static function DisplayWizard.<T> (title : string) : T
Parameters
Name | Description |
T |
The class implementing the wizard. It has to derive from ScriptableWizard.
|
title |
The title shown at the top of the wizard window.
|
Returns
T - The wizard.
Description
Creates a wizard.
When the user hits the Create button OnWizardCreate function will be called.
DisplayWizard will only show one wizard for every wizard class.
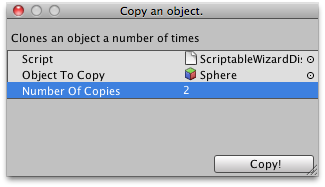
Simple Wizard Window that copies a GameObject several times.
using UnityEngine;
using UnityEditor;
using System.Collections;
public class ScriptableWizardDisplayWizard : ScriptableWizard {
public GameObject ObjectToCopy = null;
public int numberOfCopies = 2;
[
MenuItem (
"Example/Show DisplayWizard usage")]
static void CreateWindow() {
ScriptableWizard.DisplayWizard(
"Copy an object.",
typeof(ScriptableWizardDisplayWizard),
"Copy!");
}
void OnWizardUpdate() {
helpString =
"Clones an object a number of times";
if(!ObjectToCopy) {
errorString =
"Please assign an object";
isValid =
false;
}
else {
errorString =
"";
isValid = true;
}
}
void OnWizardCreate () {
for(
int i = 0; i < numberOfCopies; i++)
Instantiate(ObjectToCopy,
Vector3.zero,
Quaternion.identity);
}
}