csGraphics2D Class Reference
[Common Plugin Classes]
This is the base class for 2D canvases.
More...
#include <csplugincommon/canvas/graph2d.h>
Inheritance diagram for csGraphics2D:
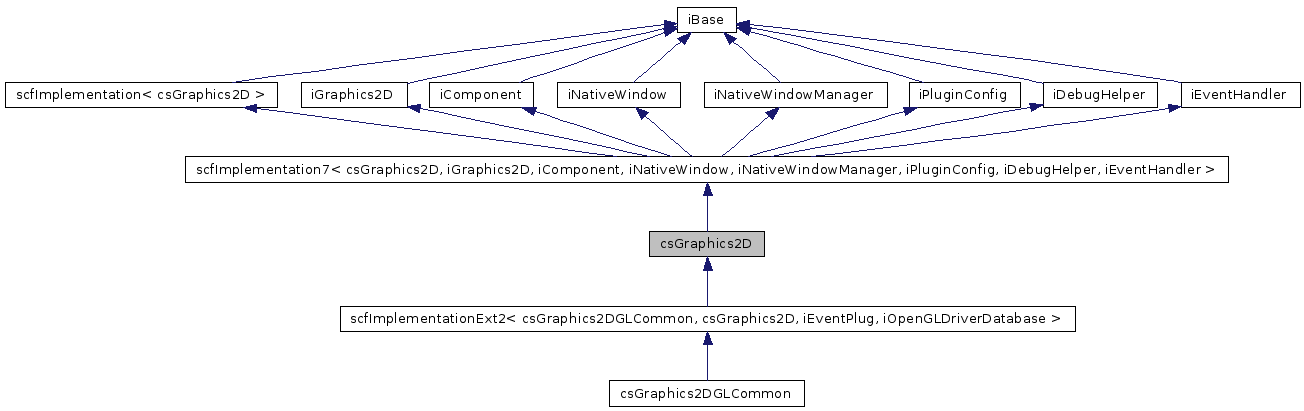
Public Member Functions | |
virtual void | AllowResize (bool) |
Enable/disable canvas resize (Over-ride in sub classes). | |
virtual bool | BeginDraw () |
This routine should be called before any draw operations. | |
virtual void | Blit (int x, int y, int width, int height, unsigned char const *data) |
Blit a memory block. Format of the image is RGBA in bytes. Row by row. | |
virtual void | ChangeDepth (int d) |
Change the depth of the canvas. | |
virtual void | Clear (int color) |
Clear backbuffer. | |
virtual void | ClearAll (int color) |
Clear all video pages. | |
virtual bool | ClipLine (float &x1, float &y1, float &x2, float &y2, int xmin, int ymin, int xmax, int ymax) |
Clip a line against given rectangle Function returns true if line is not visible. | |
virtual void | Close () |
(*) Close graphics system | |
virtual csPtr< iGraphics2D > | CreateOffscreenCanvas (void *memory, int width, int height, int depth, iOffscreenCanvasCallback *ofscb) |
Create an off-screen canvas so you can render on a given memory area. | |
csGraphics2D (iBase *) | |
Create csGraphics2D object. | |
virtual bool | DoubleBuffer (bool Enable) |
Enable or disable double buffering; return TRUE if supported. | |
virtual void | DrawBox (int x, int y, int w, int h, int color) |
Draw a box of given width and height. | |
virtual void | DrawLine (float x1, float y1, float x2, float y2, int color) |
Draw a line. | |
virtual void | DrawPixel (int x, int y, int color) |
Same but exposed through iGraphics2D interface. | |
virtual void | DrawPixels (csPixelCoord const *pixels, int num_pixels, int color) |
Draw an array of pixel coordinates with the given color. | |
virtual int | FindRGB (int r, int g, int b, int a=255) |
Find an RGB (0. | |
virtual void | FinishDraw () |
This routine should be called when you finished drawing. | |
virtual void | FreeArea (csImageArea *Area) |
Free storage allocated for a subarea of screen. | |
virtual void | GetClipRect (int &xmin, int &ymin, int &xmax, int &ymax) |
Query clipping rectangle. | |
virtual bool | GetDoubleBufferState () |
Return current double buffering state. | |
virtual iFontServer * | GetFontServer () |
Gets the font server. | |
virtual bool | GetFullScreen () |
Returns 'true' if the program is being run full-screen. | |
virtual float | GetGamma () const |
Get gamma value. | |
virtual int | GetHeight () |
Return the height of the framebuffer. | |
virtual const char * | GetName () const |
Get the name of this canvas. | |
virtual iNativeWindow * | GetNativeWindow () |
Return the Native Window interface for this canvas (if it has one). | |
virtual int | GetPage () |
Get active videopage number (starting from zero). | |
virtual int | GetPalEntryCount () |
Return the number of palette entries that can be modified. | |
virtual csRGBpixel * | GetPalette () |
Get the palette (if there is one). | |
virtual void | GetPixel (int x, int y, uint8 &oR, uint8 &oG, uint8 &oB, uint8 &oA) |
As GetPixel() above, but with alpha. | |
virtual void | GetPixel (int x, int y, uint8 &oR, uint8 &oG, uint8 &oB) |
Query pixel R,G,B at given screen location. | |
virtual unsigned char * | GetPixelAt (int x, int y) |
Same but exposed through iGraphics2D interface. | |
virtual int | GetPixelBytes () |
Return the number of bytes for every pixel. | |
virtual csPixelFormat const * | GetPixelFormat () |
Return information about about the pixel format. | |
virtual void | GetRGB (int color, int &r, int &g, int &b, int &a) |
Retrieve the R,G,B,A tuple for a given color index. | |
virtual void | GetRGB (int color, int &r, int &g, int &b) |
Retrieve the R,G,B tuple for a given color index. | |
virtual int | GetWidth () |
Return the width of the framebuffer. | |
virtual bool | HandleEvent (iEvent &) |
Event handler for plugin. | |
virtual bool | Initialize (iObjectRegistry *) |
Initialize the plugin. | |
virtual bool | Open () |
(*) Open graphics system (set videomode, open window etc) | |
virtual bool | PerformExtension (char const *command,...) |
Perform a system specific extension. | |
virtual bool | PerformExtensionV (char const *command, va_list) |
Perform a system specific extension. | |
virtual void | Print (csRect const *=0) |
(*) Flip video pages (or dump backbuffer into framebuffer). | |
virtual bool | Resize (int w, int h) |
Resize the canvas. | |
virtual void | RestoreArea (csImageArea *Area, bool Free=true) |
Restore a subarea of screen saved with SaveArea(). | |
virtual csImageArea * | SaveArea (int x, int y, int w, int h) |
Save a subarea of screen area into the variable Data. | |
virtual csPtr< iImage > | ScreenShot () |
Do a screenshot: return a new iImage object. | |
virtual void | SetClipRect (int xmin, int ymin, int xmax, int ymax) |
Set clipping rectangle. | |
virtual void | SetFullScreen (bool b) |
Change the fullscreen state of the canvas. | |
virtual bool | SetGamma (float) |
Set gamma value (if supported by canvas). | |
virtual bool | SetMouseCursor (iImage *image, const csRGBcolor *keycolor=0, int hotspot_x=0, int hotspot_y=0, csRGBcolor fg=csRGBcolor(255, 255, 255), csRGBcolor bg=csRGBcolor(0, 0, 0)) |
Set mouse cursor using an image. | |
virtual bool | SetMouseCursor (csMouseCursorID iShape) |
Set mouse cursor to one of predefined shape classes (see csmcXXX enum above). | |
virtual bool | SetMousePosition (int x, int y) |
Set mouse cursor position; return success status. | |
virtual void | SetRGB (int i, int r, int g, int b) |
(*) Set a color index to given R,G,B (0..255) values | |
virtual | ~csGraphics2D () |
Destroy csGraphics2D object. | |
virtual void | Write (iFont *font, int x, int y, int fg, int bg, const wchar_t *text, uint flags=0) |
Write a text string into the back buffer. | |
virtual void | Write (iFont *font, int x, int y, int fg, int bg, const char *text, uint flags=0) |
Write a text string into the back buffer. | |
virtual void | WriteBaseline (iFont *font, int x, int y, int fg, int bg, const char *text) |
Write a text string into the back buffer. | |
Public Attributes | |
void(* | _DrawPixel )(csGraphics2D *This, int x, int y, int color) |
Draw a pixel. | |
unsigned char *(* | _GetPixelAt )(csGraphics2D *This, int x, int y) |
(*) Get address of video RAM at given x,y coordinates | |
bool | AllowResizing |
Whether to allow resizing. | |
int | ClipX1 |
The clipping rectangle. | |
int | ClipX2 |
int | ClipY1 |
int | ClipY2 |
csConfigAccess | config |
The configuration file. | |
int | Depth |
int | DisplayNumber |
Display number. | |
csFontCache * | fontCache |
The font cache. | |
csWeakRef< iFontServer > | FontServer |
The font server. | |
int | FrameBufferLocked |
The counter that is incremented inside BeginDraw and decremented in FinishDraw(). | |
bool | FullScreen |
True if visual is full-screen. | |
int | Height |
bool | is_open |
Open/Close state. | |
int * | LineAddress |
Keep a array of Y*width to avoid multiplications. | |
unsigned char * | Memory |
Most systems have a pointer to (real or pseudo) video RAM. | |
iObjectRegistry * | object_reg |
The object registry. | |
csRef< iOffscreenCanvasCallback > | ofscb |
Callback to use for informing an external agent when several canvas operations have occured. | |
csRGBpixel * | Palette |
256-color palette. | |
bool | PaletteAlloc [256] |
true if some palette entry is already allocated. | |
csPixelFormat | pfmt |
The pixel format. | |
csWeakRef< iPluginManager > | plugin_mgr |
The plugin manager. | |
int | vpHeight |
bool | vpSet |
int | vpWidth |
int | Width |
The width, height and depth of visual. | |
csString | win_title |
Pointer to a title. | |
Protected Member Functions | |
iNativeWindowManager implementation | |
virtual void | Alert (int type, const wchar_t *title, const wchar_t *okMsg, const wchar_t *msg,...) |
Show an alert. | |
virtual void | Alert (int type, const char *title, const char *okMsg, const char *msg,...) |
Show an alert. | |
virtual void | AlertV (int type, const wchar_t *title, const wchar_t *okMsg, const wchar_t *msg, va_list args) |
Show an alert. | |
virtual void | AlertV (int type, const char *title, const char *okMsg, const char *msg, va_list args) |
Show an alert. | |
iDebugHelper implementation | |
virtual csTicks | Benchmark (int) |
Perform a benchmark. | |
virtual bool | DebugCommand (const char *cmd) |
Perform a debug command as defined by the module itself. | |
virtual void | Dump (iGraphics3D *) |
Do a graphical dump of the current state of this object. | |
virtual csPtr< iString > | Dump () |
Do a text dump of the current state of this object. | |
virtual int | GetSupportedTests () const |
Return a bit field indicating what types of functions this specific unit test implementation supports. | |
virtual csPtr< iString > | StateTest () |
Perform a state test. | |
virtual csPtr< iString > | UnitTest () |
Perform a unit test. | |
iPluginConfig implementation | |
virtual bool | GetOption (int id, csVariant *value) |
Get option. | |
virtual bool | GetOptionDescription (int idx, csOptionDescription *) |
Get option description; return FALSE if there is no such option. | |
virtual bool | SetOption (int id, csVariant *value) |
Set option. | |
iNativeWindow implementation | |
virtual void | SetTitle (const wchar_t *title) |
Set the title for this window. | |
virtual void | SetTitle (const char *title) |
Set the title for this window. | |
Static Protected Member Functions | |
static void | DrawPixel16 (csGraphics2D *This, int x, int y, int color) |
Draw a pixel in 16-bit modes. | |
static void | DrawPixel32 (csGraphics2D *This, int x, int y, int color) |
Draw a pixel in 32-bit modes. | |
static void | DrawPixel8 (csGraphics2D *This, int x, int y, int color) |
Draw a pixel in 8-bit modes. | |
static unsigned char * | GetPixelAt16 (csGraphics2D *This, int x, int y) |
Return address of a 16-bit pixel. | |
static unsigned char * | GetPixelAt32 (csGraphics2D *This, int x, int y) |
Return address of a 32-bit pixel. | |
static unsigned char * | GetPixelAt8 (csGraphics2D *This, int x, int y) |
Return address of a 8-bit pixel. | |
Protected Attributes | |
csString | name |
int | refreshRate |
Screen refresh rate. | |
bool | vsync |
Activate Vsync. | |
csRef< iEventHandler > | weakEventHandler |
Detailed Description
This is the base class for 2D canvases.Plugins should derive their own class from this one and implement required (marked with an asterisk (*)) functions. Functions not marked with an asterisk are optional, but possibly slow since they are too general.
Definition at line 62 of file graph2d.h.
Constructor & Destructor Documentation
csGraphics2D::csGraphics2D | ( | iBase * | ) |
Create csGraphics2D object.
virtual csGraphics2D::~csGraphics2D | ( | ) | [virtual] |
Destroy csGraphics2D object.
Member Function Documentation
virtual void csGraphics2D::Alert | ( | int | type, | |
const wchar_t * | title, | |||
const wchar_t * | okMsg, | |||
const wchar_t * | msg, | |||
... | ||||
) | [protected, virtual] |
virtual void csGraphics2D::Alert | ( | int | type, | |
const char * | title, | |||
const char * | okMsg, | |||
const char * | msg, | |||
... | ||||
) | [protected, virtual] |
Show an alert.
Type is one of CS_ALERT_???.
- Remarks:
- All strings are expected to be UTF-8 encoded.
Implements iNativeWindowManager.
virtual void csGraphics2D::AlertV | ( | int | type, | |
const wchar_t * | title, | |||
const wchar_t * | okMsg, | |||
const wchar_t * | msg, | |||
va_list | args | |||
) | [protected, virtual] |
virtual void csGraphics2D::AlertV | ( | int | type, | |
const char * | title, | |||
const char * | okMsg, | |||
const char * | msg, | |||
va_list | args | |||
) | [protected, virtual] |
Show an alert.
Type is one of CS_ALERT_???.
- Remarks:
- All strings are expected to be UTF-8 encoded.
Implements iNativeWindowManager.
virtual void csGraphics2D::AllowResize | ( | bool | ) | [inline, virtual] |
virtual bool csGraphics2D::BeginDraw | ( | ) | [virtual] |
This routine should be called before any draw operations.
It should return true if graphics context is ready.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual csTicks csGraphics2D::Benchmark | ( | int | ) | [inline, protected, virtual] |
Perform a benchmark.
This function will return a number indicating how long the benchmark lasted in milliseconds.
Implements iDebugHelper.
virtual void csGraphics2D::Blit | ( | int | x, | |
int | y, | |||
int | width, | |||
int | height, | |||
unsigned char const * | data | |||
) | [virtual] |
Blit a memory block. Format of the image is RGBA in bytes. Row by row.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::ChangeDepth | ( | int | d | ) | [virtual] |
Change the depth of the canvas.
virtual void csGraphics2D::Clear | ( | int | color | ) | [virtual] |
virtual void csGraphics2D::ClearAll | ( | int | color | ) | [virtual] |
virtual bool csGraphics2D::ClipLine | ( | float & | x1, | |
float & | y1, | |||
float & | x2, | |||
float & | y2, | |||
int | xmin, | |||
int | ymin, | |||
int | xmax, | |||
int | ymax | |||
) | [virtual] |
Clip a line against given rectangle Function returns true if line is not visible.
Implements iGraphics2D.
virtual void csGraphics2D::Close | ( | ) | [virtual] |
virtual csPtr<iGraphics2D> csGraphics2D::CreateOffscreenCanvas | ( | void * | memory, | |
int | width, | |||
int | height, | |||
int | depth, | |||
iOffscreenCanvasCallback * | ofscb | |||
) | [virtual] |
Create an off-screen canvas so you can render on a given memory area.
If depth==8 then the canvas will use palette mode. In that case you can do SetRGB() to initialize the palette. The callback interface (if given) is used to communicate from the canvas back to the caller. You can use this to detect when the texture data has changed for example.
Implements iGraphics2D.
virtual bool csGraphics2D::DebugCommand | ( | const char * | cmd | ) | [protected, virtual] |
Perform a debug command as defined by the module itself.
Returns 'false' if the command was not recognized.
Implements iDebugHelper.
Reimplemented in csGraphics2DGLCommon.
virtual bool csGraphics2D::DoubleBuffer | ( | bool | Enable | ) | [virtual] |
Enable or disable double buffering; return TRUE if supported.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::DrawBox | ( | int | x, | |
int | y, | |||
int | w, | |||
int | h, | |||
int | color | |||
) | [virtual] |
Draw a box of given width and height.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::DrawLine | ( | float | x1, | |
float | y1, | |||
float | x2, | |||
float | y2, | |||
int | color | |||
) | [virtual] |
virtual void csGraphics2D::DrawPixel | ( | int | x, | |
int | y, | |||
int | color | |||
) | [inline, virtual] |
Same but exposed through iGraphics2D interface.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
static void csGraphics2D::DrawPixel16 | ( | csGraphics2D * | This, | |
int | x, | |||
int | y, | |||
int | color | |||
) | [static, protected] |
Draw a pixel in 16-bit modes.
static void csGraphics2D::DrawPixel32 | ( | csGraphics2D * | This, | |
int | x, | |||
int | y, | |||
int | color | |||
) | [static, protected] |
Draw a pixel in 32-bit modes.
static void csGraphics2D::DrawPixel8 | ( | csGraphics2D * | This, | |
int | x, | |||
int | y, | |||
int | color | |||
) | [static, protected] |
Draw a pixel in 8-bit modes.
virtual void csGraphics2D::DrawPixels | ( | csPixelCoord const * | pixels, | |
int | num_pixels, | |||
int | color | |||
) | [virtual] |
Draw an array of pixel coordinates with the given color.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::Dump | ( | iGraphics3D * | ) | [inline, protected, virtual] |
Do a text dump of the current state of this object.
Returns 0 if not supported or else a string which you should DecRef() after use.
Implements iDebugHelper.
virtual int csGraphics2D::FindRGB | ( | int | r, | |
int | g, | |||
int | b, | |||
int | a = 255 | |||
) | [inline, virtual] |
Find an RGB (0.
.255) color. If there is a palette, this returns an entry index set with SetRGB(). If the returned value is -1, a suitable palette entry was not found. Without a palette, the actual color bytes are returned.
Use returned value for color arguments in iGraphics2D.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::FinishDraw | ( | ) | [virtual] |
This routine should be called when you finished drawing.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::FreeArea | ( | csImageArea * | Area | ) | [virtual] |
virtual void csGraphics2D::GetClipRect | ( | int & | xmin, | |
int & | ymin, | |||
int & | xmax, | |||
int & | ymax | |||
) | [virtual] |
virtual bool csGraphics2D::GetDoubleBufferState | ( | ) | [virtual] |
Return current double buffering state.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual iFontServer* csGraphics2D::GetFontServer | ( | ) | [inline, virtual] |
virtual bool csGraphics2D::GetFullScreen | ( | ) | [inline, virtual] |
virtual float csGraphics2D::GetGamma | ( | ) | const [inline, virtual] |
virtual int csGraphics2D::GetHeight | ( | ) | [inline, virtual] |
virtual const char* csGraphics2D::GetName | ( | ) | const [virtual] |
virtual iNativeWindow* csGraphics2D::GetNativeWindow | ( | ) | [virtual] |
virtual bool csGraphics2D::GetOption | ( | int | id, | |
csVariant * | value | |||
) | [protected, virtual] |
virtual bool csGraphics2D::GetOptionDescription | ( | int | idx, | |
csOptionDescription * | ||||
) | [protected, virtual] |
virtual int csGraphics2D::GetPage | ( | ) | [virtual] |
virtual int csGraphics2D::GetPalEntryCount | ( | ) | [inline, virtual] |
Return the number of palette entries that can be modified.
This should return 0 if there is no palette (true color displays). This function is equivalent to the PalEntries field that you get from GetPixelFormat(). It is just a little bit easier to obtain this way.
Implements iGraphics2D.
virtual csRGBpixel* csGraphics2D::GetPalette | ( | ) | [inline, virtual] |
virtual unsigned char* csGraphics2D::GetPixelAt | ( | int | x, | |
int | y | |||
) | [inline, virtual] |
Same but exposed through iGraphics2D interface.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
Definition at line 259 of file graph2d.h.
Referenced by csG2DDrawBox< Tpixel, Tpixmixer >::DrawBox(), csG2DDrawLine< Tpixel, Tpixmixer >::DrawLine(), and csG2DDrawText< Tpixel, Tpixmixer1, Tpixmixer2, Tpixmixer3 >::DrawText().
static unsigned char* csGraphics2D::GetPixelAt16 | ( | csGraphics2D * | This, | |
int | x, | |||
int | y | |||
) | [static, protected] |
Return address of a 16-bit pixel.
static unsigned char* csGraphics2D::GetPixelAt32 | ( | csGraphics2D * | This, | |
int | x, | |||
int | y | |||
) | [static, protected] |
Return address of a 32-bit pixel.
static unsigned char* csGraphics2D::GetPixelAt8 | ( | csGraphics2D * | This, | |
int | x, | |||
int | y | |||
) | [static, protected] |
Return address of a 8-bit pixel.
virtual int csGraphics2D::GetPixelBytes | ( | ) | [inline, virtual] |
Return the number of bytes for every pixel.
This function is equivalent to the PixelBytes field that you get from GetPixelFormat().
Implements iGraphics2D.
virtual csPixelFormat const* csGraphics2D::GetPixelFormat | ( | ) | [inline, virtual] |
virtual void csGraphics2D::GetRGB | ( | int | color, | |
int & | r, | |||
int & | g, | |||
int & | b, | |||
int & | a | |||
) | [virtual] |
Retrieve the R,G,B,A tuple for a given color index.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::GetRGB | ( | int | color, | |
int & | r, | |||
int & | g, | |||
int & | b | |||
) | [virtual] |
Retrieve the R,G,B tuple for a given color index.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual int csGraphics2D::GetSupportedTests | ( | ) | const [inline, protected, virtual] |
Return a bit field indicating what types of functions this specific unit test implementation supports.
This will return a combination of the CS_DBGHELP_... flags:
Implements iDebugHelper.
virtual int csGraphics2D::GetWidth | ( | ) | [inline, virtual] |
virtual bool csGraphics2D::HandleEvent | ( | iEvent & | ) | [virtual] |
virtual bool csGraphics2D::Initialize | ( | iObjectRegistry * | ) | [virtual] |
virtual bool csGraphics2D::Open | ( | ) | [virtual] |
(*) Open graphics system (set videomode, open window etc)
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual bool csGraphics2D::PerformExtension | ( | char const * | command, | |
... | ||||
) | [virtual] |
Perform a system specific extension.
Return false if extension not supported.
Implements iGraphics2D.
virtual bool csGraphics2D::PerformExtensionV | ( | char const * | command, | |
va_list | ||||
) | [virtual] |
Perform a system specific extension.
Return false if extension not supported.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::Print | ( | csRect const * | = 0 |
) | [inline, virtual] |
virtual bool csGraphics2D::Resize | ( | int | w, | |
int | h | |||
) | [virtual] |
virtual void csGraphics2D::RestoreArea | ( | csImageArea * | Area, | |
bool | Free = true | |||
) | [virtual] |
Restore a subarea of screen saved with SaveArea().
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual csImageArea* csGraphics2D::SaveArea | ( | int | x, | |
int | y, | |||
int | w, | |||
int | h | |||
) | [virtual] |
Save a subarea of screen area into the variable Data.
Storage is allocated in this call, you should either FreeArea() it after usage or RestoreArea() it.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
Do a screenshot: return a new iImage object.
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::SetClipRect | ( | int | xmin, | |
int | ymin, | |||
int | xmax, | |||
int | ymax | |||
) | [virtual] |
virtual void csGraphics2D::SetFullScreen | ( | bool | b | ) | [virtual] |
virtual bool csGraphics2D::SetGamma | ( | float | ) | [inline, virtual] |
Set gamma value (if supported by canvas).
By default this is 1. Smaller values are darker. If the canvas doesn't support gamma then this function will return false.
Implements iGraphics2D.
virtual bool csGraphics2D::SetMouseCursor | ( | iImage * | image, | |
const csRGBcolor * | keycolor = 0 , |
|||
int | hotspot_x = 0 , |
|||
int | hotspot_y = 0 , |
|||
csRGBcolor | fg = csRGBcolor(255, 255, 255) , |
|||
csRGBcolor | bg = csRGBcolor(0, 0, 0) | |||
) | [virtual] |
Set mouse cursor using an image.
If the operation is unsupported, return 'false' otherwise return 'true'. On some platforms there is only monochrome pointers available. In this all black colors in the image will become the value of 'bg' and all non-black colors will become 'fg'
Implements iGraphics2D.
virtual bool csGraphics2D::SetMouseCursor | ( | csMouseCursorID | iShape | ) | [virtual] |
Set mouse cursor to one of predefined shape classes (see csmcXXX enum above).
If a specific mouse cursor shape is not supported, return 'false'; otherwise return 'true'. If system supports it and iBitmap != 0, shape should be set to the bitmap passed as second argument; otherwise cursor should be set to its nearest system equivalent depending on iShape argument.
Implements iGraphics2D.
virtual bool csGraphics2D::SetMousePosition | ( | int | x, | |
int | y | |||
) | [virtual] |
virtual bool csGraphics2D::SetOption | ( | int | id, | |
csVariant * | value | |||
) | [protected, virtual] |
virtual void csGraphics2D::SetRGB | ( | int | i, | |
int | r, | |||
int | g, | |||
int | b | |||
) | [virtual] |
(*) Set a color index to given R,G,B (0..255) values
Implements iGraphics2D.
Reimplemented in csGraphics2DGLCommon.
virtual void csGraphics2D::SetTitle | ( | const wchar_t * | title | ) | [inline, protected, virtual] |
virtual void csGraphics2D::SetTitle | ( | const char * | title | ) | [protected, virtual] |
Set the title for this window.
- Remarks:
title
is expected to be UTF-8 encoded.
Implements iNativeWindow.
Perform a state test.
This function will test if the current state of the object is ok. It will return 0 if it is ok. Otherwise an iString is returned containing some information about the errors. DecRef() this returned string after using it.
Implements iDebugHelper.
Perform a unit test.
This function will try to test as much as possible of the given module. This function returns 0 if the test succeeded. Otherwise an iString is returned containing some information about the errors. DecRef() this returned string after using it.
Implements iDebugHelper.
virtual void csGraphics2D::Write | ( | iFont * | font, | |
int | x, | |||
int | y, | |||
int | fg, | |||
int | bg, | |||
const wchar_t * | text, | |||
uint | flags = 0 | |||
) | [virtual] |
Write a text string into the back buffer.
A value of -1 for bg
color will not draw the background.
- Remarks:
- For transparent backgrounds, it is recommended to obtain a color value from FindRGB() that has the same R, G, B components as the foreground color, but an alpha component of 0.
Implements iGraphics2D.
virtual void csGraphics2D::WriteBaseline | ( | iFont * | font, | |
int | x, | |||
int | y, | |||
int | fg, | |||
int | bg, | |||
const char * | text | |||
) | [virtual] |
Write a text string into the back buffer.
A value of -1 for bg
color will not draw the background. x and y are the pen position on a baseline. The actual font baseline is shifted up by the font's descent.
- Deprecated:
- Instead, use Write() with the CS_WRITE_BASELINE flag set.
Implements iGraphics2D.
Member Data Documentation
void(* csGraphics2D::_DrawPixel)(csGraphics2D *This, int x, int y, int color) |
Draw a pixel.
This allows deciding at run-time which function we will choose.
unsigned char*(* csGraphics2D::_GetPixelAt)(csGraphics2D *This, int x, int y) |
(*) Get address of video RAM at given x,y coordinates
The counter that is incremented inside BeginDraw and decremented in FinishDraw().
Keep a array of Y*width to avoid multiplications.
Definition at line 84 of file graph2d.h.
Referenced by csG2DDrawLine< Tpixel, Tpixmixer >::DrawLine().
unsigned char* csGraphics2D::Memory |
Most systems have a pointer to (real or pseudo) video RAM.
Definition at line 78 of file graph2d.h.
Referenced by csG2DDrawLine< Tpixel, Tpixmixer >::DrawLine().
bool csGraphics2D::PaletteAlloc[256] |
The pixel format.
Definition at line 75 of file graph2d.h.
Referenced by csPixMixerRGBA< Tpixel >::csPixMixerRGBA().
int csGraphics2D::refreshRate [protected] |
bool csGraphics2D::vsync [protected] |
The documentation for this class was generated from the following file:
- csplugincommon/canvas/graph2d.h
Generated for Crystal Space by doxygen 1.4.7