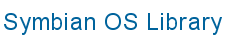
![]() |
![]() |
|
Location:
FRMTLAY.H
Link against: form.lib
class CTextLayout : public CBase;
Text layout.
CTextLayout is the lowest-level text formatting class in the Text Views API. It obtains text and formatting attributes via
the MLayDoc
interface class and formats the text to a certain width.
It has functions for drawing the text and performing hit-detection that is, converting x-y coordinates to document positions,
and vice versa. It defines many public functions that are not generally useful, but are called by the higher-level CTextView
class, or exist only for the convenience of closely associated classes like the printing and pagination systems. These are
identified in the documentation by the text "not generally useful".
When using the CTextLayout class, you must be aware of what functions have previously been called. For example:
1) Formatting and scrolling functions must not be called if a CTextView
object owns the CTextLayout object, because the CTextView
object may be reformatting the CTextLayout object asynchronously by means of an active object, or may hold some state information
about the CTextLayout object that would be invalidated by reformatting. These functions are identified in the documentation
by the text "Do not use if a CTextView object owns this CTextLayout object.".
2) Functions that raise out of memory exceptions can leave the object in an inconsistent state; these functions can be identified
as usual by their trailing L. When this occurs, it is necessary to discard the formatting information by calling DiscardFormat()
.
3) Some functions change formatting parameters like the wrap width or band height, but do not reformat to reflect the change. These functions are identified in the documentation by the text "Reformat needed".
CBase
- Base class for all classes to be instantiated on the heap
CTextLayout
- Text layout
Defined in CTextLayout
:
AdjustVerticalAlignment()
, BandHeight()
, CalculateHorizontalExtremesL()
, ChangeBandTopL()
, CustomDraw()
, CustomWrap()
, DiscardFormat()
, DocPosToXyPosL()
, DocumentLength()
, DrawBorders()
, DrawL()
, EBadCharacterEditType
, ECharacterNotFormatted
, EDrawingBorderError
, EFAllParagraphsNotWrapped
, EFAllowScrollingBlankSpace
, EFCharacterInsert
, EFDisallowScrollingBlankSpace
, EFFormatAllText
, EFFormatBand
, EFLeftDelete
, EFMaximumLineWidth
, EFNoCurrentFormat
, EFNotInCurrentFormat
, EFParagraphDelimiter
, EFParagraphsWrappedByDefault
, EFRightDelete
, EFScrollOnlyToTopsOfLines
, EFScrollRedrawWholeScreen
, EFViewDiscardAllFormat
, EFViewDontDiscardFormat
, EFormatDeviceNotSet
, EImageDeviceNotSet
, EInvalidDocPos
, EInvalidLineNumber
, EInvalidRedraw
, EMustFormatAllText
, ENoCharRangeToFormat
, ENoMemory
, EPageScrollError
, EPixelNotInFormattedLine
, EPosNotFormatted
, EPrintPreviewModeError
, EUnimplemented
, ExcludingPartialLines()
, ExtendFormattingToCoverPosL()
, FindDocPos()
, FindXyPos()
, FirstCharOnLine()
, FirstDocPosFullyInBand()
, FirstFormattedPos()
, FirstLineInBand()
, FontHeightIncreaseFactor()
, ForceNoWrapping()
, FormatBandL()
, FormatCharRangeL()
, FormatLineL()
, FormatNextLineL()
, FormattedHeightInPixels()
, FormattedLength()
, GetCharacterHeightAndAscentL()
, GetCustomInvisibleCharacterRemapper()
, GetFontHeightAndAscentL()
, GetLineNumber()
, GetLineRect()
, GetLineRectL()
, GetMinimumSizeL()
, GetMinimumSizeL()
, GetNextVisualCursorPos()
, GetOrigin()
, HandleAdditionalCharactersAtEndL()
, HandleBlockChangeL()
, HandleCharEditL()
, Highlight()
, HighlightExtensions()
, InvertRangeL()
, IsBackgroundFormatting()
, IsFormattingBand()
, IsWrapping()
, MajorVersion()
, MakeVisible()
, MinimumLineDescent()
, NewL()
, NonPrintingCharsVisibility()
, NotifyTerminateBackgroundFormatting()
, NumFormattedLines()
, PageDownL()
, PageUpL()
, ParagraphHeight()
, ParagraphRectL()
, PictureRectangleL()
, PictureRectangleL()
, PixelsAboveBand()
, PosInBand()
, PosInBand()
, PosInBand()
, PosIsFormatted()
, PosRangeInBand()
, ReformatVerticalSpaceL()
, RestrictScrollToTopsOfLines()
, ScrollLinesL()
, ScrollParagraphsL()
, SetAmountToFormat()
, SetBandHeight()
, SetCustomDraw()
, SetCustomInvisibleCharacterRemapper()
, SetCustomWrap()
, SetExcludePartialLines()
, SetFontHeightIncreaseFactor()
, SetFormatMode()
, SetImageDeviceMap()
, SetInterfaceProvider()
, SetLabelsDeviceMap()
, SetLabelsMarginWidth()
, SetLayDoc()
, SetMinimumLineDescent()
, SetNonPrintingCharsVisibility()
, SetTruncating()
, SetTruncatingEllipsis()
, SetViewL()
, SetViewL()
, SetWrapWidth()
, TAllowDisallow
, TAmountFormatted
, TCurrentFormat
, TDiscard
, TPanicNumber
, TRangeChange
, TScrollFlags
, TagmaTextLayout()
, ToParagraphStart()
, Truncating()
, TruncatingEllipsis()
, XyPosToDocPosL()
, YBottomLastFormattedLine()
, anonymous
, anonymous
, anonymous
, ~CTextLayout()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
static IMPORT_C CTextLayout *NewL(MLayDoc *aDoc, TInt aWrapWidth);
Allocates and constructs a CTextLayout object. By default, the formatting is set to the entire document (EFFormatAllText).
The text needs to be reformatted after a call to this function.
|
|
IMPORT_C void DiscardFormat();
Discards all formatting information. This function is used by the CTextView
and the printing classes, but should be called by higher-level classes that need to clean up after any CTextLayout function
has caused an out-of-memory exception.
IMPORT_C void SetLayDoc(MLayDoc *aDoc);
Sets the layout object's source text to aDoc.
The text needs to be reformatted after a call to this function.
|
IMPORT_C void SetWrapWidth(TInt aWrapWidth);
Sets the wrap width. If the current format mode is screen mode (CLayoutData::EFScreenMode
) aWrapWidth is in pixels, otherwise it is in twips.
The text needs to be reformatted after a call to this function.
Note:
A valid wrap width (>0) must be supplied or the expected amount of formatting will not take place. This could lead to panics when trying to retrieve formatting information that does not exist.
|
IMPORT_C void SetBandHeight(TInt aHeight);
Sets the height of the band in pixels or twips. This is the height of the visible text, or the view window, and it is also
the page height for the purposes of scrolling up and down by page. If the current mode is screen mode (CLayoutData::EFScreenMode
) or what-you-see-is-what-you-get mode (CLayoutData::EFWysiwygMode
), aHeight is in pixels, otherwise it is in twips.
The text needs to be reformatted after a call to this function.
Note:
A valid band height (>0) must be supplied or the expected amount of formatting will not take place. This could lead to panics when trying to retrieve formatting information that does not exist.
|
IMPORT_C TInt BandHeight() const;
Gets the height of the band in pixels or twips.
|
IMPORT_C void SetImageDeviceMap(MGraphicsDeviceMap *aGd);
Sets the device map used for drawing and formatting. This device map is also used for formatting and drawing paragraph labels
unless a separate label device map has been set (see SetLabelsDeviceMap()
).
The text needs to be reformatted after a call to this function.
Note:
Although the name of the function suggests that only the image device is set, the formatting device is also set.
|
IMPORT_C void SetLabelsDeviceMap(MGraphicsDeviceMap *aDeviceMap);
Sets the device map used for formatting and drawing paragraph labels. If not set, the device map used for labels will be the same as that used for the text.
The text needs to be reformatted after a call to this function.
|
IMPORT_C void SetAmountToFormat(TAmountFormatted aAmountOfFormat=EFFormatBand);
Sets whether to format all the text (if aAmountOfFormat is EFFormatAllText), or just the visible band (if aAmountOfFormat is EFFormatBand). If band formatting is selected, enough text is formatted to fill the visible height.
The text needs to be reformatted after a call to this function.
|
IMPORT_C TBool IsFormattingBand() const;
Tests whether band formatting is on, as set by CTextLayout::SetAmountToFormat()
.
|
IMPORT_C void SetFormatMode(CLayoutData::TFormatMode aFormatMode, TInt aWrapWidth, MGraphicsDeviceMap *aFormatDevice);
Sets the format mode and wrap width and (for certain format modes only) sets the formatting device.
The text needs to be reformatted after a call to this function.
Notes:
If aFormatMode is CLayoutData::EFWysiwygMode
or CLayoutData::EFPrintPreviewMode
, the format device is set to aFormatDevice, which must not be NULL.
If aFormatMode is CLayoutData::EFScreenMode
or CLayoutData::EFPrintMode
, aFormatDevice is ignored and should be NULL; the format device is set to the image device.
The wrap width is set in either twips or pixels using the same rule as for SetWrapWidth()
.
|
IMPORT_C void ForceNoWrapping(TBool aNoWrapping=EFAllParagraphsNotWrapped);
Turns wrapping on (if aNoWrapping is EFParagraphsWrappedByDefault) or off (if aNoWrapping is EFAllParagraphsNotWrapped). Overrides
the paragraph format when wrapping is turned off -paragraphs are not broken into lines even if the iWrap member of CParaFormat
is ETrue. If wrapping is turned on, CParaFormat::iWrap
is honoured.
The text needs to be reformatted after a call to this function.
|
IMPORT_C TBool IsWrapping() const;
Tests whether wrapping is on or off.
|
IMPORT_C void SetTruncating(TBool aOn);
Sets the truncation mode. If truncation is on, lines that exceed the wrap width, either because they have no legal line break, or because wrapping is off, are truncated, and an ellipsis is inserted.
|
IMPORT_C TBool Truncating() const;
Tests whether truncation is on (as set by SetTruncating()
).
|
IMPORT_C void SetTruncatingEllipsis(TChar aEllipsis);
Sets the ellipsis character to be used if truncation is on. Specify the value 0xFFFFÂ (the illegal Unicode character) if no ellipsis character should be used. By default, the ellipsis character is 0x2026, the ordinary horizontal ellipsis.
|
IMPORT_C TChar TruncatingEllipsis() const;
Returns the ellipsis character used when truncation is on. The value 0xFFFF (the illegal Unicode character) means that no ellipsis character is appended to truncated text.
|
IMPORT_C void SetLabelsMarginWidth(TInt aWidth);
Sets the width in pixels of the margin in which labels are drawn.
The text needs to be reformatted after a call to this function.
|
IMPORT_C void SetNonPrintingCharsVisibility(TNonPrintingCharVisibility aVisibility);
Specifies which non-printing characters (e.g. space, paragraph break, etc.) are to be drawn using symbols.
The text needs to be reformatted after a call to this function.(because non-printing characters may differ in width from their visible representations).
|
IMPORT_C TNonPrintingCharVisibility NonPrintingCharsVisibility() const;
Returns which non-printing characters are drawn using symbols.
|
IMPORT_C TBool IsBackgroundFormatting() const;
Tests whether background formatting is currently taking place. Background formatting is managed by CTextView
, using an active object, when the CTextLayout object is owned by a CTextView
object.
Not generally useful.
|
IMPORT_C void NotifyTerminateBackgroundFormatting();
CTextView
calls this function when background formatting has ended. It allows the CTextLayout object to discard information used only
during background formatting.
Not generally useful.
IMPORT_C void SetExcludePartialLines(TBool aExcludePartialLines=1);
Specifies whether partially displayed lines (at the top and bottom of the view) are to be prevented from being drawn, and whether the top of the display is to be aligned to the nearest line.
This function takes effect only when the text is next formatted or scrolled.Note:This function was designed for non-editable
text in the Agenda application, and there is an important restriction: CTextView
functions that reformat the text after editing must not be used while partial lines are excluded; these functions are CTextView::HandleCharEditL()
, CTextView::HandleInsertDeleteL()
and CTextView::HandleRangeFormatChangeL()
.
|
IMPORT_C TBool ExcludingPartialLines() const;
Tests whether partial lines at the top and bottom of the view are currently excluded.
|
IMPORT_C void SetFontHeightIncreaseFactor(TInt aPercentage);
Sets the percentage by which font heights are increased in order to provide automatic extra spacing (leading) between lines.
This amount is set to CLayoutData::EFFontHeightIncreaseFactor
, which is 7, when a CTextLayout object is created.
The text needs to be reformatted after a call to this function.
|
IMPORT_C TInt FontHeightIncreaseFactor() const;
Returns the font height increase factor as a percentage (i.e. a return value of 7 means that font heights are increased by 7% to provide automatic extra spacing between lines).
|
IMPORT_C void SetMinimumLineDescent(TInt aPixels);
Sets the minimum line descent in pixels. This amount is set to CLayoutData::EFMinimumLineDescent
, which is 3, when a CTextLayout object is created.
The text needs to be reformatted after a call to this function.
|
IMPORT_C TInt MinimumLineDescent() const;
Returns the minimum line descent in pixels.
|
IMPORT_C TInt DocumentLength() const;
Returns the document length in characters, including all the text, not just the formatted portion, but not including the final paragraph delimiter (the "end-of-text character") if any. Thus the length of an empty document is zero.
|
IMPORT_C TInt ToParagraphStart(TInt &aDocPos) const;
Sets aDocPos to the paragraph start and returns the amount by which aDocPos has changed, as a non-negative number.
|
|
IMPORT_C TInt PixelsAboveBand() const;
Returns the height in pixels of any formatted text above the visible region.
|
IMPORT_C TInt YBottomLastFormattedLine() const;
Returns the y coordinate of the bottom of the last formatted line, relative to the top of the visible region.
|
IMPORT_C TInt FormattedHeightInPixels() const;
Returns the height in pixels of the formatted text.
|
IMPORT_C TInt PosRangeInBand(TInt &aDocPos) const;
Returns the number of fully or partially visible characters in the visible band.
|
|
IMPORT_C TBool PosInBand(const TTmDocPos &aDocPos, TTmLineInfo *aLineInfo=0) const;
Tests whether the document position aDocPos is fully or partially visible. If it is, puts the baseline of the line containing aDocPos into aLineInfo.
|
|
IMPORT_C TBool PosInBand(TTmDocPos aDocPos, TPoint &aXyPos) const;
Tests whether the document position aDocPos is fully or partially visible. If it is, puts the y coordinate of the left-hand end of the baseline of the line containing aDocPos into aXyPos.
|
|
IMPORT_C TBool PosInBand(TInt aDocPos, TPoint &aXyPos) const;
Tests whether the document position aDocPos is fully or partially visible. If it is, puts the y coordinate of the left-hand end of the baseline of the line containing aDocPos into aXyPos.
|
|
IMPORT_C TBool PosIsFormatted(TInt aDocPos) const;
Tests whether the character aDocPos is formatted.
Note:
If a section of text contains characters p to q, it contains document positions p to q + 1; but this function returns ETrue for positions p to q only, so it refers to characters, not positions. However, it will return ETrue for q if q is the end of the document.
|
|
IMPORT_C TInt FirstCharOnLine(TInt aLineNo) const;
Gets the document position of the first character in the specified line, counting the first line as line one (not zero) in
the band. If the line is after the band, returns the last character position of the band. If there is no formatted text, returns
CTextLayout::EFNoCurrentFormat
.
|
|
IMPORT_C TInt FormattedLength() const;
Returns the number of formatted characters. This will be one more than expected if the formatted text runs to the end of the document, because it will include the end-of-text character.
|
IMPORT_C TInt FirstFormattedPos() const;
Returns the document position of the first formatted character.
|
IMPORT_C TInt NumFormattedLines() const;
Gets the number of formatted lines.
|
IMPORT_C TInt FirstLineInBand() const;
Returns the line number, counting from 0, of the first fully visible line.
|
IMPORT_C TInt GetLineRect(TInt aYPos, TRect &aLine) const;
Gets the rectangle enclosing the formatted line that contains or is closest to y coordinate aYPos. If aYPos is above the first
formatted line, the rectangle returned is that of the first formatted line. If aYPos is below the last formatted line the
rectangle returned is that of the last formatted line. If there is no formatted text, returns CTextLayout::EFNoCurrentFormat
.
|
|
IMPORT_C TInt ParagraphHeight(TInt aDocPos) const;
Returns the height of the paragraph containing aDocPos. If the paragraph is not formatted, returns zero. If the paragraph is partially formatted, returns the height of the formatted part.
|
|
IMPORT_C TRect ParagraphRectL(TInt aDocPos) const;
Returns the rectangle enclosing the paragraph containing aDocPos. If the paragraph is not formatted, returns an empty rectangle. If the paragraph is partially formatted, returns the rectangle enclosing the formatted part.
|
|
IMPORT_C TBool CalculateHorizontalExtremesL(TInt &aLeftX, TInt &aRightX, TBool aOnlyVisibleLines, TBool aIgnoreWrapCharacters=0)
const;
Returns the left and right extremes, in layout coordinates, of the formatted text.
|
|
IMPORT_C void GetCharacterHeightAndAscentL(TInt aDocPos, TInt &aHeight, TInt &aAscent) const;
Gets the height (ascent + descent) and ascent of the font of the character at aDocPos, as created using the graphics device
map used for drawing (the "image device") and returns them in aHeight and aAscent, after increasing aHeight by the font height
increase factor (see SetFontHeightIncreaseFactor()
).
|
IMPORT_C void GetFontHeightAndAscentL(const TFontSpec &aFontSpec, TInt &aHeight, TInt &aAscent) const;
Gets the height (ascent + descent) and ascent of the font specified by aFontSpec, as created using the graphics device map
used for drawing (the "image device") and puts them into aHeight and aAscent, after increasing aHeight by the font height
increase factor (see SetFontHeightIncreaseFactor()
).
|
IMPORT_C TInt XyPosToDocPosL(TPoint &aPos, TUint aFlags=0) const;
Returns the document position of the nearest character edge to aPos. Sets aPos to the actual position of the intersection of the line's baseline with the character's edge. If aPos is before the start of the formatted area, returns the first formatted character; if it is after the end of the formatted area, returns the position after the last formatted character, or the end of the document, whichever is less.
This function is deprecated in v7.0s. Use the more powerful FindXYPos() instead.
|
|
IMPORT_C TBool DocPosToXyPosL(TInt aDocPos, TPoint &aPos, TUint aFlags=0) const;
Returns the x-y coordinates of the document position aDocPos in aPos. The return value is ETrue if the position is formatted, or EFalse if it is not, in which case aPos is undefined.
Deprecated - use the more powerful FindDocPos()
instead
|
|
IMPORT_C TBool FindXyPos(const TPoint &aXyPos, TTmPosInfo2 &aPosInfo, TTmLineInfo *aLineInfo=0) const;
Finds the document position nearest to aXyPos. If aXyPos is in the formatted text returns ETrue, otherwise returns EFalse. If ETrue is returned, places information about the document position in aPosInfo and information about the line containing the document position in aLineInfo if it is non-NULL.
|
|
IMPORT_C TBool FindDocPos(const TTmDocPosSpec &aDocPos, TTmPosInfo2 &aPosInfo, TTmLineInfo *aLineInfo=0) const;
Finds the x-y position of the document position aDocPos.
If ETrue is returned, places information about the document position in aPosInfo and information about the line containing the document position in aLineInfo if it is non-NULL.
|
|
IMPORT_C TRect GetLineRectL(TInt aDocPos1, TInt aDocPos2) const;
Gets a rectangle enclosing two formatted document positions on the same line. If the second position is less than the first, or on a different line, it is taken to indicate the end of the line. This function panics if either position is unformatted.
Note:
CTextLayout must have been set with a valid wrap width and band height before calling this function, otherwise no formatting will take place and the function will panic. Wrap width and band height values must be > 0 to be valid.
|
|
IMPORT_C TBool PictureRectangleL(TInt aDocPos, TRect &aPictureRect, TBool *aCanScaleOrCrop=0) const;
Gets the bounding rectangle of the picture, if any, located at the document position or coordinates specified, and returns it in aPictureRect.
If aCanScaleOrCrop is non-null, sets aCanScaleOrCrop to indicate whether the picture can be scaled or cropped. Returns ETrue if the operation was successful. Returns EFalse otherwise; that is, if there is no picture at the position, or if the position is unformatted.
|
|
IMPORT_C TBool PictureRectangleL(const TPoint &aXyPos, TRect &aPictureRect, TBool *aCanScaleOrCrop=0) const;
Gets the bounding rectangle of the picture (if any) at aXyPos and puts it in aPictureRect. If aCanScaleOrCrop is non-null sets *aCanScaleOrCrop to indicate whether the picture can be scaled or cropped. Note that aXyPos may be outside aPictureRect on a successful return, if the picture does not occupy the whole of the section of the line it is in.
|
|
IMPORT_C TInt FirstDocPosFullyInBand() const;
Gets the first document position in a line that starts at or below the top of the visible area. If there is no such line, returns the position after the last formatted character.
|
IMPORT_C void GetMinimumSizeL(TInt aWrapWidth, TSize &aSize);
Gets the width and height of the bounding box of the text, including indents and margins, when formatted to the specified wrap width.
This is useful for applications like a web browser that need to determine the minimum width for a piece of text: if you specify zero as the wrap width, the returned aSize.iWidth contains the minimum width that could be used for the text without illegal line breaks, and if you specify KMaxTInt for aWrapWidth, the returned aSize.iHeight contains the minimum height: the height when each paragraph is a single line of unlimited length.
|
IMPORT_C void GetMinimumSizeL(TInt aWrapWidth, TBool aAllowLegalLineBreaksOnly, TSize &aSize);
Gets the width and height of the bounding box of the text, including indents and margins, when formatted to the specified wrap width.
This is useful for applications like a web browser that need to determine the minimum width for a piece of text: if you specify zero as the wrap width, the returned aSize.iWidth contains the minimum width that could be used for the text, and if you specify KMaxTInt for aWrapWidth, the returned aSize.iHeight contains the minimum height: the height when each paragraph is a single line of unlimited length. Use aAllowLegalLineBreaksOnly to set whether or not illegal line breaks should be considered when determining aSize.
|
IMPORT_C TInt MajorVersion() const;
Return the FORM major version number. This function is not generally useful. It is used in test code and was used while making a transition between this and the former version of FORM. The return value is always 2.
|
IMPORT_C TInt SetViewL(const TTmDocPos &aDocPos, TInt &aYPos, TViewYPosQualifier aYPosQualifier, TDiscard aDiscardFormat=EFViewDontDiscardFormat);
Changes the top of the visible area so that the line containing aDocPos is vertically positioned at aYPos. Which part of the
line is set to appear at aYPos (top, baseline, or bottom) is controlled by the TViewYPosQualifier
argument, which also specifies whether the visible area is to be filled and whether the line should be made fully visible
if possible.
Do not use if a CTextView
object owns this CTextLayout object.
|
|
IMPORT_C TInt SetViewL(TInt aDocPos, TInt &aYPos, TViewYPosQualifier aYPosQualifier, TDiscard aDiscardFormat=EFViewDontDiscardFormat);
This interface is deprecated, and is made available in version 7.0s solely to provide binary compatibility with Symbian OS v6.1. Developers are strongly advised not to make use of this API in new applications. In particular, use the other overload of this function if you need to distinguish between leading and trailing edge positions.
Do not use if a CTextView
object owns this CTextLayout object.
|
|
IMPORT_C void FormatBandL();
Formats enough text to fill the visible band.
Note: Do not use if a CTextView
object owns this CTextLayout object.
IMPORT_C void FormatCharRangeL(TInt aStartDocPos, TInt aEndDocPos);
Sets the formatted text to begin at the start of the paragraph including aStartPos and end at aEndPos. Moves the line containing aStartDocPos to the top of the visible area.
Notes:
This function is not generally useful; it exists for the convenience of the printing system.
Do not use if a CTextView
object owns this CTextLayout object.
|
IMPORT_C TBool FormatNextLineL(TInt &aBotPixel);
A special function to support background formatting by the higher level CTextView
class. It formats the next pending line. The return value is ETrue if there is more formatting to do. On entry, aBotPixel
contains the y coordinate of the bottom of the formatted text; this is updated by the function.
Notes:
Not generally useful.
Do not use if a CTextView
object owns this CTextLayout object.
|
|
IMPORT_C TBool FormatLineL(CParaFormat *aParaFormat, TInt &aDocPos, TInt &aHeight, TBool &aPageBreak);
Controls the height of a single line, for use by the pagination system only. Using the format supplied in aParaFormat, determines the height of the line containing aDocPos and returns it in aHeight. Changes aDocPos to the end of the line and returns ETrue if that position is not the end of the paragraph.
Notes:
Not generally useful; it exists for use by the pagination system only.
Do not use if a CTextView
object owns this CTextLayout object.
|
|
IMPORT_C TInt ScrollParagraphsL(TInt &aNumParas, TAllowDisallow aScrollBlankSpace);
aPars must not scroll the display beyond the formatted range. If aPars scrolls beyond the formatted range, this method will
leave with the error code CTextLayout::EPosNotFormatted
Scrolls the text up or down by aNumParas paragraphs, disallowing blank space at the bottom of the visible area if aScrollBlankSpace
is CTextLayout::EFDisallowScrollingBlankSpace
.
Do not use if a CTextView
object owns this CTextLayout object.
|
|
IMPORT_C TInt ScrollLinesL(TInt &aNumLines, TAllowDisallow aScrollBlankSpace=EFDisallowScrollingBlankSpace);
aLines must not scroll the display beyond the formatted range. If aLines scrolls beyond the formatted range, this method will
leave with the error code CTextLayout::EPosNotFormatted
Scrolls the text up or down by aNumLines lines, disallowing blank space at the bottom of the visible area if aScrollBlankSpace
is CTextLayout::EFDisallowScrollingBlankSpace
.
Do not use if a CTextView
object owns this CTextLayout object.
|
|
IMPORT_C TInt ChangeBandTopL(TInt &aPixels, TAllowDisallow aScrollBlankSpace=EFDisallowScrollingBlankSpace);
Scrolls the text up or down by aPixels pixels, disallowing blank space at the bottom of the visible area if aScrollBlankSpace
is CTextLayout::EFDisallowScrollingBlankSpace
.
The return value (not aPixels, as you would expect from ScrollParagraphsL()
and ScrollLinesL()
) contains the number of pixels not successfully scrolled, that is, the original value of aPixels, minus the number of pixels
actually scrolled. On return, aPixels is set to the number of pixels actually scrolled.
Do not use if a CTextView
object owns this CTextLayout object.
|
|
IMPORT_C void PageUpL(TInt &aYCursorPos, TInt &aPixelsScrolled);
Scrolls up by a page (that is the band height as set by SetBandHeight()
, or half that amount if scrolling over lines taller than this), moving the text downwards. The current desired vertical cursor
position is passed in aYCursorPos and updated to a new suggested position as near as possible to it, but within the visible
text and on a baseline.
Do not use if a CTextView
object owns this CTextLayout object.
|
IMPORT_C void PageDownL(TInt &aYCursorPos, TInt &aPixelsScrolled);
Scrolls down by a page (that is the band height as set by SetBandHeight()
, or half that amount if scrolling over lines taller than this), moving the text upwards. The current desired vertical cursor
position is passed in aYCursorPos and updated to a new suggested position as near as possible to it, but within the visible
text and on a baseline.
Do not use if a CTextView
object owns this CTextLayout object.
|
IMPORT_C TBool HandleCharEditL(TUint aType, TInt &aCursorPos, TInt &aGood, TInt &aFormattedUpTo, TInt &aFormattedFrom, TInt
&aScroll, TBool aFormatChanged);
Reformats to reflect a single character edit.
Do not use if a CTextView
object owns this CTextLayout object.
|
|
IMPORT_C void HandleBlockChangeL(TCursorSelection aSelection, TInt aOldCharsChanged, TViewRectChanges &aViewChanges, TBool
aFormatChanged);
Reformats to reflect changes to a block of text.
Do not use if a CTextView
object owns this CTextLayout object.
|
IMPORT_C void HandleAdditionalCharactersAtEndL(TInt &aFirstPixel, TInt &aLastPixel);
Reformats to reflect the addition of one or more complete paragraphs at the end of the text.
Do not use if a CTextView
object owns this CTextLayout object.
|
IMPORT_C void ReformatVerticalSpaceL();
Reformats to reflect changes to the space above and below paragraphs (CParaFormat::iSpaceBeforeInTwips
and iSpaceAfterInTwips).
Do not use if a CTextView
object owns this CTextLayout object.
IMPORT_C void AdjustVerticalAlignment(CParaFormat::TAlignment aVerticalAlignment);
Temporarily changes the vertical alignment of the text with respect to the visible height.
Notes:
Not generally useful.
Do not use if a CTextView
object owns this CTextLayout object.
|
static IMPORT_C void DrawBorders(const MGraphicsDeviceMap *aGd, CGraphicsContext &aGc, const TRect &aRect, const TParaBorderArray
&aBorder, const TRgb *aBackground=0, TRegion *aClipRegion=0,const TRect *aDrawRect=0);
Draws paragraph borders, optionally with a background colour for the border and a clip region. Provided for applications that display a menu of border styles, like a wordprocessor.
|
IMPORT_C void DrawL(const TRect &aDrawRect, const TDrawTextLayoutContext *aDrawTextLayoutContext, const TCursorSelection *aHighlight=0);
Draws the text. Draws any lines that intersect aDrawRect, which is specified in window coordinates. The drawing parameters, including the graphics context, are given in aDrawTextLayoutContext. If aHighlight is non-null, highlights (by exclusive-ORing) the specified range of text.
|
IMPORT_C TBool GetNextVisualCursorPos(const TTmDocPosSpec &aDocPos, TTmPosInfo2 &aPosInfo, TBool aToLeft) const;
Finds the next cursor position to aDocPos in the visually ordered line.
|
|
IMPORT_C void InvertRangeL(const TCursorSelection &aHighlight, const TRect &aDrawRect, const TDrawTextLayoutContext *aDrawTextLayoutContext);
Toggles the selection highlight for the range of text in aHighlight.
Highlights only those lines that intersect aDrawRect, which is specified in window coordinates. The drawing parameters, including the graphics context, are given in aDrawTextLayoutContext.
In v7.0 and onwards, this function is deprecated -Highlight() should be used instead.
|
IMPORT_C void Highlight(const TRangeChange &aHighlight, const TRect &aDrawRect, const TDrawTextLayoutContext *aDrawTextLayoutContext);
Sets or clears a highlight.
If the range of characters to highlight is of zero length, the function has no effect.
The function affects only those lines that intersect aDrawRect, which is specified in window coordinates. The drawing parameters, including the graphics context, are given in aDrawTextLayoutContext.
From v7.0, this function replaces InvertRangeL()
.
This function is not intended to be used to set any part of a highlight already set, nor to clear any piece of text not highlighted.
It is intended to do either or both of: clear an existing selection, set a new selection. See the class description for TRangeChange
for a code fragment showing how this function should be used.
|
IMPORT_C void SetCustomDraw(const MFormCustomDraw *aCustomDraw);
Sets the custom drawing object, for customising the way text and its background are drawn.
|
IMPORT_C const MFormCustomDraw *CustomDraw() const;
Returns a pointer to the current custom drawing implementation. Returns NULL if custom drawing is not in force.
|
IMPORT_C void SetCustomWrap(const MFormCustomWrap *aCustomWrap);
Sets custom line breaking.
If this function is not called, default line breaking behaviour is used.
Ownership of the custom line breaking object is not transferred to this object.
|
IMPORT_C const MFormCustomWrap *CustomWrap() const;
Gets the custom line breaking object, as set using SetCustomWrap()
.
|
IMPORT_C void ExtendFormattingToCoverPosL(TInt aDocPos);
aDocPos is in the range 0...DocumentLength
Allows you to increase the formatted text range by specifying a point in the text you want to increase the range to. Makes sure the line that aDocPos is in (if it exists) is covered by the formatting.
aDocPos has been formatted
|
IMPORT_C void SetInterfaceProvider(MFormCustomInterfaceProvider *aProvider);
This method allows Form clients to register an object able to create or return references to customisation objects used within Form for various tasks e.g. inline text.
|
inline void RestrictScrollToTopsOfLines(TBool aRestrict);
Dangerous function. Makes scroll operations set the top of the screen flush to the top of a line. In general this might scroll the cursor off the screen.
|
inline const TTmHighlightExtensions &HighlightExtensions() const;
|
IMPORT_C void SetCustomInvisibleCharacterRemapper(MFormCustomInvisibleCharacterRemapper *aInvisibleCharacterRemapper);
Allows Form clients to register an invisible character remapper object to customize the visible display of invisible characters such as paragraph marks.
|
IMPORT_C MFormCustomInvisibleCharacterRemapper *GetCustomInvisibleCharacterRemapper();
Allows Form clients to see which character remapper object is currently registered.
|
IMPORT_C void MakeVisible(TBool aVisible);
Stops or allows text to be drawn. Included to allow users to control visibility if text is part of an invisible control.
|
class TRangeChange;
Specifies the range of characters involved when setting or clearing a text selection.
This class is used in the CTextLayout::Highlight()
function. The following code demonstrates how it should be used to clear an existing highlight and set a new one:
CTextLayout::TRangeChange oldHighlight(anchorPos, old_cursorPos,
CTextLayout::TRangeChange::EClear); // existing highlight
CTextLayout::TRangeChange newHighlight(anchorPos, new_cursorPos,
CTextLayout::TRangeChange::ESet); // new one
newHighlight.OptimizeWith(oldHighlight); // doesn't matter which range is
the parameter and which is the calling object
layout.Highlight(oldHighlight,drawRect,context); // doesn't matter in which
order this and following line occur
layout.Highlight(newHighlight,drawRect,context);
Defined in CTextLayout::TRangeChange
:
Clip()
, EClear
, ESet
, Get()
, NonNull()
, OptimizeWith()
, Set()
, TChangeType
, TRangeChange()
, TRangeChange()
TRangeChange()
IMPORT_C TRangeChange(TInt aStart, TInt aEnd, TChangeType aChange);
Constructor with a start and end position and whether the highlighting in the range should be set or cleared.
The start and end positions can be specified in any order.
|
TRangeChange()
IMPORT_C TRangeChange();
Default constructor.
The start and end positions are set to zero - Set()
should be called to initialise the object.
Set()
IMPORT_C void Set(TInt aStart, TInt aEnd, TChangeType aChange);
Sets the start and end positions and whether the highlighting should be set or cleared.
Called by the non-default constructor. The start and end positions can be specified in any order.
|
Get()
IMPORT_C TChangeType Get(TInt &aStart, TInt &aEnd) const;
Gets the start and end of the range and whether the highlighting should be set or cleared.
|
|
OptimizeWith()
IMPORT_C void OptimizeWith(TRangeChange &aBuddy);
Try to cancel out sections of the ranges that overlap Merges two ranges of characters.
Two successive calls to CTextLayout::Highlight()
could cause unecessary flicker or redrawing if the arguments to each call overlap. For example, if extending a highlight
involved removing the old highlight and then drawing the new one, this would cause visible flicker. This can be eliminated
by calling this function to remove any overlap between the two ranges. If there is overlap, this range is set to the result
of the merge, and the other range (aBuddy) is set to zero.
When calling this function, it does not matter whether or not the two ranges overlap. Also it does not matter which range
is the parameter and which is the calling object. After calling OptimizeWith()
, it is guaranteed that the resulting ranges will not overlap, and they will represent the same change to the highlight as
the original two ranges.
See the code fragment in the class description for TRangeChange
for an example of how this function is used.
|
NonNull()
IMPORT_C TBool NonNull() const;
Tests whether the range is not null.
|
Clip()
IMPORT_C TBool Clip(TInt aMin, TInt aMax);
Clips the range so that its start and end positions are within the minimum and maximum positions specified.
|
|
TChangeType
TChangeType
Enumerates the possible change types.
|
TDiscard
Flags used by CTextLayout::SetViewL()
. Whether to reformat and redraw.
|
TAllowDisallow
Indicates whether blank space should scroll. Used by several CTextView
and CTextLayout scrolling functions.
|
n/a
|
n/a
|
n/a
|
TAmountFormatted
Amount to format. Used by CTextLayout::SetAmountToFormat()
.
|
TCurrentFormat
Formatting information.
|
TPanicNumber