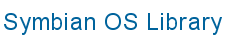
![]() |
![]() |
|
Location:
videorecordhwdevice.h
Link against: devvideo.lib
class CMMFVideoEncodeHwDevice : public CMMFVideoRecordHwDevice;
CMMFVideoEncodeHwDevice is the MSL video encoder hardware device interface. All video encoders must implement this interface.
CBase
- Base class for all classes to be instantiated on the heap
CMMFVideoHwDevice
- CMMFVideoHwDevice is a base class for all video hardware devices
CMMFVideoRecordHwDevice
- A base class for all video recording (encoding and pre-processing) hardware devices
CMMFVideoEncodeHwDevice
- CMMFVideoEncodeHwDevice is the MSL video encoder hardware device interface
Defined in CMMFVideoEncodeHwDevice
:
CMMFVideoEncodeHwDevice()
, CancelSupplementalInfo()
, CodingStandardSpecificInitOutputLC()
, CodingStandardSpecificSettingsOutputLC()
, GetOutputBufferStatus()
, ImplementationSpecificInitOutputLC()
, ImplementationSpecificSettingsOutputLC()
, NewL()
, NewPuAdapterL()
, PictureLoss()
, PictureLoss()
, ReferencePictureSelection()
, ReturnBuffer()
, SendSupplementalInfoL()
, SendSupplementalInfoL()
, SetBufferOptionsL()
, SetChannelBitErrorRate()
, SetChannelPacketLossRate()
, SetCodingStandardSpecificOptionsL()
, SetErrorProtectionLevelL()
, SetErrorProtectionLevelsL()
, SetErrorsExpected()
, SetGlobalReferenceOptions()
, SetImplementationSpecificEncoderOptionsL()
, SetInLayerScalabilityL()
, SetInputDevice()
, SetLayerPromotionPointPeriod()
, SetLayerReferenceOptions()
, SetMinRandomAccessRate()
, SetNumBitrateLayersL()
, SetOutputFormatL()
, SetOutputRectL()
, SetProxy()
, SetRateControlOptions()
, SetScalabilityLayerTypeL()
, SetSegmentTargetSize()
, SliceLoss()
, VideoEncoderInfoLC()
, ~CMMFVideoEncodeHwDevice()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CMMFVideoHwDevice
:
CustomInterface()
Inherited from CMMFVideoRecordHwDevice
:
CommitL()
,
Freeze()
,
GetFrameStabilisationOutput()
,
GetPictureCounters()
,
Initialize()
,
InputEnd()
,
NumComplexityLevels()
,
Pause()
,
PreProcessorInfoLC()
,
RecordingPosition()
,
ReleaseFreeze()
,
Resume()
,
Revert()
,
SetClockSource()
,
SetColorEnhancementOptionsL()
,
SetComplexityLevel()
,
SetCustomPreProcessOptionsL()
,
SetFrameStabilisationOptionsL()
,
SetInputCropOptionsL()
,
SetInputFormatL()
,
SetOutputCropOptionsL()
,
SetOutputPadOptionsL()
,
SetPreProcessTypesL()
,
SetRgbToYuvOptionsL()
,
SetRotateOptionsL()
,
SetScaleOptionsL()
,
SetSourceCameraL()
,
SetSourceMemoryL()
,
SetYuvToYuvOptionsL()
,
Start()
,
Stop()
,
WritePictureL()
static IMPORT_C CMMFVideoEncodeHwDevice *NewL(TUid aUid, MMMFDevVideoRecordProxy &aProxy);
Creates a new video encoder hardware device object, based on the implementation UID.
|
|
|
static IMPORT_C CMMFVideoEncodeHwDevice *NewPuAdapterL(const CImplementationInformation &aImplInfo, MMMFDevVideoRecordProxy
&aProxy);
Creates a new video encoder hardware device adapter object, based on the Interface Implementation of a Processing Unit.
|
|
|
virtual CVideoEncoderInfo *VideoEncoderInfoLC()=0;
Retrieves information about the video encoder.
|
|
virtual void SetOutputFormatL(const CCompressedVideoFormat &aFormat, TVideoDataUnitType aDataUnitType, TVideoDataUnitEncapsulation
aDataEncapsulation, TBool aSegmentationAllowed=EFalse)=0;
"This method may only be called before the hwdevice has been initialized using Initialize()."
Sets the encoder output format. The picture size depends on the input data format and possible scaling performed.
|
|
virtual void SetInputDevice(CMMFVideoPreProcHwDevice *aDevice)=0;
"This method may only be called before the hwdevice has been initialized using Initialize()."
Sets the pre-processor device that will write data to this encoder. Uncompressed pictures will be written with WritePictureL()
or through a custom interface. After pictures have been processed, they must be returned to the pre-processor using ReturnPicture().
|
virtual void SetNumBitrateLayersL(TUint aNumLayers)=0;
"This method may only be called before the hwdevice has been initialized using Initialize()."
Sets the number of bit-rate scalability layers to use. Set to 1 to disable layered scalability.
Bit-rate scalability refers to the ability of a coded stream to be decoded at different bit-rates. Scalable video is typically ordered into hierarchical layers of data. A base layer contains an individual representation of a video stream and enhancement layers contain refinement data in addition to the base layer. The quality of the decoded video stream progressively improves as enhancement layers are added to the base layer.
|
|
virtual void SetScalabilityLayerTypeL(TUint aLayer, TScalabilityType aScalabilityType)=0;
"This method may only be called before the hwdevice has been initialized using Initialize()."
Sets the scalability type for a bit-rate scalability layer.
|
|
virtual void SetGlobalReferenceOptions(TUint aMaxReferencePictures, TUint aMaxPictureOrderDelay)=0;
"This method may only be called before the hwdevice has been initialized using Initialize()."
Sets the reference picture options to be used for all scalability layers. The settings can be overridden for an individual
scalability layer by using SetLayerReferenceOptions()
.
|
virtual void SetLayerReferenceOptions(TUint aLayer, TUint aMaxReferencePictures, TUint aMaxPictureOrderDelay)=0;
"This method may only be called before the hwdevice has been initialized using Initialize()."
Sets the reference picture options to be used for a single scalability layer. These settings override those set with SetGlobalReferenceOptions()
.
|
virtual void SetBufferOptionsL(const TEncoderBufferOptions &aOptions)=0;
"This method may only be called before the hwdevice has been initialized using Initialize()."
Sets encoder buffering options.
|
|
virtual void SetOutputRectL(const TRect &aRect)=0;
"This method can be called either before or after the hwdevice has been initialized with Initialize()
. If called after initialization, the change must only be committed when CommitL()
is called."
Sets the encoder output rectangle. This rectangle specifies the part of the input and output pictures which is displayed after encoding. Many video codecs process data in 16x16 pixel units but enable specifying and coding the encoder output rectangle for image sizes that are not multiple of 16 pixels (e.g 160x120).
|
|
virtual void SetErrorsExpected(TBool aBitErrors, TBool aPacketLosses)=0;
"This method can be called either before or after the hwdevice has been initialized with Initialize()
. If called after initialization, the change must only be committed when CommitL()
is called."
Sets whether bit errors or packets losses can be expected in the video transmission. The video encoder can use this information to optimize the bitstream.
|
virtual void SetMinRandomAccessRate(TReal aRate)=0;
"This method can be called either before or after the hwdevice has been initialized with Initialize()
. If called after initialization, the change must only be committed when CommitL()
is called."
Sets the minimum frequency (in time) for instantaneous random access points in the bitstream. An instantaneous random access point is such where the decoder can achieve a full output picture immediately by decoding data starting from the random access point. The random access point frequency may be higher than signalled, if the sequence contains scene cuts which typically cause a coding of a random access point.
|
virtual void SetCodingStandardSpecificOptionsL(const TDesC8 &aOptions)=0;
"This method can be called either before or after the hwdevice has been initialized with Initialize()
. If called after initialization, the change must only be committed when CommitL()
is called."
Sets coding-standard specific encoder options.
|
|
virtual void SetImplementationSpecificEncoderOptionsL(const TDesC8 &aOptions)=0;
"This method can be called either before or after the hwdevice has been initialized with Initialize()
. If called after initialization, the change must only be committed when CommitL()
is called."
Sets implementation-specific encoder options.
|
|
virtual HBufC8 *CodingStandardSpecificInitOutputLC()=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Returns coding-standard specific initialization output from the encoder. The information can contain, for example, the MPEG-4
VOL header. This method can be called after Initialize()
has been called.
|
|
virtual HBufC8 *ImplementationSpecificInitOutputLC()=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Returns implementation-specific initialization output from the encoder. This method can be called after Initialize()
has been called.
|
|
virtual void SetErrorProtectionLevelsL(TUint aNumLevels, TBool aSeparateBuffers)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Sets the number of unequal error protection levels. By default unequal error protection is not used.
|
|
virtual void SetErrorProtectionLevelL(TUint aLevel, TUint aBitrate, TUint aStrength)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Sets up an unequal error protection level. If unequal error protection is not used, this method can be used to control settings for the whole encoded bitstream.
|
|
virtual void SetChannelPacketLossRate(TUint aLevel, TReal aLossRate, TTimeIntervalMicroSeconds32 aLossBurstLength)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Sets the expected or prevailing channel conditions for an unequal error protection level, in terms of expected packet loss rate. The video encoder can use this information to optimize the generated bitstream.
|
virtual void SetChannelBitErrorRate(TUint aLevel, TReal aErrorRate, TReal aStdDeviation)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Sets the expected or prevailing channel conditions for an unequal error protection level, in terms of expected bit error rate. The video encoder can use this information to optimize the generated bitstream.
|
virtual void SetSegmentTargetSize(TUint aLayer, TUint aSizeBytes, TUint aSizeMacroblocks)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Sets the target size of each coded video segment. The segment target size can be specified in terms of number of bytes per segment, number of macroblocks per segment, or both.
|
virtual void SetRateControlOptions(TUint aLayer, const TRateControlOptions &aOptions)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Sets the bit-rate control options for a layer. If layered bit-rate scalability is not used, the options are set for the whole bitstream.
|
virtual void SetInLayerScalabilityL(TUint aLayer, TUint aNumSteps, TInLayerScalabilityType aScalabilityType, const TArray<
TUint > &aBitrateShare, const TArray< TUint > &aPictureShare)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Sets in-layer scalability options for a layer. In-layer bit-rate scalability refers to techniques where a specific part of a single-layer coded stream can be decoded correctly without decoding the leftover part. For example, B-pictures can be used for this. By default in-layer scalability is not used.
|
|
virtual void SetLayerPromotionPointPeriod(TUint aLayer, TUint aPeriod)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Sets the period for layer promotions points for a scalability layer. A layer promotion point is a picture where it is possible to start decoding this enhancement layer if only the lower layers were decoded earlier.
|
virtual HBufC8 *CodingStandardSpecificSettingsOutputLC()=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Returns coding-standard specific settings output from the encoder. The information can contain, for example, some bitstream headers that can change based on settings modified while encoding is in progress.
|
|
virtual HBufC8 *ImplementationSpecificSettingsOutputLC()=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Returns implementation-specific settings output from the encoder. The information can contain, for example, some bitstream headers that can change based on settings modified while encoding is in progress.
|
|
virtual void SendSupplementalInfoL(const TDesC8 &aData)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Requests the encoder to sends supplemental information in the bitstream. The information data format is coding-standard dependent. Only one supplemental information send request can be active at a time. This variant encodes the information to the next possible picture.
The client must be notified after then information has been sent by calling MMMFDevVideoRecordProxy::MdvrpSupplementalInfoSent()
.
|
|
virtual void SendSupplementalInfoL(const TDesC8 &aData, const TTimeIntervalMicroSeconds &aTimestamp)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Requests the encoder to sends supplemental information in the bitstream. The information data format is coding-standard dependent. Only one supplemental information send request can be active at a time. This variant encodes the information to the picture specified.
|
|
virtual void CancelSupplementalInfo()=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Cancels the current supplemental information send request. The memory buffer reserved for supplemental information data can be reused or deallocated after the method returns.
virtual void GetOutputBufferStatus(TUint &aNumFreeBuffers, TUint &aTotalFreeBytes)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Gets the current output buffer status. The information includes the number of free output buffers and the total size of free buffers in bytes.
|
virtual void ReturnBuffer(TVideoOutputBuffer *aBuffer)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Returns a used output buffer back to the encoder. The buffer can be reused or deallocated.
|
virtual void PictureLoss()=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Indicates a picture loss to the encoder, without specifying the lost picture. The encoder can react to this by transmitting an intra-picture.
virtual void PictureLoss(const TArray< TPictureId > &aPictures)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Indicates to the encoder the pictures that have been lost. The encoder can react to this by transmitting an intra-picture.
|
virtual void SliceLoss(TUint aFirstMacroblock, TUint aNumMacroblocks, const TPictureId &aPicture)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Indicates a slice loss to the encoder.
|
virtual void ReferencePictureSelection(const TDesC8 &aSelectionData)=0;
"This method can only be called after the hwdevice has been initialized with Initialize()."
Sends a reference picture selection request to the encoder. The request is delivered as a coding-standard specific binary message. Reference picture selection can be used to select a previous correctly transmitted picture to use as a reference in case later pictures have been lost.
|
protected: virtual void SetProxy(MMMFDevVideoRecordProxy &aProxy)=0;
Set the proxy implementation to be used. Called just after the object is constructed.
|