csShaderVariable Class Reference
[3D]
Storage class for "shader vars", inheritable variables in the shader system.
More...
#include <csgfx/shadervar.h>
Inheritance diagram for csShaderVariable:
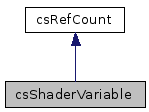
Public Types | |
ARRAY | |
Array. | |
COLOR | |
Color. | |
FLOAT | |
Float. | |
INT = 1 | |
Integer. | |
MATRIX | |
Matrix. | |
RENDERBUFFER | |
Renderbuffer. | |
TEXTURE | |
Texture. | |
TRANSFORM | |
Transform. | |
UNKNOWN = 0 | |
No value was yet set, hence the type is unknown. | |
enum | VariableType { UNKNOWN = 0, INT = 1, FLOAT, COLOR, TEXTURE, RENDERBUFFER, VECTOR2, VECTOR3, VECTOR4, MATRIX, TRANSFORM, ARRAY } |
Data types that can be stored. More... | |
VECTOR2 | |
Vector with 2 components. | |
VECTOR3 | |
Vector with 3 components. | |
VECTOR4 | |
Vector with 4 components. | |
Public Member Functions | |
void | AddVariableToArray (csShaderVariable *variable) |
csShaderVariable (const csShaderVariable &other) | |
csShaderVariable (csStringID name) | |
Construct with name. | |
csShaderVariable () | |
Construct without a name. | |
csShaderVariable * | GetArrayElement (size_t element) |
Get a specific element in an array variable Do not hold on to this for long, since it might change if the array size changes. | |
size_t | GetArraySize () |
Get the number of elements in an array variable. | |
csStringID | GetName () const |
Get the name of the variable. | |
VariableType | GetType () |
Get type of data stored. | |
bool | GetValue (csReversibleTransform &value) |
Retrieve a csReversibleTransform. | |
bool | GetValue (csMatrix3 &value) |
Retrieve a csMatrix3. | |
bool | GetValue (csVector4 &value) |
Retrieve a csVector4. | |
bool | GetValue (csColor &value) |
Retrieve a csColor. | |
bool | GetValue (csVector3 &value) |
Retrieve a csVector3. | |
bool | GetValue (csVector2 &value) |
Retrieve a csVector2. | |
bool | GetValue (iRenderBuffer *&value) |
Retrieve a iRenderBuffer. | |
bool | GetValue (iTextureWrapper *&value) |
Retrieve a texture wrapper. | |
bool | GetValue (iTextureHandle *&value) |
Retrieve a texture handle. | |
bool | GetValue (csRGBpixel &value) |
Retrieve a color. | |
bool | GetValue (float &value) |
Retrieve a float. | |
bool | GetValue (int &value) |
Retrieve an int. | |
void | RemoveFromArray (size_t element) |
void | SetAccessor (iShaderVariableAccessor *a) |
Set an accessor to use when getting the value. | |
void | SetArrayElement (size_t element, csShaderVariable *variable) |
Set a specific element in an array variable. | |
void | SetArraySize (size_t size) |
Set the number of elements in an array variable. | |
void | SetName (csStringID newName) |
Set the name of the variable. | |
void | SetType (VariableType t) |
Set type (calling this after SetValue will cause undefined behaviour). | |
bool | SetValue (const csReversibleTransform &value) |
Store a csReversibleTransform. | |
bool | SetValue (const csMatrix3 &value) |
Store a csMatrix3. | |
bool | SetValue (const csVector4 &value) |
Store a csVector4. | |
bool | SetValue (const csColor &value) |
Store a csColor. | |
bool | SetValue (const csVector3 &value) |
Store a csVector3. | |
bool | SetValue (const csVector2 &value) |
Store a csVector2. | |
bool | SetValue (iRenderBuffer *value) |
Store a render buffer. | |
bool | SetValue (iTextureWrapper *value) |
Store a texture wrapper. | |
bool | SetValue (iTextureHandle *value) |
Store a texture handle. | |
bool | SetValue (const csRGBpixel &value) |
Store a color. | |
bool | SetValue (float value) |
Store a float. | |
bool | SetValue (int value) |
Store an int. | |
virtual | ~csShaderVariable () |
Detailed Description
Storage class for "shader vars", inheritable variables in the shader system.Shader vars are a primary system to transport information from the engine/meshes/etc. to the renderer.
Definition at line 71 of file shadervar.h.
Member Enumeration Documentation
Data types that can be stored.
Data storage and retrieval is not strict - data stored as INT, FLOAT, COLOR or any VECTORx data can also be retrieved as any other of those.
- Enumerator:
Definition at line 79 of file shadervar.h.
Constructor & Destructor Documentation
csShaderVariable::csShaderVariable | ( | ) |
Construct without a name.
SetName() must be called before the variable can be used.
csShaderVariable::csShaderVariable | ( | csStringID | name | ) |
Construct with name.
Member Function Documentation
csShaderVariable* csShaderVariable::GetArrayElement | ( | size_t | element | ) | [inline] |
Get a specific element in an array variable Do not hold on to this for long, since it might change if the array size changes.
Definition at line 442 of file shadervar.h.
size_t csShaderVariable::GetArraySize | ( | ) | [inline] |
csStringID csShaderVariable::GetName | ( | ) | const [inline] |
VariableType csShaderVariable::GetType | ( | ) | [inline] |
bool csShaderVariable::GetValue | ( | csReversibleTransform & | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | csMatrix3 & | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | csVector4 & | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | csColor & | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | csVector3 & | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | csVector2 & | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | iRenderBuffer *& | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | iTextureWrapper *& | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | iTextureHandle *& | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | csRGBpixel & | value | ) | [inline] |
Retrieve a color.
Definition at line 187 of file shadervar.h.
References csRGBpixel::alpha, csRGBpixel::blue, csRGBpixel::green, and csRGBpixel::red.
bool csShaderVariable::GetValue | ( | float & | value | ) | [inline] |
bool csShaderVariable::GetValue | ( | int & | value | ) | [inline] |
Retrieve an int.
Definition at line 171 of file shadervar.h.
Referenced by csLightProperties::csLightProperties().
void csShaderVariable::SetAccessor | ( | iShaderVariableAccessor * | a | ) | [inline] |
void csShaderVariable::SetArrayElement | ( | size_t | element, | |
csShaderVariable * | variable | |||
) | [inline] |
void csShaderVariable::SetArraySize | ( | size_t | size | ) | [inline] |
void csShaderVariable::SetName | ( | csStringID | newName | ) | [inline] |
Set the name of the variable.
- Warning:
- Changing the name of a variable while it's in use can cause inexpected behaviour.
Definition at line 165 of file shadervar.h.
void csShaderVariable::SetType | ( | VariableType | t | ) | [inline] |
Set type (calling this after SetValue will cause undefined behaviour).
Definition at line 155 of file shadervar.h.
bool csShaderVariable::SetValue | ( | const csReversibleTransform & | value | ) | [inline] |
bool csShaderVariable::SetValue | ( | const csMatrix3 & | value | ) | [inline] |
bool csShaderVariable::SetValue | ( | const csVector4 & | value | ) | [inline] |
Store a csVector4.
Definition at line 370 of file shadervar.h.
References csVector4T< T >::w, csVector4T< T >::x, csVector4T< T >::y, and csVector4T< T >::z.
bool csShaderVariable::SetValue | ( | const csColor & | value | ) | [inline] |
Store a csColor.
Definition at line 361 of file shadervar.h.
References csColor::blue, csColor::green, and csColor::red.
bool csShaderVariable::SetValue | ( | const csVector3 & | value | ) | [inline] |
Store a csVector3.
Definition at line 352 of file shadervar.h.
References csVector3::x, csVector3::y, and csVector3::z.
bool csShaderVariable::SetValue | ( | const csVector2 & | value | ) | [inline] |
Store a csVector2.
Definition at line 343 of file shadervar.h.
References csVector2::x, and csVector2::y.
bool csShaderVariable::SetValue | ( | iRenderBuffer * | value | ) | [inline] |
bool csShaderVariable::SetValue | ( | iTextureWrapper * | value | ) | [inline] |
bool csShaderVariable::SetValue | ( | iTextureHandle * | value | ) | [inline] |
bool csShaderVariable::SetValue | ( | const csRGBpixel & | value | ) | [inline] |
Store a color.
Definition at line 308 of file shadervar.h.
References csRGBpixel::alpha, csRGBpixel::blue, csRGBpixel::green, and csRGBpixel::red.
bool csShaderVariable::SetValue | ( | float | value | ) | [inline] |
bool csShaderVariable::SetValue | ( | int | value | ) | [inline] |
The documentation for this class was generated from the following file:
- csgfx/shadervar.h
Generated for Crystal Space by doxygen 1.4.7