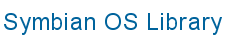
![]() |
![]() |
|
CVideoPlayerUtility
provides a simple interface to
open, play and obtain information from sampled video data. The video data can
supplied either in a file, a descriptor or a URL.
For the purposes of this description, the playing process has been broken down into the following sections.
Opening - describes the
options available for opening video clips after the
CVideoPlayerUtility
object has been instantiated.
Configuration - describes the various configuration settings such as volume and balance settings that can be queried or set.
Plug-in Control - describes the information and commands that can be sent to or retrieved from plug-in controllers.
Meta data control - describes the controls available for meta data manipulation.
Playing - describes the controls available for playing video clips.
In the same way that CMdaAudioPlayerUtility
provides methods for playing more than one audio file within a single instance,
CVideoPlayerUtility
provides methods to play multiple
video clips within a single instance. A noticeable difference however, is that
video clips cannot be opened during instantiation of the
CVideoPlayerUtility
object. Instead, all video clips must
be opened using one of the open methods listed below after the player object
has been created.
The following open methods are provided:
Open statements for opening video clips after the player object has been created.
OpenFileL(const TDesC& aFileName, TUid aControllerUid=KNullUid);
OpenDesL(const TDesC8& aDescriptor, TUid aControllerUid=KNullUid);
OpenUrlL(const TDesC& aUrl, TInt aIapId = KUseDefaultIap, const TDesC8& aMimeType=KNullDesC8, TUid aControllerUid=KNullUid);
Open statements for opening and automatically playing video clips after the player object has been created.
OpenAndPlayFileL(const TDesC& aFileName, TUid aControllerUid=KNullUid);
OpenAndPlayDesL(const TDesC8& aDescriptor, TUid aControllerUid=KNullUid);
OpenAndPlayUrlL(const TDesC& aUrl, TInt aIapId = KUseDefaultIap, const TDesC8& aMimeType=KNullDesC8, TUid aControllerUid=KNullUid);
As soon as the open method has completed, successfully or otherwise,
the callback function
MVideoPlayerUtilityObserver::MvpuoOpenComplete()
is
called.
If a video clip is already open and playing, use
Stop()
followed by Close()
to unload
the video clip before specifying new video data to open.
Following the opening of the video clip, configuration adjustments can be made using the methods described below.
The configuration related methods are:
General - covers the setting and reporting of playback priorities in relation to other applications that may be running, the detection of audio tracks within video clips, the detection of frame sizes and the setting of the display window. The specific methods are:
SetPriorityL()
- sets the priority of the
video/audio device which is used to arbitrate between multiple objects trying
to access a controller.
PriorityL()
- get the priority setting of
the video/audio device.
AudioEnabledL()
- determines whether an
audio track is present within the video clip.
VideoFrameSizeL()
- get the current frame
size of the video clip.
SetDisplayWindowL()
- set the display
window to use when playing back the video clip.
MIME type and codecs - covers the reporting of MIME type and codecs for video and audio data that is already open.
The MIME type of the video clip currently open can be retrieved
using VideoFormatMimeType()
.
The codecs used by the video clip and possible audio track can
be retrieved using VideoTypeL()
and
AudioTypeL()
respectively.
Note: the presence of an audio track within a video clip can be
determined using AudioEnabledL()
.
Bit and frame rates - covers the reporting of the bit and frame rate settings for video clips and possible audio tracks.
The bit rate of the video data can be retrieved using
VideoBitRateL()
.
The bit rate of a possible audio track can be retrieved using
AudioBitRateL()
.
The video frame rate in frames per second can be retrieved
using VideoFrameRateL()
.
Note: the presence of an audio track within a video clip can be
determined using AudioEnabledL()
.
Volume and gain - covers the reporting and setting of
the volume levels for audio tracks belonging to video clips. The methods for
controlling volume settings for playback are Volume()
,
SetVolumeL()
and MaxVolume()
.
Balance - covers the reporting and setting of the audio playback balance settings (if an audio track exists within the video clip).
The balance of a possible audio track can be set using
SetBalanceL()
.
The balance setting of a possible audio track can be retrieved
using Balance()
.
You can govern the relationship with a video plug-n in various ways:
ControllerImplementationInformationL()
-
allows applications to query implementation information about the controller
plugin in use.
CustomCommandSync()
- allows applications to
send custom commands to controller plugins.
RegisterForVideoLoadingNotification()
-
registers to receive notifications of video clip loading/rebuffering.
GetVideoLoadingProgressL()
- gets the progress
of video clip loading/rebuffering.
Some video formats enable the use of meta data, enabling the player of a clip to retrieve information that is held within the clip itself. This meta data is usually used to store information such as copyright information, creator, creation date and so on.
CVideoPlayerUtility
provides two meta data methods
that enable you to:
Get the number of meta data entries in a clip
withNumberOfMetaDataEntriesL()
.
Retrieve a specific meta data entry within a clip with
MetaDataEntryL()
.
Note: it is not possible to set meta data using this class. If you need
to set meta data, use CVideoRecorderUtility
instead.
This class is intended for playing video clips only. As with
CMdaAudioPlayerUtility
, the
CVideoPlayerUtility
is capable of playing more than one
clip within the same instance of the object.
The play related methods are:
Play()
- start playback of video data from the
current position. When playing of the video sample is complete, successfully or
otherwise, the callback function
MVideoPlayerUtilityObserver::MvpuoPlayComplete()
is
called.
You can also specify a start and endpoint in the videofile to be
played using Play(const TTimeIntervalMicroSeconds& aStartPoint, const
TTimeIntervalMicroSeconds& aEndPoint)
.
PauseL()
enables you to pause playback; the
position within the video clip is maintained in case a subsequent
Play()
is issued.
Stop()
stops playback of the video sample as
soon as is possible and calls the callback function
MVideoPlayerUtilityObserver::MvpuoPlayComplete()
. If
playback is already complete, this function has no effect.
Close()
closes all related controllers.
DurationL()
- determine the duration of the
video clip in microseconds.
PositionL()
and
SetPositionL()
retrieves or sets the current playing
position within the video clip.
RegisterForVideoLoadingNotification()
-
activate callbacks that report on video rebuffering. The callback used for this
mechanism are, MVideoLoadingObserver::MvloLoadingStarted()
and MVideoLoadingObserver::MvloLoadingComplete()
.
GetFrame()
- uses the
MVideoPlayerUtilityObserver::MvpuoFrameReady()
callback to
return a bitmap of the frame currently being played.
RefreshFrameL();
- re-draw the current frame.
Multi Media Framework Client Overview - general overview of the various components of the MMF Client API and the functionality they provide.
Audio Recording, Conversion and Playing - advanced audio file manipulation features; specifically, the ability to record, convert and playback sound clips as well as to manipulate meta data.
Audio Recording - introduction to the audio recording class.
Audio Conversion - introduction to the audio conversion class.
Audio Playing - introduction to the audio playing class.
Audio Streaming - the interface to streaming sampled audio.
Audio Tone Player - a simple interface for tone generation (synthesized sounds) that is supported on all audio-capable devices.
Video Recording and Playing - video manipulation features; specifically, the ability to record and playback video clips as well as to manipulate meta data.
Video Recording - introduction to the video recording class.
Video Playing - introduction to the video playing class.