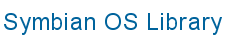
![]() |
![]() |
|
Location:
videoplayhwdevice.h
Link against: devvideo.lib
class CMMFVideoDecodeHwDevice : public CMMFVideoPlayHwDevice;
CMMFVideoDecodeHwDevice is the MSL video decoder hardware device interface. All video decoders must implement this interface.
CBase
- Base class for all classes to be instantiated on the heap
CMMFVideoHwDevice
- CMMFVideoHwDevice is a base class for all video hardware devices
CMMFVideoPlayHwDevice
- A base class for all video playback (decoder and post-processor) hardware devices
CMMFVideoDecodeHwDevice
- CMMFVideoDecodeHwDevice is the MSL video decoder hardware device interface
Defined in CMMFVideoDecodeHwDevice
:
CMMFVideoDecodeHwDevice()
, ConfigureDecoderL()
, DecodingPosition()
, GetBitstreamCounters()
, GetBufferL()
, GetBufferOptions()
, GetHeaderInformationL()
, NewL()
, NewPuAdapterL()
, NumFreeBuffers()
, PreDecoderBufferBytes()
, ReturnHeader()
, SetBufferOptionsL()
, SetHrdVbvSpec()
, SetInputFormatL()
, SetOutputDevice()
, SetProxy()
, SynchronizeDecoding()
, VideoDecoderInfoLC()
, WriteCodedDataL()
, ~CMMFVideoDecodeHwDevice()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CMMFVideoHwDevice
:
CustomInterface()
Inherited from CMMFVideoPlayHwDevice
:
AbortDirectScreenAccess()
,
CancelTimedSnapshot()
,
CommitL()
,
FreezePicture()
,
GetComplexityLevelInfo()
,
GetOutputFormatListL()
,
GetPictureCounters()
,
GetSnapshotL()
,
GetSupportedSnapshotFormatsL()
,
GetTimedSnapshotL()
,
Initialize()
,
InputEnd()
,
IsPlaying()
,
NumComplexityLevels()
,
Pause()
,
PictureBufferBytes()
,
PlaybackPosition()
,
PostProcessorInfoLC()
,
Redraw()
,
ReleaseFreeze()
,
Resume()
,
ReturnPicture()
,
Revert()
,
SetClockSource()
,
SetComplexityLevel()
,
SetInputCropOptionsL()
,
SetOutputCropOptionsL()
,
SetOutputFormatL()
,
SetPauseOnClipFail()
,
SetPosition()
,
SetPostProcSpecificOptionsL()
,
SetPostProcessTypesL()
,
SetRotateOptionsL()
,
SetScaleOptionsL()
,
SetScreenClipRegion()
,
SetVideoDestScreenL()
,
SetYuvToRgbOptionsL()
,
Start()
,
StartDirectScreenAccessL()
,
Stop()
static IMPORT_C CMMFVideoDecodeHwDevice *NewL(TUid aUid, MMMFDevVideoPlayProxy &aProxy);
Creates a new video decoder hardware device object, based on the implementation UID.
|
|
|
static IMPORT_C CMMFVideoDecodeHwDevice *NewPuAdapterL(const CImplementationInformation &aImplInfo, MMMFDevVideoPlayProxy
&aProxy);
Creates a new video decoder hardware device adapter object, based on the Implementation Information of a Processing Unit.
|
|
|
virtual CVideoDecoderInfo *VideoDecoderInfoLC()=0;
Retrieves decoder information about this hardware device. The device creates a CVideoDecoderInfo
structure, fills it with correct data, pushes it to the cleanup stack and returns it. The client will delete the object when
it is no longer needed.
|
|
virtual TVideoPictureHeader *GetHeaderInformationL(TVideoDataUnitType aDataUnitType, TVideoDataUnitEncapsulation aEncapsulation,
TVideoInputBuffer *aDataUnit)=0;
Reads header information from a coded data unit.
|
|
|
virtual void ReturnHeader(TVideoPictureHeader *aHeader)=0;
"This method can only be called before the hwdevice has been initialized with Initialize()."
Returns a header from GetHeaderInformationL()
back to the decoder so that the memory can be freed.
|
virtual void SetInputFormatL(const CCompressedVideoFormat &aFormat, TVideoDataUnitType aDataUnitType, TVideoDataUnitEncapsulation
aEncapsulation, TBool aDataInOrder)=0;
"This method can only be called before the hwdevice has been initialized with Initialize()."
Sets the device input format to a compressed video format.
|
|
virtual void SynchronizeDecoding(TBool aSynchronize)=0;
"This method can only be called before the hwdevice has been initialized with Initialize()."
Sets whether decoding should be synchronized to the current clock source, if any, or if pictures should instead be decoded as soon as possible. If decoding is synchronized, decoding timestamps are used if available, presentation timestamps are used if not. When decoding is not synchronized, pictures are decoded as soon as source data is available for them, and the decoder has a free output buffer. If a clock source is not available, decoding will not be synchronized.
|
virtual void SetBufferOptionsL(const CMMFDevVideoPlay::TBufferOptions &aOptions)=0;
"This method can only be called before the hwdevice has been initialized with Initialize()."
Sets decoder buffering options. See [3] for a description of the options available.
|
|
virtual void GetBufferOptions(CMMFDevVideoPlay::TBufferOptions &aOptions)=0;
"This method can only be called before the hwdevice has been initialized with Initialize()."
Gets the video decoder buffer options actually in use. This can be used before calling SetBufferOptions() to determine the default options, or afterwards to check the values actually in use (if some default values were used).
|
virtual void SetHrdVbvSpec(THrdVbvSpecification aHrdVbvSpec, const TDesC8 &aHrdVbvParams)=0;
"This method can only be called before the hwdevice has been initialized with Initialize()."
Indicates which HRD/VBV specification is fulfilled in the input stream and any related parameters.
|
virtual void SetOutputDevice(CMMFVideoPostProcHwDevice *aDevice)=0;
"This method can only be called before the hwdevice has been initialized with Initialize()."
Sets the output post-processor device to use. If an output device is set, all decoded pictures are delivered to that device, and not drawn on screen or returned to the client. Pictures are written using CMMDVideoPostProcDevice::WritePictureL() or a custom interface after they have been decoded. The post-processor must then synchronize rendering to the clock source if necessary.
|
virtual TTimeIntervalMicroSeconds DecodingPosition()=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Returns the current decoding position, i.e. the timestamp for the most recently decoded picture.
|
virtual TUint PreDecoderBufferBytes()=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Returns the current pre-decoder buffer size.
|
virtual void GetBitstreamCounters(CMMFDevVideoPlay::TBitstreamCounters &aCounters)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Reads various counters related to the received input bitstream and coded data units. The counters are reset when Initialize()
or this method is called, and thus they only include data processed since the last call.
|
virtual TUint NumFreeBuffers()=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Retrieves the number of free input buffers the decoder has available.
|
virtual TVideoInputBuffer *GetBufferL(TUint aBufferSize)=0;
Retrieves an empty video input buffer from the decoder. After input data has been written to the buffer, it can be written
to the decoder using WriteCodedDataL()
. The number of buffers the decoder must be able to provide before expecting any back, and the maximum size for each buffer,
are specified in the buffer options.
The decoder maintains ownership of the buffers even while they have been retrieved by the client, and will take care of deallocating them.
|
|
|
virtual void WriteCodedDataL(TVideoInputBuffer *aBuffer)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Writes a piece of coded video data to the decoder. The data buffer must be retrieved from the decoder with GetBufferL()
.
|
|
inline virtual void ConfigureDecoderL(const TVideoPictureHeader &aVideoPictureHeader);
"This method can only be called before the hwdevice has been initialized with Initialize()."
Configures the Decoder using header information known by the client.
|
|
protected: virtual void SetProxy(MMMFDevVideoPlayProxy &aProxy)=0;
Set the proxy implementation to be used. Called just after the object is constructed.
|