iGraphics2D Struct Reference
[2D]
This is the interface for 2D renderer.
More...
#include <ivideo/graph2d.h>
Inheritance diagram for iGraphics2D:
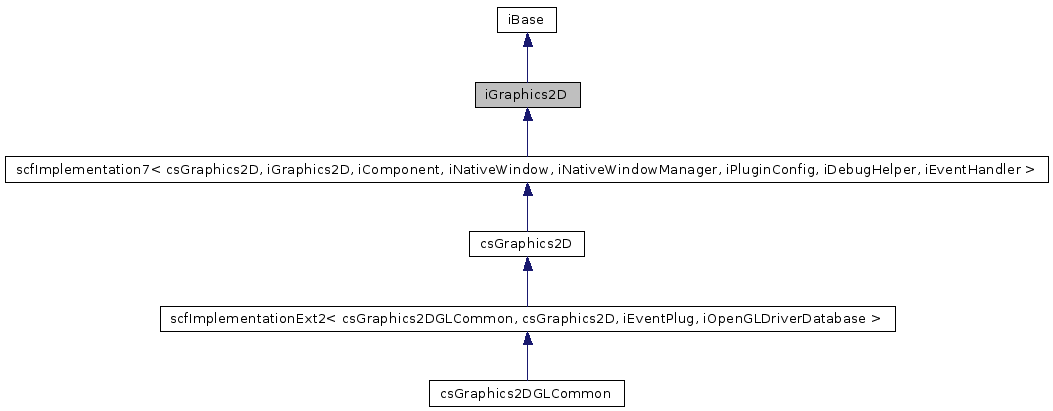
Public Member Functions | |
virtual void | AllowResize (bool iAllow)=0 |
Enable/disable canvas resizing. | |
virtual bool | BeginDraw ()=0 |
This routine should be called before any draw operations. | |
virtual void | Blit (int x, int y, int width, int height, unsigned char const *data)=0 |
Blit a memory block. Format of the image is RGBA in bytes. Row by row. | |
virtual void | Clear (int color)=0 |
Clear backbuffer. | |
virtual void | ClearAll (int color)=0 |
Clear all video pages. | |
virtual bool | ClipLine (float &x1, float &y1, float &x2, float &y2, int xmin, int ymin, int xmax, int ymax)=0 |
Clip a line against given rectangle. | |
virtual void | Close ()=0 |
Close the device. | |
virtual csPtr< iGraphics2D > | CreateOffscreenCanvas (void *memory, int width, int height, int depth, iOffscreenCanvasCallback *ofscb)=0 |
Create an off-screen canvas so you can render on a given memory area. | |
virtual bool | DoubleBuffer (bool Enable)=0 |
Enable or disable double buffering; returns success status. | |
virtual void | DrawBox (int x, int y, int w, int h, int color)=0 |
Draw a box. | |
virtual void | DrawLine (float x1, float y1, float x2, float y2, int color)=0 |
Draw a line. | |
virtual void | DrawPixel (int x, int y, int color)=0 |
Draw a pixel. | |
virtual void | DrawPixels (csPixelCoord const *pixels, int num_pixels, int color)=0 |
Draw an array of pixel coordinates with the given color. | |
virtual int | FindRGB (int r, int g, int b, int a=255)=0 |
Find an RGB (0. | |
virtual void | FinishDraw ()=0 |
This routine should be called when you finished drawing. | |
virtual void | FreeArea (csImageArea *Area)=0 |
Free storage allocated for a subarea of screen. | |
virtual void | GetClipRect (int &nMinX, int &nMinY, int &nMaxX, int &nMaxY)=0 |
Retrieve clipping rectangle. | |
virtual bool | GetDoubleBufferState ()=0 |
Get the double buffer state. | |
virtual iFontServer * | GetFontServer ()=0 |
Get the active font server (does not do IncRef()). | |
virtual bool | GetFullScreen ()=0 |
Returns 'true' if the program is being run full-screen. | |
virtual float | GetGamma () const =0 |
Get gamma value. | |
virtual int | GetHeight ()=0 |
Return the height of the framebuffer. | |
virtual const char * | GetName () const =0 |
Get the name of the canvas. | |
virtual iNativeWindow * | GetNativeWindow ()=0 |
Get the native window corresponding with this canvas. | |
virtual int | GetPage ()=0 |
Get active videopage number (starting from zero). | |
virtual int | GetPalEntryCount ()=0 |
Return the number of palette entries that can be modified. | |
virtual csRGBpixel * | GetPalette ()=0 |
Get the palette (if there is one). | |
virtual void | GetPixel (int x, int y, uint8 &oR, uint8 &oG, uint8 &oB, uint8 &oA)=0 |
As GetPixel() above, but with alpha. | |
virtual void | GetPixel (int x, int y, uint8 &oR, uint8 &oG, uint8 &oB)=0 |
Query pixel R,G,B at given screen location. | |
virtual unsigned char * | GetPixelAt (int x, int y)=0 |
Returns the address of the pixel at the specified (x, y) coordinates. | |
virtual int | GetPixelBytes ()=0 |
Return the number of bytes for every pixel. | |
virtual csPixelFormat const * | GetPixelFormat ()=0 |
Return information about the pixel format. | |
virtual void | GetRGB (int color, int &r, int &g, int &b, int &a)=0 |
Retrieve the R,G,B,A tuple for a given color index. | |
virtual void | GetRGB (int color, int &r, int &g, int &b)=0 |
Retrieve the R,G,B tuple for a given color index. | |
virtual int | GetWidth ()=0 |
Return the width of the framebuffer. | |
virtual bool | Open ()=0 |
Open the device. | |
virtual bool | PerformExtension (char const *command,...)=0 |
Perform a system specific exension. | |
virtual bool | PerformExtensionV (char const *command, va_list)=0 |
Perform a system specific exension. | |
virtual void | Print (csRect const *pArea)=0 |
Flip video pages (or dump backbuffer into framebuffer). | |
virtual bool | Resize (int w, int h)=0 |
Resize the canvas. | |
virtual void | RestoreArea (csImageArea *Area, bool Free)=0 |
Restore a subarea of screen saved with SaveArea(). | |
virtual csImageArea * | SaveArea (int x, int y, int w, int h)=0 |
Save a subarea of screen and return a handle to saved buffer. | |
virtual csPtr< iImage > | ScreenShot ()=0 |
Do a screenshot: return a new iImage object. | |
virtual void | SetClipRect (int nMinX, int nMinY, int nMaxX, int nMaxY)=0 |
Set clipping rectangle. | |
virtual void | SetFullScreen (bool b)=0 |
Change the fullscreen state of the canvas. | |
virtual bool | SetGamma (float gamma)=0 |
Set gamma value (if supported by canvas). | |
virtual bool | SetMouseCursor (iImage *image, const csRGBcolor *keycolor=0, int hotspot_x=0, int hotspot_y=0, csRGBcolor fg=csRGBcolor(255, 255, 255), csRGBcolor bg=csRGBcolor(0, 0, 0))=0 |
Set mouse cursor using an image. | |
virtual bool | SetMouseCursor (csMouseCursorID iShape)=0 |
Set mouse cursor to one of predefined shape classes (see csmcXXX enum above). | |
virtual bool | SetMousePosition (int x, int y)=0 |
Set mouse position (relative to top-left of CS window). | |
virtual void | SetRGB (int i, int r, int g, int b)=0 |
Set a color index to given R,G,B (0. | |
virtual void | Write (iFont *font, int x, int y, int fg, int bg, const wchar_t *str, uint flags=0)=0 |
Write a text string into the back buffer. | |
virtual void | Write (iFont *font, int x, int y, int fg, int bg, const char *str, uint flags=0)=0 |
Write a text string into the back buffer. | |
virtual void | WriteBaseline (iFont *font, int x, int y, int fg, int bg, const char *str)=0 |
Write a text string into the back buffer. |
Detailed Description
This is the interface for 2D renderer.The 2D renderer is responsible for all 2D operations such as creating the window, switching pages, returning pixel format and so on.
Main creators of instances implementing this interface:
- OpenGL/Windows canvas plugin (crystalspace.graphics2d.glwin32)
- OpenGL/X11 canvas plugin (crystalspace.graphics2d.glx)
- DirectDraw canvas plugin (crystalspace.graphics2d.directdraw)
- X11 canvas plugin (crystalspace.graphics2d.x2d)
- Memory canvas plugin (crystalspace.graphics2d.memory)
- Null 2D canvas plugin (crystalspace.graphics2d.null)
- Some others.
- Note that it is the 3D renderer that will automatically create the right instance of the canvas that it requires.
Main ways to get pointers to this interface:
Main users of this interface:
- 3D renderers (iGraphics3D implementations)
Definition at line 173 of file graph2d.h.
Member Function Documentation
virtual void iGraphics2D::AllowResize | ( | bool | iAllow | ) | [pure virtual] |
virtual bool iGraphics2D::BeginDraw | ( | ) | [pure virtual] |
This routine should be called before any draw operations.
It should return true if graphics context is ready.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::Blit | ( | int | x, | |
int | y, | |||
int | width, | |||
int | height, | |||
unsigned char const * | data | |||
) | [pure virtual] |
Blit a memory block. Format of the image is RGBA in bytes. Row by row.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::Clear | ( | int | color | ) | [pure virtual] |
virtual void iGraphics2D::ClearAll | ( | int | color | ) | [pure virtual] |
virtual bool iGraphics2D::ClipLine | ( | float & | x1, | |
float & | y1, | |||
float & | x2, | |||
float & | y2, | |||
int | xmin, | |||
int | ymin, | |||
int | xmax, | |||
int | ymax | |||
) | [pure virtual] |
Clip a line against given rectangle.
Function returns true if line is not visible.
Implemented in csGraphics2D.
virtual void iGraphics2D::Close | ( | ) | [pure virtual] |
virtual csPtr<iGraphics2D> iGraphics2D::CreateOffscreenCanvas | ( | void * | memory, | |
int | width, | |||
int | height, | |||
int | depth, | |||
iOffscreenCanvasCallback * | ofscb | |||
) | [pure virtual] |
Create an off-screen canvas so you can render on a given memory area.
If depth==8 then the canvas will use palette mode. In that case you can do SetRGB() to initialize the palette. The callback interface (if given) is used to communicate from the canvas back to the caller. You can use this to detect when the texture data has changed for example.
Implemented in csGraphics2D.
virtual bool iGraphics2D::DoubleBuffer | ( | bool | Enable | ) | [pure virtual] |
Enable or disable double buffering; returns success status.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::DrawBox | ( | int | x, | |
int | y, | |||
int | w, | |||
int | h, | |||
int | color | |||
) | [pure virtual] |
virtual void iGraphics2D::DrawLine | ( | float | x1, | |
float | y1, | |||
float | x2, | |||
float | y2, | |||
int | color | |||
) | [pure virtual] |
virtual void iGraphics2D::DrawPixel | ( | int | x, | |
int | y, | |||
int | color | |||
) | [pure virtual] |
virtual void iGraphics2D::DrawPixels | ( | csPixelCoord const * | pixels, | |
int | num_pixels, | |||
int | color | |||
) | [pure virtual] |
Draw an array of pixel coordinates with the given color.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual int iGraphics2D::FindRGB | ( | int | r, | |
int | g, | |||
int | b, | |||
int | a = 255 | |||
) | [pure virtual] |
Find an RGB (0.
.255) color. If there is a palette, this returns an entry index set with SetRGB(). If the returned value is -1, a suitable palette entry was not found. Without a palette, the actual color bytes are returned.
Use returned value for color arguments in iGraphics2D.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::FinishDraw | ( | ) | [pure virtual] |
This routine should be called when you finished drawing.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::FreeArea | ( | csImageArea * | Area | ) | [pure virtual] |
virtual void iGraphics2D::GetClipRect | ( | int & | nMinX, | |
int & | nMinY, | |||
int & | nMaxX, | |||
int & | nMaxY | |||
) | [pure virtual] |
virtual bool iGraphics2D::GetDoubleBufferState | ( | ) | [pure virtual] |
virtual iFontServer* iGraphics2D::GetFontServer | ( | ) | [pure virtual] |
virtual bool iGraphics2D::GetFullScreen | ( | ) | [pure virtual] |
virtual float iGraphics2D::GetGamma | ( | ) | const [pure virtual] |
virtual int iGraphics2D::GetHeight | ( | ) | [pure virtual] |
virtual const char* iGraphics2D::GetName | ( | ) | const [pure virtual] |
virtual iNativeWindow* iGraphics2D::GetNativeWindow | ( | ) | [pure virtual] |
Get the native window corresponding with this canvas.
If this is an off-screen canvas then this will return 0.
Implemented in csGraphics2D.
virtual int iGraphics2D::GetPage | ( | ) | [pure virtual] |
virtual int iGraphics2D::GetPalEntryCount | ( | ) | [pure virtual] |
Return the number of palette entries that can be modified.
This should return 0 if there is no palette (true color displays). This function is equivalent to the PalEntries field that you get from GetPixelFormat(). It is just a little bit easier to obtain this way.
Implemented in csGraphics2D.
virtual csRGBpixel* iGraphics2D::GetPalette | ( | ) | [pure virtual] |
virtual unsigned char* iGraphics2D::GetPixelAt | ( | int | x, | |
int | y | |||
) | [pure virtual] |
Returns the address of the pixel at the specified (x, y) coordinates.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual int iGraphics2D::GetPixelBytes | ( | ) | [pure virtual] |
Return the number of bytes for every pixel.
This function is equivalent to the PixelBytes field that you get from GetPixelFormat().
Implemented in csGraphics2D.
virtual csPixelFormat const* iGraphics2D::GetPixelFormat | ( | ) | [pure virtual] |
virtual void iGraphics2D::GetRGB | ( | int | color, | |
int & | r, | |||
int & | g, | |||
int & | b, | |||
int & | a | |||
) | [pure virtual] |
Retrieve the R,G,B,A tuple for a given color index.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::GetRGB | ( | int | color, | |
int & | r, | |||
int & | g, | |||
int & | b | |||
) | [pure virtual] |
Retrieve the R,G,B tuple for a given color index.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual int iGraphics2D::GetWidth | ( | ) | [pure virtual] |
virtual bool iGraphics2D::Open | ( | ) | [pure virtual] |
virtual bool iGraphics2D::PerformExtension | ( | char const * | command, | |
... | ||||
) | [pure virtual] |
Perform a system specific exension.
The command is a string; any arguments may follow. There is no way to guarantee the uniquiness of commands, so please try to use descriptive command names rather than "a", "b" and so on...
Implemented in csGraphics2D.
virtual bool iGraphics2D::PerformExtensionV | ( | char const * | command, | |
va_list | ||||
) | [pure virtual] |
Perform a system specific exension.
Just like PerformExtension() except that the command arguments are passed as a `va_list'.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::Print | ( | csRect const * | pArea | ) | [pure virtual] |
Flip video pages (or dump backbuffer into framebuffer).
The area parameter is only a hint to the canvas driver. Changes outside the rectangle may or may not be printed as well.
Implemented in csGraphics2D.
virtual bool iGraphics2D::Resize | ( | int | w, | |
int | h | |||
) | [pure virtual] |
virtual void iGraphics2D::RestoreArea | ( | csImageArea * | Area, | |
bool | Free | |||
) | [pure virtual] |
Restore a subarea of screen saved with SaveArea().
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual csImageArea* iGraphics2D::SaveArea | ( | int | x, | |
int | y, | |||
int | w, | |||
int | h | |||
) | [pure virtual] |
Save a subarea of screen and return a handle to saved buffer.
Storage is allocated in this call, you should either FreeArea() the handle after usage or RestoreArea () it.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::SetClipRect | ( | int | nMinX, | |
int | nMinY, | |||
int | nMaxX, | |||
int | nMaxY | |||
) | [pure virtual] |
Set clipping rectangle.
The clipping rectangle is inclusive the top and left edges and exclusive for the right and bottom borders.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::SetFullScreen | ( | bool | b | ) | [pure virtual] |
virtual bool iGraphics2D::SetGamma | ( | float | gamma | ) | [pure virtual] |
Set gamma value (if supported by canvas).
By default this is 1. Smaller values are darker. If the canvas doesn't support gamma then this function will return false.
Implemented in csGraphics2D.
virtual bool iGraphics2D::SetMouseCursor | ( | iImage * | image, | |
const csRGBcolor * | keycolor = 0 , |
|||
int | hotspot_x = 0 , |
|||
int | hotspot_y = 0 , |
|||
csRGBcolor | fg = csRGBcolor(255, 255, 255) , |
|||
csRGBcolor | bg = csRGBcolor(0, 0, 0) | |||
) | [pure virtual] |
Set mouse cursor using an image.
If the operation is unsupported, return 'false' otherwise return 'true'. On some platforms there is only monochrome pointers available. In this all black colors in the image will become the value of 'bg' and all non-black colors will become 'fg'
Implemented in csGraphics2D.
virtual bool iGraphics2D::SetMouseCursor | ( | csMouseCursorID | iShape | ) | [pure virtual] |
Set mouse cursor to one of predefined shape classes (see csmcXXX enum above).
If a specific mouse cursor shape is not supported, return 'false'; otherwise return 'true'. If system supports it the cursor should be set to its nearest system equivalent depending on iShape argument and the routine should return "true".
Implemented in csGraphics2D.
virtual bool iGraphics2D::SetMousePosition | ( | int | x, | |
int | y | |||
) | [pure virtual] |
virtual void iGraphics2D::SetRGB | ( | int | i, | |
int | r, | |||
int | g, | |||
int | b | |||
) | [pure virtual] |
Set a color index to given R,G,B (0.
.255) values. Only use if there is a palette.
Implemented in csGraphics2D, and csGraphics2DGLCommon.
virtual void iGraphics2D::Write | ( | iFont * | font, | |
int | x, | |||
int | y, | |||
int | fg, | |||
int | bg, | |||
const wchar_t * | str, | |||
uint | flags = 0 | |||
) | [pure virtual] |
Write a text string into the back buffer.
A value of -1 for bg
color will not draw the background.
- Remarks:
- For transparent backgrounds, it is recommended to obtain a color value from FindRGB() that has the same R, G, B components as the foreground color, but an alpha component of 0.
Implemented in csGraphics2D.
virtual void iGraphics2D::Write | ( | iFont * | font, | |
int | x, | |||
int | y, | |||
int | fg, | |||
int | bg, | |||
const char * | str, | |||
uint | flags = 0 | |||
) | [pure virtual] |
Write a text string into the back buffer.
A value of -1 for bg
color will not draw the background.
- Remarks:
str
is expected to be UTF-8 encoded.For transparent backgrounds, it is recommended to obtain a color value from FindRGB() that has the same R, G, B components as the foreground color, but an alpha component of 0.
Implemented in csGraphics2D.
virtual void iGraphics2D::WriteBaseline | ( | iFont * | font, | |
int | x, | |||
int | y, | |||
int | fg, | |||
int | bg, | |||
const char * | str | |||
) | [pure virtual] |
Write a text string into the back buffer.
A value of -1 for bg
color will not draw the background. x and y are the pen position on a baseline. The actual font baseline is shifted up by the font's descent.
- Deprecated:
- Instead, use Write() with the CS_WRITE_BASELINE flag set.
Implemented in csGraphics2D.
The documentation for this struct was generated from the following file:
- ivideo/graph2d.h
Generated for Crystal Space by doxygen 1.4.7