aEndian, Int2Type< true >);
Calls the deserialise method for strings
|
inline void PackImpl(const T &aType, TDes8 &aBuffer, const TEndian &aEndian, Int2Type< false >);
Calls the serialise method for generic types
|
inline void UnpackImplL(T &aType, TDes8 &aBuffer, const TEndian &aEndian, Int2Type< false >);
Calls the deserialise method for generic types
|
inline void Pack(const T &aType, TDes8 &aBuffer, const TEndian &aEndian);
Packs any type into the buffer, including strings.
Performs a test to determine if the template arugment can be converted to a TDesC, i.e. is a string. It then uses Int2Type
to call the correct implementation of PackImpl()
, either the one for strings or the one for any other type.
Note: Unfortunately the implementation of CanBeConvertedTo
is not completed because of a problem with the gcc compiler. The test "sizeof(I::Test(aType)) == sizeof(I::Small)" should
be encapsulated into an enum inside CanBeConvertedTo
but the gnu compiler complains that there is not "Test" method, although there is one. Therefore there is this meaningless
test on the sizeof as opposite to something like "CanBeConvertedTo::Yes", which would evalutate to 1 if it is convertable
and to 0 otherwise.
|
inline void UnpackL(T &aType, TDes8 &aBuffer, const TEndian &aEndian);
Unpacks any type from a buffer, including strings. More details in the documentation of Pack()
.
|
class MMessage;
The message class.
Receives message header and data from the client socket.
Defined in conn::MMessage
:
Data()
, IsReceiveComplete()
, IsSendComplete()
, Receive()
, ReceiveCompleteL()
, Reset()
, Send()
, SendCompleteL()
, ~MMessage()
~MMessage()
inline virtual ~MMessage();
Empty virtual destructor to avoid memory leaks
Data()
virtual TDes8 &Data()=0;
This returns a pointer to the next data to be sent
|
Receive()
virtual void Receive()=0;
Initiates or resumes a receive operation.
ReceiveCompleteL()
virtual void ReceiveCompleteL()=0;
Called when a previous receive has completed. The state machine should be progressed in this function.
IsReceiveComplete()
virtual TBool IsReceiveComplete() const=0;
Returns true if the whole receive operation (header and body) has completed.
|
Send()
virtual void Send()=0;
Initiates or resumes a send operation (header and body).
SendCompleteL()
virtual void SendCompleteL()=0;
A partial send has completed.
IsSendComplete()
virtual TBool IsSendComplete() const=0;
Returns true if the whole send operation (header and body) has completed.
|
Reset()
virtual void Reset()=0;
Resets an ongoing send or receive operation. Cancels any data content in the message body.
class MHeader;
The message header.
Abstract class that allows library clients to use CMessage
instead of re-implementing the MMessage
interface.
Defined in conn::MHeader
:
BodyLength()
, MaxBodyLength()
, PrepareToWrite()
, ReadL()
, ReadPtr()
, Reset()
, SetBodyLength()
, WritePtr()
, ~MHeader()
~MHeader()
inline virtual ~MHeader();
Empty virtual destructor to avoid memory leaks
ReadPtr()
virtual const TDes8 &ReadPtr() const=0;
The pointer for reading data from the socket The buffer returned can be partially filled. The message class will try to read starting at the current length of this buffer up to its maximum length.
|
ReadL()
virtual TBool ReadL(TUint aReadBytes)=0;
The header is notified when the read operation completes, the number of bytes that have been received is passed as a parameter. The header can do any unpacking of data in here.
|
|
PrepareToWrite()
virtual void PrepareToWrite()=0;
The header should get ready to send data, i.e. do any packing in here.
WritePtr()
virtual const TDes8 &WritePtr() const=0;
The pointer for writing data to the socket, data will be written up to the current length of this buffer.
|
Reset()
virtual void Reset()=0;
The current send or read operation is aborted
SetBodyLength()
virtual void SetBodyLength(TInt aLength)=0;
Sets the length of the message body, called by CMessage
immediately before calling PrepareToWrite()
|
BodyLength()
virtual TInt BodyLength() const=0;
Returns the length of the message body
Note: I know the return should be unsigned but having it signed avoids warning due to descriptor's length being signed.
|
MaxBodyLength()
virtual TInt MaxBodyLength() const=0;
Returns the maximum allowed length of the message body
Note: I know the return should be unsigned but having it signed avoids warning due to descriptor's length being signed.
|
class TFreeSpaceCache;
This class is used to store a cache of the previous results of an IsFreeSpaceAvailable operation within a component which may call IsFreeSpaceAvailable again. It is provided so that specific implementations of IsFreeSpaceAvailable can provide performance enhancements based on the disk space situation after a previous call if desired.
Defined in conn::TFreeSpaceCache
:
iBytesFree
, iDriveId
, iDriveSize
, iLastChecked
iDriveId
TInt iDriveId;
The ID of the drive
iBytesFree
TInt iBytesFree;
A count of the remaining free bytes
iLastChecked
TInt64 iLastChecked;
Used to determine whether or not to do an actual check
iDriveSize
TInt iDriveSize;
This attribute is unused
class MServerSocketObserver;
The observer of the server socket, gets notified of events relative to the server socket.
Defined in conn::MServerSocketObserver
:
ServerSocketStoppedDueToErr()
ServerSocketStoppedDueToErr()
virtual void ServerSocketStoppedDueToErr(TInt aErr)=0;
Called when the server socket is stopping due to an error
|
class MCommand;
A client command. It is created with a message, which is passed as a pointer and the command is then responsible for the memory of this message. A pointer to the client socket is also passed as a parameter in the constructor. It can process more than one message
Defined in conn::MCommand
:
ExecuteLD()
, NewMessageReceived()
, SendCompleteL()
, SendErrorMessage()
, SocketClosing()
, TakeOwnershipOfMessage()
, ~MCommand()
~MCommand()
inline virtual ~MCommand();
Empty virtual destructor to avoid memory leaks
ExecuteLD()
virtual void ExecuteLD()=0;
Executes the command, called by the client socket when the command has been created, or after an observer command has captured a message.
SendErrorMessage()
virtual void SendErrorMessage(TUint aErrorCode)=0;
Sends an error message to the remote client
|
NewMessageReceived()
virtual TBool NewMessageReceived(MMessage *aMessage)=0;
Called by the client socket when a receive operation has completed, it gives observers (i.e. commands) a chance of grabbing extra messages in addition to the one that created the command in the first place. The observer implementing this method can take ownership of the pointer parameter, in which case true should be returned. Alternatively it may ignore the message (default behaviour for all commands) in which case false should be returned.
|
|
SendCompleteL()
virtual void SendCompleteL()=0;
Called when a send operation has completed. No error is reported by the send operation because it is always assumed to succeed.
Should it fail, the socket is closed and therefore SocketClosing()
is called. Observers don't need to take any action on write failure, they simply stop to exist.
SocketClosing()
virtual void SocketClosing()=0;
Called when the socket is closing
TakeOwnershipOfMessage()
virtual void TakeOwnershipOfMessage(MMessage *aMessage)=0;
Pure virtual function to be implemented in order to take ownership of the message
|
class RServiceBrokerClient : public RSessionBase;
Client-side API for the Symbian Connect Service Broker
RHandleBase
- A handle to an object
RSessionBase
- Client-side handle to a session with a server
conn::RServiceBrokerClient
- Client-side API for the Symbian Connect Service Broker
Defined in conn::RServiceBrokerClient
:
Connect()
, Disconnect()
, FailedToStart()
, RServiceBrokerClient()
, RegisterPort()
, StopServer()
, Version()
, ~RServiceBrokerClient()
Inherited from RHandleBase
:
Attributes()
,
Close()
,
Duplicate()
,
FullName()
,
Handle()
,
HandleInfo()
,
Name()
,
SetHandle()
,
SetHandleNC()
,
iHandle
Inherited from RSessionBase
:
CreateSession()
,
EAutoAttach
,
EExplicitAttach
,
Open()
,
Send()
,
SendReceive()
,
SetReturnedHandle()
,
ShareAuto()
,
ShareProtected()
,
TAttachMode
RServiceBrokerClient()
IMPORT_C RServiceBrokerClient();
Class constructor.
~RServiceBrokerClient()
IMPORT_C ~RServiceBrokerClient();
Class default destructor.
Connect()
IMPORT_C TInt Connect();
Connects the handle to the service.
|
Disconnect()
IMPORT_C void Disconnect();
Detaches from the service.
Version()
IMPORT_C TVersion Version() const;
Getter method for the version of the Service Broker client API
|
RegisterPort()
IMPORT_C TInt RegisterPort(const TDesC &aServiceName, TUint aPortNumber);
Registers the port number corresponding to the specified service name.
|
|
FailedToStart()
IMPORT_C TInt FailedToStart(const TDesC &aServiceName, TUint aErrorCode);
Communicates to the named service server that the specified service failed the startup procedure. The reason of failure should be specified in the error code parameter, if known.
|
|
StopServer()
IMPORT_C TInt StopServer();
Works only in DEBUG builds, in RELEASE builds the server does not take action of this command, intended for debugging purposes
|
class MFactory;
The command factory.
Creates concrete instances of messages and commands.
Defined in conn::MFactory
:
NewClientL()
, NewCommandL()
, NewMessageL()
, ~MFactory()
~MFactory()
inline virtual ~MFactory();
Empty virtual destructor to avoid memory leaks
NewClientL()
virtual IMPORT_C CClientSocket *NewClientL(CServerSocket *aServerSocket);
Creates an instance of the client socket
|
|
NewCommandL()
virtual MCommand *NewCommandL(MMessage *aMessage, CClientSocket *aSocket)=0;
Creates and returns a new command
|
|
NewMessageL()
virtual MMessage *NewMessageL()=0;
Creates and returns a new message
|
class CMessage : public CBase, public conn::MMessage;
The message class.
Receives message header and data from the client socket. Gives access to the data and message ID to command classes. Commands extract information from the message data buffer and package response information into the message data buffer.
conn::MMessage
- The message class
CBase
- Base class for all classes to be instantiated on the heap
conn::CMessage
- The message class
Defined in conn::CMessage
:
Append()
, CMessage()
, ConstructL()
, Data()
, DataBuf()
, DataBuf()
, DataPtr()
, DataPtr()
, ENull
, EReceiveComplete
, EReceiveData
, EReceiveHdr
, ESendComplete
, ESendData
, ESendHdr
, Endian()
, ExtractDataL()
, ExtractL()
, ExtractL()
, ExtractLC()
, ExtractRawDataBlockL()
, ExtractRawDataBlockLC()
, ExtractRawDataL()
, ExtractWithRawLenPrefixLC()
, Header()
, Header()
, IsReceiveComplete()
, IsSendComplete()
, Length()
, NewL()
, PeekL()
, Receive()
, ReceiveCompleteL()
, Reset()
, Send()
, SendCompleteL()
, State()
, State()
, TState
, ~CMessage()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
NewL()
static IMPORT_C CMessage *NewL(MHeader *aHeader, const TEndian &aEndian, CMessage *aMessage=0);
The factory method. By default the parameter is NULL, this signals default construction. If the parameter is not NULL it means
we are cloned from another message. This is not yet implmented and will panic when passed a non-NULL CMessage
.
|
|
~CMessage()
IMPORT_C ~CMessage();
The class destructor, delete the data buffer.
CMessage()
protected: IMPORT_C CMessage(const TEndian &aEndian);
Class constructor
|
ConstructL()
protected: virtual IMPORT_C void ConstructL(CMessage *aMessage, MHeader *aHeader);
Constructs the message. The received parameter by default is null (default construction). If the parameter pointer is not
null, this is a copy operation and the data of the parameter is copied (copy construction). This is not yet implmented and
will panic when passed a non-NULL CMessage
.
The data buffer is allocated to the maximum possible length as specified by the header. This might be inefficient and we might need to change this or allow specialised implementations to override this.
|
|
Reset()
virtual IMPORT_C void Reset();
Set the state to null and reset the data buffer
Data()
virtual IMPORT_C TDes8 &Data();
Gives writable access to the data. Used by the client socket for receiving data. Allows segmented receiving of data.
When our state is either EReceiveHdr or EReceiveData this method is called in order to retrieve a buffer for the socket read
calls. This is ensured by IsReceiving() returning true. When we are receiving the header, we return a TPtr8
pointing to the header buffer. The position inside this buffer is determined by the data already received - set in ReceiveCompleteL()
. Likewise when receiving data we return the data buffer, with the position determined by the data already received.
When our state is either ESendHdr or ESendData this method is called in order to retrieve a buffer for the socket write calls.
This is ensured by IsSending() returning true. We send the header first, by returning a pointer to the beginning of the header
buffer. Then we send the data, by returning a pointer to the beginning of the data buffer. SendCompleteL()
ensures that we do not try to send any data when the length of the data is zero.
|
Receive()
virtual IMPORT_C void Receive();
Signals that we are in the receving phase, this is true until Reset()
is called.
ReceiveCompleteL()
virtual IMPORT_C void ReceiveCompleteL();
Data has been received by the socket. The socket used the data returned by CMessage::Data()
for receiving. Therefore, depending on our state, either the header or the message data has been received. If the header
has been received, unpack the header content and set the state to receiving data. If the data has been received, set the state
to receive complete.
IsReceiveComplete()
virtual IMPORT_C TBool IsReceiveComplete() const;
Returns true if the state is EReceiveComplete, false otherwise. Signals to the client socket that the message has received all of the data required.
|
Send()
virtual IMPORT_C void Send();
Signals that we are sending data, starting with the header. This is true until Reset()
is called. Also packs the header content and sets the state to ESendHdr.
SendCompleteL()
virtual IMPORT_C void SendCompleteL();
Data has been sent by the socket.
|
IsSendComplete()
virtual IMPORT_C TBool IsSendComplete() const;
Signals that data has been successfully sent.
|
Append()
inline void Append(const T &aData);
Appends aData to the data trailer
|
ExtractL()
inline void ExtractL(T &aData);
Extracts aData from the data trailer
|
PeekL()
inline void PeekL(T &aData) const;
Peeks aData from the data trailer
|
|
ExtractLC()
IMPORT_C HBufC *ExtractLC();
Extracts a string from the data buffer, and returns it. The string is stored in an HBufC, which is put on the cleanup stack
|
|
ExtractL()
IMPORT_C HBufC *ExtractL();
Extracts a string from the data buffer, and returns it. The string is stored in an HBufC and not left on the cleanup stack.
|
ExtractWithRawLenPrefixLC()
IMPORT_C HBufC *ExtractWithRawLenPrefixLC();
Extracts a unicode string which is prefixed by its size in bytes from the data buffer, and returns it. The string is stored in an HBufC, which is put on the cleanup stack
|
|
ExtractDataL()
IMPORT_C HBufC8 *ExtractDataL();
Extracts raw data from a message and returns a HBufC8
pointer to it
|
|
ExtractRawDataL()
IMPORT_C TPtrC8 ExtractRawDataL();
Extracts raw data from the data buffer, it returns a pointer to the start of the data.
|
|
ExtractRawDataBlockLC()
IMPORT_C HBufC8 *ExtractRawDataBlockLC();
Extracts a raw data block from a message and returns a HBufC8
pointer to it
|
|
ExtractRawDataBlockL()
IMPORT_C HBufC8 *ExtractRawDataBlockL();
Extracts a raw data block from a message and returns a HBufC8
pointer to it and is not left on the cleanup stack
|
Length()
inline TUint Length();
Getter for the length of the messgae body
|
Header()
inline MHeader *&Header();
Returns a reference to the message header pointer
|
Header()
inline const MHeader *const &Header() const;
Returns a const referenct to the message header pointer
|
State()
protected: inline TState &State();
Returns a reference to the state of the message
|
State()
protected: inline const TState &State() const;
Returns a const reference to the state of the message
|
DataBuf()
protected: inline HBufC8 *&DataBuf();
Returns a pointer reference to the body data
|
DataBuf()
protected: inline const HBufC8 *const &DataBuf() const;
Returns a const pointer reference to the body data
|
DataPtr()
protected: inline TPtr8 &DataPtr();
Returns the current pointer to the message body data. The position of this pointer depends on the message state
|
DataPtr()
protected: inline const TPtr8 &DataPtr() const;
Returns the current pointer to the message body data. The position of this pointer depends on the message state
|
Endian()
protected: inline const TEndian &Endian() const;
Returns the endianess as defined in serialise.h, by default this is big endian
|
TState
TState
The possible states for this class
|
class CCommand : public CBase, public conn::MCommand;
A client command. It is created with a message, which is passed as a pointer and the command is then responsible for the memory
of this message. A pointer to the client socket is also passed as a parameter in the constructor. Although usually not needed,
it can process more than one message by registering itself as an observer of the client socket. It forwards a request to the
file server and then packages the answer into a message, which is sent to the client via the client socket. It is created
by the command factory, TFactory
.
conn::MCommand
- A client command
CBase
- Base class for all classes to be instantiated on the heap
conn::CCommand
- A client command
Defined in conn::CCommand
:
CCommand()
, Message()
, Message()
, NewMessageReceived()
, SendCompleteL()
, SendResponse()
, Socket()
, SocketClosing()
, TakeOwnershipOfMessage()
, ~CCommand()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from conn::MCommand
:
ExecuteLD()
,
SendErrorMessage()
CCommand()
protected: IMPORT_C CCommand(CClientSocket *aSocket);
The class constructor. This command is added to the observers of the client socket.
|
~CCommand()
protected: virtual IMPORT_C ~CCommand();
Class destructor
SendResponse()
protected: virtual IMPORT_C void SendResponse();
Sends a response message to the client via the connected socket.
SendCompleteL()
protected: virtual IMPORT_C void SendCompleteL();
The reply message has been sent, by default the command deletes itself in this method.
NewMessageReceived()
protected: virtual IMPORT_C TBool NewMessageReceived(MMessage *aMessage);
Called by the client socket when a new message is received. This should be implemented only by those command that receive the client request in more than one message, i.e. file copy.
|
|
SocketClosing()
protected: virtual IMPORT_C void SocketClosing();
Socket closing, need to delete self
TakeOwnershipOfMessage()
protected: virtual IMPORT_C void TakeOwnershipOfMessage(MMessage *aMessage);
Takes ownership of the message passed in
|
Socket()
protected: inline CClientSocket *Socket() const;
Returns a pointer to the client socket
|
Message()
protected: inline CMessage *&Message();
Returns a reference to the CMessage
pointer
|
Message()
protected: inline const CMessage *const &Message() const;
Returns a const reference to the CMessage
pointer
|
class CClientSocket : public CBase;
The client socket wrapper.
This class owns a CClientSocketImpl
instance and publishes the public API of CClientSocketImpl
to the outside world. The reason for this class is twofold:
Hiding the implementation details of CClientSocket
Future binary compatibility
CBase
- Base class for all classes to be instantiated on the heap
conn::CClientSocket
- The client socket wrapper
Defined in conn::CClientSocket
:
CommandArray()
, CommandArray()
, ConstructL()
, HandleSocketError()
, NewL()
, Receive()
, ReceiveCompleteL()
, RemoveCommand()
, Send()
, SendCompleteL()
, Socket()
, Socket()
, ~CClientSocket()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
NewL()
static IMPORT_C CClientSocket *NewL(CServerSocket *aServerSocket, MFactory *aFactory);
Static factory method (two phase construction)
|
|
|
~CClientSocket()
virtual IMPORT_C ~CClientSocket();
Class destructor. Deletes the implementation.
ConstructL()
protected: virtual IMPORT_C void ConstructL(CServerSocket *aServerSocket, MFactory *aFactory);
Creates an instance of CClientSocketImpl
, the client socket.
|
Receive()
IMPORT_C void Receive();
Starts a receive operation.
Send()
IMPORT_C void Send(MCommand *aCommand, MMessage *aMessage);
Sends a message to the socket. Only one message can be sent at any one time.
|
RemoveCommand()
IMPORT_C void RemoveCommand(MCommand *aCommand);
Removes a command from the socket. Existing commands that are registered with the socket receive a notification each time a message is received. By default commands are registered with the socket when they are created. They should remove themselves either when they cease to exist (i.e. in their destructor). As long as commands are registered they will also be deleted if the client socket is disconnected.
|
ReceiveCompleteL()
IMPORT_C void ReceiveCompleteL();
Called when a message chunk has been received on the socket.
SendCompleteL()
IMPORT_C void SendCompleteL();
Called when a message chunk has been sent to the socket.
HandleSocketError()
IMPORT_C void HandleSocketError(TInt aErr);
Handles a socket error.
|
Socket()
IMPORT_C const RSocket &Socket() const;
Returns a const handle to the client socket
|
Socket()
IMPORT_C RSocket &Socket();
Returns a handle to the client socket
|
CommandArray()
IMPORT_C const RPointerArray< MCommand > &CommandArray() const;
Returns a const handle to the command array
|
CommandArray()
IMPORT_C RPointerArray< MCommand > &CommandArray();
Returns a handle to the command array
|
class TServerInfo;
Stores information regarding the server socket
Defined in conn::TServerInfo
:
TServerInfo()
, iFactory
, iMaxConnectionQueue
, iObserver
, iPortNumber
, iServiceName
TServerInfo()
inline TServerInfo(MFactory *aFactory, TUint16 aPortNumber, TUint16 aMaxConnectionQueue, const TDesC &aServiceName, MServerSocketObserver
*aObserver);
Class constructor, initialises data members
|
iFactory
MFactory * iFactory;
A concrete instance of the factory
iPortNumber
TUint16 iPortNumber;
The server port number
iMaxConnectionQueue
TUint16 iMaxConnectionQueue;
The maximum number of outstanding connection requests
iServiceName
TPtrC iServiceName;
The service name
iObserver
MServerSocketObserver * iObserver;
The observer of the server socket
class CServerSocket : public CBase;
The server socket wrapper.
This class owns an instance of CServerSocketImpl
and publishes its public API.
The reasons for this class are:
Hiding the implementation details
Future binary compatibility
CBase
- Base class for all classes to be instantiated on the heap
conn::CServerSocket
- The server socket wrapper
Defined in conn::CServerSocket
:
Cancel()
, IsActive()
, NewL()
, StartL()
, ~CServerSocket()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
NewL()
static IMPORT_C CServerSocket *NewL(const TServerInfo &aInfo);
Static factory method (two phase construction)
|
|
~CServerSocket()
IMPORT_C ~CServerSocket();
Class destructor, deletes the implementation. socket
StartL()
IMPORT_C void StartL();
Starts listening on the server socket.
Cancel()
IMPORT_C void Cancel();
Stops listening on the server socket.
IsActive()
IMPORT_C TBool IsActive();
Returns true if the server socket is listening, false otherwise
|