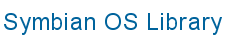
![]() |
![]() |
|
Location:
MSVFIND.H
Link against: msgs.lib
Link against: msgs_autoshutdown.lib
class CMsvFindOperation : public CMsvOperation;
Encapsulates a text search operation.
The class offers an interface for locating messages which contain specified text. Messages may contain data created for, or received from, any of the transport protocols that are supported by the Messaging Architecture (e.g. Email, FAX and SMS).
Clients create a new instance for each search operation to be performed. They are also responsible for destroying the instance once a search operation is complete.
Clients can derive from this class, typically to implement their own version of the function IsValid()
.
CBase
- Base class for all classes to be instantiated on the heap
CActive
- The core class of the active object abstraction
CMsvOperation
- Defines an interface for use by objects which control asynchronous commands in the messaging system
CMsvFindOperation
- Encapsulates a text search operation
Defined in CMsvFindOperation
:
CMsvFindOperation()
, ConstructFindInChildrenL()
, ConstructFindInSelectionL()
, DoCancel()
, FinalProgress()
, FindInChildrenL()
, FindInSelectionL()
, GetFindResult()
, IsValid()
, ProgressL()
, RunL()
, ~CMsvFindOperation()
Inherited from CActive
:
Cancel()
,
Deque()
,
EPriorityHigh
,
EPriorityIdle
,
EPriorityLow
,
EPriorityStandard
,
EPriorityUserInput
,
IsActive()
,
IsAdded()
,
Priority()
,
RunError()
,
SetActive()
,
SetPriority()
,
TPriority
,
iStatus
Inherited from CBase
:
Delete()
,
operator new()
Inherited from CMsvOperation
:
Extension_()
,
Id()
,
Mtm()
,
Service()
,
SystemProgress()
,
iMsvSession
,
iMtm
,
iObserverRequestStatus
,
iService
protected: IMPORT_C CMsvFindOperation(CMsvSession &aSession, const TDesC &aTextToFind, TMsvPartList aPartList, TRequestStatus
&aObserverRequestStatus);
Constructor, specifying search parameters.
|
|
static IMPORT_C CMsvFindOperation *FindInChildrenL(CMsvSession &aSession, const TDesC &aTextToFind, TMsvId aParentId, TMsvPartList
aPartList, TRequestStatus &aObserverRequestStatus);
Creates a new search operation to search for text within a specified root, folder or service.
Searching for messages is done recursively through all child services and folders. All messages found are searched for text.
|
|
static IMPORT_C CMsvFindOperation *FindInSelectionL(CMsvSession &aSession, const TDesC &aTextToFind, const CMsvEntrySelection
&aSelection, TMsvPartList aPartList, TRequestStatus &aObserverRequestStatus);
Creates a new search operation to search for text within a specified selection of messages.
The function leaves with the KErrArgument code if the first entry in the selection is not recognised as a message.
|
|
virtual IMPORT_C const TDesC8 &ProgressL();
Returns progress information.
Progress information supplies sufficient data to drive a progress gauge for the text search operation and also identifies the message currently being searched. It does not provide any information about the search progress within a message.
|
virtual IMPORT_C const TDesC8 &FinalProgress();
Returns progress information after the search operation is complete.
The function returns the same information as ProgressL()
but can only be called after the search operation is complete. The function raises a MSGS 285 panic if it is called while
the search operation is still in progress.
|
inline const CMsvFindResultSelection &GetFindResult() const;
Returns the result of the search operation.
If the search operation is still in progress, then the results returned are those that have been found at the time of this call.
|
protected: IMPORT_C void ConstructFindInChildrenL(TMsvId aId);
Second phase constructor, for constructing a search of an entry and of all its children.
|
protected: IMPORT_C void ConstructFindInSelectionL(const CMsvEntrySelection &aSelection);
Second phase constructor, for constructing a search of a specified selection of messages.
|
|
private: virtual IMPORT_C void DoCancel();
Implements cancellation of an outstanding request.
This function is called as part of the active object's Cancel()
.
It must call the appropriate cancel function offered by the active object's asynchronous service provider. The asynchronous service provider's cancel is expected to act immediately.
DoCancel()
must not wait for event completion; this is handled by Cancel()
.
private: virtual IMPORT_C void RunL();
Handles an active object's request completion event.
A derived class must provide an implementation to handle the completed request. If appropriate, it may issue another request.
The function is called by the active scheduler when a request completion event occurs, i.e. after the active scheduler's WaitForAnyRequest() function completes.
Before calling this active object's RunL()
function, the active scheduler has:
1. decided that this is the highest priority active object with a completed request
2. marked this active object's request as complete (i.e. the request is no longer outstanding)
RunL()
runs under a trap harness in the active scheduler. If it leaves, then the active scheduler calls RunError()
to handle the leave.
Note that once the active scheduler's Start() function has been called, all user code is run under one of the program's active
object's RunL()
or RunError()
functions.
private: virtual TBool IsValid(const TMsvEntry &aEntry) const;
Determines whether a message is to be included in the text search operation.
The function acts as a filter that decides whether a message should be included in the text search operation. The function is called before the message is searched.
The default implementation always returns true.
Clients can provide their own implementation.
Note: Messages which are not included in the search are still included in the count of entries completed.
|
|