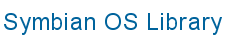
![]() |
![]() |
|
Location:
FRMTVIEW.H
Link against: form.lib
class CTextView : public CBase;
Lays out formatted text for display.
The class provides functions to:
convert between document positions and x,y coordinates
set the dimensions of the rectangle in which text can be viewed (the view rectangle)
set margin widths
do horizontal and vertical scrolling
do text selection
set the cursor position and appearance
After a change has been made to the text layout, a reformat and redraw should normally take place. CTextView provides functions
which are optimised to reformat the minimum amount necessary. For example, when a global formatting parameter is changed (e.g.
the wrap width), the whole document's layout needs to be recalculated, so HandleGlobalChangeL()
should be called. If the change involves the insertion or deletion of a single character, only a single line may be affected;
for this, HandleCharEditL()
is appropriate. Most CTextView reformatting functions do scrolling (using block transfer), if necessary, and a redraw.
For maximum responsiveness, CTextView uses an active object to carry out reformatting as a background task, so that the application can continue to receive user input. Many CTextView functions force background formatting to complete before they take effect.
When scrolling vertically, positive numbers of pixels, lines, paragraphs, pages, etc., mean that the text moves down, and vice versa. When scrolling horizontally, positive numbers of pixels mean that the text moves left and vice versa.
A text view can display up to two cursors and up to three margins. The cursors are the text cursor and the line cursor. The purpose of the line cursor is to make it easier to see which line the text cursor (or the selection extension point) is on. The three margins are the label margin (for paragraph labels), the line cursor margin (for the line cursor) and the left text margin (the gap between the edge of the page and the text). All are optional, but if present, they appear in that order, starting at the left edge of the view rectangle.
An object of class CTextLayout
is used by the text view to calculate changes to the layout. This object must be specified when constructing the text view.
It is also used to set layout attributes, including the wrap width, the height of the visible portion of the document (the
"band"), whether formatting is set to the band or to the whole document and the text object which is the source of the text
and formatting information.
The x-y pixel coordinates used by CTextView are called window coordinates. Window coordinates have their origin at the top
left corner of the view window (unlike class CTextLayout
whose coordinates have their origin at the top left corner of the area within the view rectangle in which text can appear).
As in most bitmap graphics systems, x coordinates increase rightwards and y coordinates increase downwards.
CBase
- Base class for all classes to be instantiated on the heap
CTextView
- Lays out formatted text for display
Defined in CTextView
:
AlterViewRect()
, AlteredViewRect()
, CalculateHorizontalExtremesL()
, CancelSelectionL()
, ClearSelectionL()
, DisableFlickerFreeRedraw()
, DocPosToXyPosL()
, DrawL()
, DrawL()
, EFBackgroundFormattingPriority
, EFCharacterAfter
, EFCharacterBefore
, EFCheckForHorizontalScroll
, EFFirstCharOnLine
, EFLastCharOnLine
, EFNoHorizontalScroll
, EFViewDiscardAllFormat
, EFViewDiscardAllNoRedraw
, EFViewDontDiscardFormat
, EFViewDontDiscardFullRedraw
, EnableFlickerFreeRedraw()
, EnablePictureFrameL()
, FindDocPosL()
, FindXyPosL()
, FinishBackgroundFormattingL()
, FlickerFreeRedraw()
, FormatTextL()
, GetBackwardDeletePositionL()
, GetCursorPos()
, GetForwardDeletePositionL()
, GetOrigin()
, GetPictureRectangleL()
, GetPictureRectangleL()
, HandleAdditionalCharactersAtEndL()
, HandleCharEditL()
, HandleGlobalChangeL()
, HandleGlobalChangeNoRedrawL()
, HandleInsertDeleteL()
, HandleRangeFormatChangeL()
, HorizontalScrollJump()
, IsPictureFrameSelected()
, Layout()
, LeftTextMargin()
, MObserver
, MakeVisible()
, MarginWidths()
, MatchCursorHeightL()
, MatchCursorHeightToAdjacentChar()
, MoveCursorL()
, NewL()
, ParagraphRectL()
, ScrollDisplayL()
, ScrollDisplayLinesL()
, ScrollDisplayParagraphsL()
, ScrollDisplayPixelsL()
, Selection()
, SelectionVisible()
, SetBackgroundColor()
, SetCursorExtensions()
, SetCursorFlash()
, SetCursorPlacement()
, SetCursorVisibilityL()
, SetCursorWeight()
, SetCursorWidthTypeL()
, SetCursorXorColor()
, SetDisplayContextL()
, SetDocPosL()
, SetDocPosL()
, SetDocPosL()
, SetHorizontalScrollJump()
, SetLatentXPosition()
, SetLayout()
, SetLeftTextMargin()
, SetLineCursorBitmap()
, SetMarginWidths()
, SetObserver()
, SetOpaque()
, SetParagraphFillTextOnly()
, SetPendingSelection()
, SetSelectionL()
, SetSelectionVisibilityL()
, SetTextColorOverride()
, SetViewL()
, SetViewLineAtTopL()
, SetViewRect()
, SetXyPosL()
, TBeforeAfter
, TDiscard
, TDoHorizontalScroll
, TPriorities
, TTagmaForwarder
, ViewRect()
, VisualEndOfRunL()
, XyPosToDocPosL()
, anonymous
, ~CTextView()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
static IMPORT_C CTextView *NewL(CTextLayout *aLayout, const TRect &aDisplay, CBitmapDevice *aGd, MGraphicsDeviceMap *aDeviceMap,
RWindow *aWin, RWindowGroup *aGroupWin, RWsSession *aSession);
Allocates and constructs a CTextView object.
|
|
IMPORT_C void SetDisplayContextL(CBitmapDevice *aGd, RWindow *aWin, RWindowGroup *aGroupWin, RWsSession *aSession);
Changes the window server handles which the display uses. Any of the parameters can be NULL in which case they are not changed.
|
IMPORT_C void SetLayout(CTextLayout *aLayout);
Changes the text layout object used by the text view.
|
inline const CTextLayout *Layout() const;
Returns a pointer to the text layout object used by the text view.
|
IMPORT_C void SetViewRect(const TRect &aDisplay);
Sets the rectangle in which the text is displayed.
|
IMPORT_C void SetMarginWidths(TInt aLabels, TInt aLineCursor);
Sets the label and line cursor margin widths.
Does not redraw.
|
IMPORT_C void SetHorizontalScrollJump(TInt aScrollJump);
Sets the number of pixels by which a horizontal scroll jump will cause the view to scroll. To carry out a horizontal scroll,
use ScrollDisplayL()
.
|
IMPORT_C void SetLineCursorBitmap(const CFbsBitmap *aLineCursorBitmap);
Sets the bitmap to be used as a line cursor. This function must be called before attempting to draw the line cursor. Use SetCursorVisibilityL()
to make the line cursor visible.
|
IMPORT_C void SetBackgroundColor(TRgb aColor);
Sets the background colour for the view rectangle.
Does not redraw.
|
IMPORT_C void SetTextColorOverride(const TRgb *aOverrideColor=0);
Overrides the text colour, so that when redrawn, all text has the colour specified, rather than the colour which is set in the text object. When text is in this overridden state, no highlighting is visible. To return the text colour to its original state, call this function again with an argument of NULL. This also has the effect that if selection visibility is set, any highlighting will be made visible again.
Does not do a redraw.
|
IMPORT_C void SetCursorVisibilityL(TUint aLineCursor, TUint aTextCursor);
Sets the visibility of the line and text cursors.
Notes:
A group window must have been provided before a text cursor can be drawn.
Before making the line cursor visible, a line cursor bitmap must have been provided (see SetLineCursorBitmap()
).
|
IMPORT_C void SetSelectionVisibilityL(TBool aSelectionVisible);
Sets whether the text and pictures within a selection should be highlighted. If necessary, the view is redrawn.
An example of when this function may need to be used is when a control loses or gains focus.
|
IMPORT_C TBool SelectionVisible() const;
Returns if the selection is currently visible.
In particular, the selection is invisible if the text color has been overridden.
|
IMPORT_C void EnablePictureFrameL(TBool aEnabled);
Sets whether a picture frame should be displayed when the text cursor is located at a picture character.
Note:
If a picture frame is currently displayed, and aEnabled is EFalse, a redraw takes place, so that the frame is removed.
|
IMPORT_C const TRect &ViewRect() const;
Gets the rectangle in which the text is displayed.
|
IMPORT_C void MarginWidths(TInt &aLabels, TInt &aLineCursor) const;
Gets the label and line cursor margin widths in pixels.
|
IMPORT_C TInt HorizontalScrollJump() const;
Gets the number of pixels by which a horizontal scroll jump will cause the view to scroll. On construction, the default horizontal scroll jump value is set to 20 pixels.
|
IMPORT_C TInt LeftTextMargin() const;
Gets the left text margin width in pixels.
|
IMPORT_C void SetLatentXPosition(TInt aLatentX);
Sets the pending horizontal text cursor position. This is the horizontal coordinate to which the text cursor is moved after a scroll up or down by a line or a page.
|
IMPORT_C void SetParagraphFillTextOnly(TBool aFillTextOnly);
Sets the horizontal extent of the view rectangle to fill with paragraph fill colour (CParaFormat::iFillColor
).
Does not redraw.
|
IMPORT_C void SetCursorWidthTypeL(TTextCursor::EType aType, TInt aWidth=0);
Sets the text cursor's type and weight and redraws it. If the cursor's placement is vertical (ECursorVertical), the weight is the width of the cursor. If the placement is horizontal, the weight is the height of the cursor.
|
IMPORT_C void SetCursorPlacement(TTmCursorPlacement aPlacement);
Sets the text cursor's placement (its shape and position relative to the insertion position) and redraws it.
|
IMPORT_C void SetCursorWeight(TInt aWeight);
Sets the weight of the text cursor and redraws it.
If the cursor's placement is vertical (ECursorVertical), the weight is the width of the cursor. If the placement is horizontal, the weight is the height of the cursor. Has no effect if the weight value specified is zero or less.
|
IMPORT_C void SetCursorFlash(TBool aEnabled);
Sets the flashing state of the text cursor and redraws it.
|
IMPORT_C void SetCursorXorColor(TRgb aColor);
Sets the text cursor's colour and redraws it. The cursor is drawn using an Exclusive OR draw mode which ensures that the cursor's colour is different from the background colour.
|
IMPORT_C void SetCursorExtensions(TInt aFirstExtension, TInt aSecondExtension);
|
IMPORT_C TCursorSelection Selection() const;
Gets the current selection.
|
IMPORT_C TBool IsPictureFrameSelected(TRect &aPictureFrameRect, TInt &aDocPos) const;
Tests whether there is a picture with a picture frame at the current cursor position. If there is, the aPictureFrameRect and aDocPos arguments are set to contain the picture frame rectangle and the document position of the picture, respectively.
|
|
IMPORT_C TBool GetPictureRectangleL(TInt aDocPos, TRect &aPictureRect, TBool *aCanScaleOrCrop=0) const;
Gets the bounding rectangle of the picture, if any, located at the document position or x,y coordinates specified, and returns it in aPictureRect.
If aCanScaleOrCrop is non-null, sets aCanScaleOrCrop to indicate whether the picture can be scaled or cropped. Returns true if the operation was successful. Returns false otherwise; that is, if there is no picture at the position, or if the position is unformatted.
|
|
IMPORT_C TBool GetPictureRectangleL(TPoint aXyPos, TRect &aPictureRect, TBool *aCanScaleOrCrop=0);
Returns EFalse if the specified position is not contained within a formatted picture.
|
|
IMPORT_C TBool FindXyPosL(const TPoint &aXyPos, TTmPosInfo2 &aPosInfo, TTmLineInfo *aLineInfo=0);
Gets the document position of of the nearest character edge to the window coordinates specified.
|
|
IMPORT_C TInt XyPosToDocPosL(TPoint &aPoint);
Gets the document position of of the nearest character edge to the window coordinates specified. The function first identifies the line which contains, or is nearest to, the vertical coordinate. Then it identifies the character in that line closest to the horizontal coordinate.
|
|
IMPORT_C TBool FindDocPosL(const TTmDocPosSpec &aDocPos, TTmPosInfo2 &aPosInfo, TTmLineInfo *aLineInfo=0);
Finds the x-y position of the document position aDocPos.
If aDocPos is formatted, TRUE is returned and aPosInfo returns information about the document position and aLineInfo returns information about the line containing the document position, if it is non-null.
|
|
IMPORT_C TBool DocPosToXyPosL(TInt aDocPos, TPoint &aPoint);
Gets the window coordinates of the character located at the specified document position. If the document position specified has not been formatted, the function returns a value of false.
|
|
IMPORT_C TRect ParagraphRectL(TInt aDocPos) const;
Returns the rectangle enclosing the paragraph containing aDocPos. If the paragraph is not formatted, returns an empty rectangle. If the paragraph is partially formatted, returns the rectangle enclosing the formatted part.
|
|
IMPORT_C void CalculateHorizontalExtremesL(TInt &aLeftX, TInt &aRightX, TBool aOnlyVisibleLines);
Returns the left and right extremes, in window coordinates, of the formatted text.
|
IMPORT_C void MatchCursorHeightL(const TFontSpec &aFontSpec);
Sets the height and ascent of the text cursor to the values contained in aFontSpec and redraws it.
Note:
The values set by this function are temporary. If the cursor is subsequently moved, it reverts to being based on the preceding character (unless at the start of a paragraph, in which case, it is based on the following character).
|
IMPORT_C void MatchCursorHeightToAdjacentChar(TBeforeAfter aBasedOn=EFCharacterBefore);
Sets the height and ascent of the text cursor to be the same as an adjacent character and redraws it. By default, the values are set to be based on the preceding character.
|
IMPORT_C TPoint SetSelectionL(const TCursorSelection &aSelection);
Sets a selection. The selection is highlighted unless selection visibility has been unset. Any existing selection is cancelled. If necessary, the view is scrolled and redrawn.
Any background formatting is forced to complete first.
|
|
IMPORT_C void CancelSelectionL();
Removes a selection, and redraws the affected part of the screen.
Any background formatting is forced to complete first.
IMPORT_C void ClearSelectionL();
Un-draws a selection, redraws the affected part of the screen. The cursor selection remains as before. Analogous to CancelSelectionL()
, but leaves the cursor selection untouched. This function should be called before calling CTextView::SetPendingSelection()
_and_ SetHighlightExtensions has been used to extend the size of the highlight. Any background formatting is forced to complete
first.
IMPORT_C TPoint SetDocPosL(const TTmDocPosSpec &aDocPos, TBool aDragSelectOn=EFalse);
Moves the text cursor to a new document position. The view is scrolled so that the line containing the new cursor position is at the top, or the bottom, if the scroll direction was downwards.
Any background formatting is forced to complete first.
|
|
IMPORT_C TPoint SetDocPosL(TInt aDocPos, TBool aDragSelectOn=EFalse);
Moves the text cursor to a new document position. The view is scrolled so that the line containing the new cursor position is at the top, or the bottom, if the scroll direction was downwards.
Any background formatting is forced to complete first.
|
|
IMPORT_C TPoint SetXyPosL(TPoint aPos, TBool aDragSelectOn, TRect *&aPictureRect, TInt &aPictureFrameEdges);
Moves the text cursor to the nearest point on the screen to the x,y coordinates specified.
Any background formatting is forced to complete first.
|
|
IMPORT_C TPoint MoveCursorL(TCursorPosition::TMovementType &aMovement, TBool aDragSelectOn);
Moves the text cursor in the direction and manner specified. If necessary, the view is scrolled so that the line containing the new cursor position is visible.
Any background formatting is forced to complete first.
|
|
IMPORT_C TInt ScrollDisplayL(TCursorPosition::TMovementType aMovement, CTextLayout::TAllowDisallow aScrollBlankSpace=CTextLayout::EFDisallowScrollingBlankSpace);
aMovement must not scroll the display beyond the formatted range. If aMovement scrolls beyond the formatted range, this method
will leave with the error code CTextLayout::EPosNotFormatted
Scrolls the view either horizontally or vertically and does a redraw.
Any background formatting is forced to complete first.
|
|
IMPORT_C TPoint SetViewLineAtTopL(TInt aLineNo);
Moves the view vertically and redraws, so that the top of the specified line is located flush with the top of the view rectangle.
Note:
Line numbering is relative to formatted text only and the first formatted line is numbered one.
|
|
IMPORT_C void ScrollDisplayPixelsL(TInt &aDeltaY);
Scrolls the view vertically by a number of pixels, disallowing blank space from scrolling into the bottom of the visible area. Redraws the newly visible lines.
Any background formatting is forced to complete first.
|
IMPORT_C TInt ScrollDisplayLinesL(TInt &aDeltaLines, CTextLayout::TAllowDisallow aScrollBlankSpace=CTextLayout::EFDisallowScrollingBlankSpace);
aDeltaLines must not scroll the display beyond the formatted range. If aDeltaLines scrolls beyond the formatted range, this
method will leave with the error code CTextLayout::EPosNotFormatted
Scrolls the view vertically by a number of wholly or partially visible lines, disallowing blank space at the bottom of the
visible area if aScrollBlankSpace is CTextLayout::EFDisallowScrollingBlankSpace
. Redraws the newly visible lines.
Any background formatting is forced to complete first.
|
|
IMPORT_C TInt ScrollDisplayParagraphsL(TInt &aDeltaParas, CTextLayout::TAllowDisallow aScrollBlankSpace=CTextLayout::EFDisallowScrollingBlankSpace);
aDeltaParas must not scroll the display beyond the formatted range. If aDeltaParas scrolls beyond the formatted range, this
method will leave with the error code CTextLayout::EPosNotFormatted
Scrolls the view by a number of wholly or partially visible paragraphs disallowing blank space at the bottom of the visible
area if aScrollBlankSpace is CTextLayout::EFDisallowScrollingBlankSpace
. Redraws the newly visible paragraphs.
Any background formatting is forced to complete first.
|
|
IMPORT_C TPoint SetViewL(TInt aDocPos, TInt &aYPos, TViewYPosQualifier aYPosQualifier=TViewYPosQualifier(), TDiscard aDiscardFormat=EFViewDontDiscardFormat,
TDoHorizontalScroll aDoHorizontalScroll=EFCheckForHorizontalScroll);
Sets the vertical position of the view, so that the line containing aDocPos is located as close as possible to the vertical window coordinate aYPos.
Which part of the line is set to appear at aYPos (top, baseline, or bottom) is controlled by aYPosQualifier, which also specifies whether the visible area is to be filled and whether the line should be made fully visible if possible.
|
|
IMPORT_C void SetLeftTextMargin(TInt aLeftMargin);
Sets the left text margin width in pixels.
The left text margin width is the distance between the text and the left edge of the page. Increasing the left text margin width causes text to move leftwards and decreasing the left text margin width causes text to move rightwards.
Does not redraw.
|
IMPORT_C void DrawL(TRect aRect);
Draws the text to a rectangle. Enters OOM state before leaving. RWindow::BeginRedraw()
should always be called before calling this function, for the window that the textview draws to.
|
IMPORT_C void DrawL(TRect aRect, CBitmapContext &aGc);
Draws the text to a rectangle.
Draw to a specific GC rather than the stored one. This is essential when drawing CTextView objects that are embedded inside others, as happens for editable text controls in WEB (the outermost WEB object is a CTextView, which has embedded controls that own CEikEdwins, and these own CTextView objects).
|
inline void SetObserver(MObserver *aObserver);
Sets a text view observer. This provides notification to the owner of the text view object of changes to the formatting. Its OnReformatL() function is called after reformatting but before redisplay, so that edit windows etc. can be resized.
|
IMPORT_C void SetPendingSelection(const TCursorSelection &aSelection);
Makes a selection, but without updating the view.
This function would only be used before calling CTextView::HandleRangeFormatChangeL()
or HandleInsertDeleteL()
to make a selection which will become visible (if selection visibility is on) after the change handling function has returned.
|
inline void GetOrigin(TPoint &aPoint) const;
Gets the origin of the cursor.
|
IMPORT_C TCursorSelection GetForwardDeletePositionL();
Returns a selection for which characters should be deleted in a forwards delete.
|
IMPORT_C TCursorSelection GetBackwardDeletePositionL();
Returns a selection for which characters should be deleted in a backwards delete.
|
IMPORT_C void FormatTextL();
Reformats the whole document, or just the visible text, if formatting is set to the band only (see CTextLayout::SetAmountToFormat()
).
Notes:
Reformatting the entire document may be time consuming, so only use this function if necessary.
This function does not redraw the reformatted text.
IMPORT_C TInt HandleCharEditL(TUint aType=CTextLayout::EFCharacterInsert, TBool aFormatChanged=EFalse);
Reformats and redraws the view in response to a single key press. The precise amount of text to be reformatted is controlled by the second parameter.
|
|
IMPORT_C TPoint HandleRangeFormatChangeL(TCursorSelection aSelection, TBool aFormatChanged=EFalse);
Reformats and redraws the view after changes to the character or paragraph formatting of a block of text. Reformatting can take place either from the start of the line or the start of the paragraph containing the cursor position, depending on aFormatChanged.
Can be used in combination with SetPendingSelection()
to set a selection which should remain after the function has returned.
Note:
A panic occurs if partial lines are excluded from the view (see CTextLayout::ExcludingPartialLines()
).
Any background formatting is forced to complete first.
|
|
IMPORT_C TPoint HandleInsertDeleteL(TCursorSelection aSelection, TInt aDeletedChars, TBool aFormatChanged=EFalse);
Reformats and redraws the view after inserting, deleting or replacing a block of text.
Can be used in combination with SetPendingSelection()
to set a selection which should remain after the function has returned.
Notes:
If inserting or deleting a single character, use CTextView::HandleCharEditL()
instead.
A panic occurs if partial lines are excluded from the view (see CTextLayout::ExcludingPartialLines()
).
|
|
IMPORT_C void HandleGlobalChangeL(TViewYPosQualifier aYPosQualifier=TViewYPosQualifier());
Reformats and redraws the view after a global change has been made to the layout. Examples of global layout changes include changing the format mode, the wrap width, or the visibility of nonprinting characters.
Forces any background formatting to complete.
|
IMPORT_C void HandleGlobalChangeNoRedrawL(TViewYPosQualifier aYPosQualifier=TViewYPosQualifier());
Reformats the view after a global change has been made to the layout, but without causing a redraw. Examples of global layout changes include changing the format mode, the wrap width, or the visibility of nonprinting characters.
Forces any background formatting to complete.
|
IMPORT_C void HandleAdditionalCharactersAtEndL();
Reformats and redraws to reflect the addition of one or more complete paragraphs at the end of the text. For instance, it may be used where information is being found by an active object and appended to a document.
Note:
This function should not be used to handle the situation where text is added to the final paragraph. In this case, use HandleInsertDeleteL()
or HandleCharEditL()
instead.
IMPORT_C void FinishBackgroundFormattingL();
Forces completion of background formatting.
This is called automatically by all CTextView functions which depend on the formatting being up to date.
IMPORT_C const TTmDocPos &VisualEndOfRunL(const TTmDocPos &aStart, const TTmDocPos &aEnd, TCursorPosition::TVisualEnd aDirection);
Calculates which of two document positions would be considered more left or more right, depending on the value of aDirection.
|
|
IMPORT_C void GetCursorPos(TTmDocPos &aPos) const;
Gets the cursor position as a TTmDocPos
.
|
IMPORT_C TPoint SetDocPosL(const TTmDocPos &aDocPos, TBool aDragSelectOn=EFalse);
Moves the text cursor to a new document position. The view is scrolled so that the line containing the new cursor position is at the top, or the bottom, if the scroll direction was downwards.
Any background formatting is forced to complete first.
|
|
IMPORT_C void SetOpaque(TBool aDrawOpaque);
Sets and unsets an opaque flag on the text view's window.
|
IMPORT_C void MakeVisible(TBool aVisible);
Stops or allows text to be drawn. Included to allow users to control visibility if text is part of an invisible control.
|
class MObserver;
Provides notification to the owner of the text view object of changes to the formatting.
Its OnReformatL()
function is called after reformatting but before redisplay, so that edit windows etc. can be resized.
Defined in CTextView::MObserver
:
OnReformatL()
OnReformatL()
virtual void OnReformatL(const CTextView *aTextView)=0;
Called after reformatting but before redisplay, so that edit windows, etc., can be resized.
|
class TTagmaForwarder : public MTmTextLayoutForwarder;
A standard inquiry interface for the text formatting engine, built on top of a CTextView
object.
To use it, construct a TTagmaForwarder
object, then call InitL()
, which finishes background formatting, then call the MTmTextLayoutForwarder
functions.
MTmTextLayoutForwarder
- No description.
CTextView::TTagmaForwarder
- A standard inquiry interface for the text formatting engine, built on top of a
Defined in CTextView::TTagmaForwarder
:
GetOrigin()
, InitL()
, TTagmaForwarder()
, TextLayout()
TTagmaForwarder()
inline TTagmaForwarder();
This constructor deliberately does not take a pointer or reference to CTextView
, to prevent the class from being used unless InitL()
is called.
InitL()
inline void InitL(CTextView *aView);
Called after construction, to complete background reformatting.
|
TextLayout()
private: virtual inline const CTmTextLayout &TextLayout() const;
Returns a reference to the CTmTextLayoutObject this MTmTextLayoutForwarder
forwards inquiries to.
|
GetOrigin()
private: virtual inline void GetOrigin(TPoint &aPoint) const;
The origin is subtracted from coordinates passed in and added to those passed out.
|
TBeforeAfter
Cursor height matching.
Passed as an argument to MatchCursorHeightToAdjacentChar()
.
|
TDiscard
Whether to reformat and redraw. Argument to SetViewL()
.
|