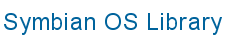
![]() |
![]() |
|
Location:
VCARD.H
Link against: vcard.lib
class CParserVCard : public CVersitParser;
A vCard parser.
Adds support for property groups (see CParserGroupedProperty
) and agents (see CParserPropertyValueAgent
) to the functionality of CVersitParser
.
Provides a constructor and overrides CVersitParser::InternalizeL()
, ExternalizeL()
, RecognizeToken()
, ConvertAllPropertyDateTimesToMachineLocalL()
, ParsePropertyL()
and MakePropertyValueL()
.
The vCard data is read from or written to a stream or file, using the InternalizeL()
and ExternalizeL()
functions. Most users of this class will only need to use these functions.
If you are sequentially creating and destroying multiple parsers, a major performance improvement may be achieved by using
thread local storage to store an instance of CVersitUnicodeUtils
which persists and can be used by all of the parsers.
See CVersitTlsData
for more information.
CBase
- Base class for all classes to be instantiated on the heap
CVersitParser
- A generic
CParserVCard
- A vCard parser
Defined in CParserVCard
:
ConvertAllPropertyDateTimesToMachineLocalL()
, ConvertDateTimesToMachineLocalAndDeleteTZL()
, ExternalizeL()
, GetGroupNamesL()
, GroupOfPropertiesL()
, InternalizeL()
, MakePropertyValueAgentL()
, MakePropertyValueL()
, MakePropertyValueSoundL()
, NewL()
, ParsePropertyL()
, RecognizeToken()
, Reserved1()
, Reserved2()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CVersitParser
:
AddEntityL()
,
AddPropertyL()
,
AdjustAllPropertyDateTimesToMachineLocalL()
,
AnalysesEncodingCharset()
,
AppendBeginL()
,
AppendEndL()
,
ArrayOfEntities()
,
ArrayOfProperties()
,
BufPtr()
,
ClearSupportsVersion()
,
ConstructL()
,
ConvertFromUnicodeToISOL()
,
ConvertToUnicodeFromISOL()
,
DecodeDateTimeL()
,
DecodePropertyValueL()
,
DecodeTimePeriodL()
,
DecodeTimeZoneL()
,
DefaultCharSet()
,
DefaultCharSetId()
,
DefaultEncoding()
,
DoAddPropertyL()
,
ECarriageReturn
,
ECharSetIdentified
,
EHTab
,
EImportSyncML
,
ELineFeed
,
ENoVersionProperty
,
ESpace
,
ESupportsVersion
,
EUseAutoDetection
,
EUseDefaultCharSetForAllProperties
,
EntityL()
,
EntityName()
,
FindFirstField()
,
FindRemainingField()
,
GetNumberL()
,
GetPropertyParamsLC()
,
IsValidLabel()
,
IsValidParameterValue()
,
LineCharSet()
,
LineCharSetId()
,
LineEncoding()
,
LineEncodingId()
,
LoadBinaryValuesFromFilesL()
,
MakeEntityL()
,
MakePropertyL()
,
MakePropertyValueCDesCArrayL()
,
MakePropertyValueDaylightL()
,
MakePropertyValueMultiDateTimeL()
,
Observer()
,
ParseBeginL()
,
ParseEndL()
,
ParseEntityL()
,
ParseParamL()
,
ParsePropertiesL()
,
PlugIn()
,
PropertyL()
,
ReadLineAndDecodeParamsLC()
,
ReadMultiLineValueL()
,
RecognizeEntityName()
,
ResetAndDestroyArrayOfDateTimes()
,
ResetAndDestroyArrayOfEntities()
,
ResetAndDestroyArrayOfParams()
,
ResetAndDestroyArrayOfProperties()
,
RestoreLineCodingDetailsToDefault()
,
SaveBinaryValuesToFilesL()
,
SetAutoDetect()
,
SetCharacterConverter()
,
SetDefaultCharSet()
,
SetDefaultCharSetId()
,
SetDefaultEncoding()
,
SetEntityNameL()
,
SetFlags()
,
SetLineCharacterSet()
,
SetLineCharacterSetId()
,
SetLineCoding()
,
SetLineEncoding()
,
SetObserver()
,
SetPlugIn()
,
SetSupportsVersion()
,
SupportsVersion()
,
TCharCodes
,
TParserCodingDetails
,
TVersitParserFlags
,
UnicodeUtils()
,
Val()
,
iArrayOfEntities
,
iArrayOfProperties
,
iAutoDetectCharSets
,
iCurrentProperty
,
iCurrentPropertyCodingDetails
,
iDecodedValue
,
iDefaultCodingDetails
,
iDefaultVersion
,
iEntityName
,
iFlags
,
iLargeDataBuf
,
iLineReader
,
iObserver
,
iOwnedLineReader
,
iPlugIn
,
iStaticUtils
,
iWriteStream
static IMPORT_C CParserVCard *NewL();
Allocates and constructs a vCard parser.
|
IMPORT_C CArrayPtr< CParserProperty > *GroupOfPropertiesL(const TDesC8 &aName) const;
Gets an array of all properties in the named property group from the vCard entity.
A property group is a collection of related properties, identified by a group name.
Ownership of the properties is not transferred.
|
|
virtual IMPORT_C void InternalizeL(RReadStream &aStream);
Internalises a vCard entity from a read stream.
The presence of this function means that the standard templated operator>>()
(defined in s32strm.h
) is available to internalise objects of this class.
|
virtual IMPORT_C void ExternalizeL(RWriteStream &aStream);
Externalises a vCard entity (and all sub-entities) to a write stream.
Sets the entity name to KVersitVarTokenVCARD if it hasn't already been set.
Adds a version property to the start of the current entity's array of properties if the entity supports this. (If there isn't an array of properties then one is made).
The presence of this function means that the standard templated operator<<()
(defined in s32strm.h
) is available to externalise objects of this class.
|
virtual IMPORT_C void ConvertAllPropertyDateTimesToMachineLocalL(const TTimeIntervalSeconds &aIncrement, const CVersitDaylight
*aDaylight);
Converts all date/time property values contained in the vCard entity into machine local values (including all date/time values contained in agent properties).
This conversion is needed because of differences in universal and local times due to time zones and daylight savings (seasonal time shifts).
If there is a daylight savings rule associated with the date/time (held in the aDaylight parameter) then this will be used to compensate for differences between universal and local times due to both time zones and the daylight savings rule. Otherwise, the aIncrement parameter is used to compensate for any difference due to time zones alone.
|
protected: virtual IMPORT_C CParserPropertyValue *MakePropertyValueAgentL(TPtr16 aValue);
|
|
protected: IMPORT_C CDesC8Array *GetGroupNamesL(TPtr8 &aGroupsAndName);
|
|
protected: virtual IMPORT_C CParserPropertyValue *MakePropertyValueL(const TUid &aPropertyUid, HBufC16 *&aValue);
Creates a property value, by parsing the specified descriptor.
This function overrides CVersitParser::MakePropertyValueL()
to ensure that agent and sound properties are correctly recognised and constructed.
Note that aValue may be set to NULL on return from this method.
|
|
protected: IMPORT_C CParserPropertyValue *MakePropertyValueSoundL(HBufC16 *&aValue);
Creates a property value object from a SOUND property value.
SOUND property values can either contain a single string (using HBufC storage) or, following the addition of Japanese pronunciation
support to Symbian's Versit
API, an array (using CDesCArray storage). This method allocates the additional storage needed to support array-based sound
properties, when necessary.
Note that aValue will be set to NULL on return from this method.
|
|
virtual IMPORT_C TUid RecognizeToken(const TDesC8 &aToken) const;
Returns a UID that identifies a specified token's type.
For example, if aToken contains the property name BDAY, the function returns KVersitPropertyDateUid. If the token is not recognized
as vCard-specific, the function calls CVersitParser::RecognizeToken()
, which recognizes generic Versit
tokens.
|
|
IMPORT_C void ConvertDateTimesToMachineLocalAndDeleteTZL();
Converts all date/time property values contained in the vCard entity into machine local values (including all date/time values contained in agent properties).
This conversion is needed because of differences in universal and local times due to time zones.
Finds the necessary increment to compensate for the time zone, using the value given by the first time zone property (named
KVersitTokenTZ) in the array of properties. This increment is passed as a parameter to ConvertAllPropertyDateTimesToMachineLocalL()
. The CVersitDaylight* parameter passed to ConvertAllPropertyDateTimesToMachineLocalL()
is NULL, so no compensation can be made by this function for any daylight saving (seasonal time shift).
private: virtual IMPORT_C void Reserved2();