static function PrefixLabel (totalPosition : Rect, id : int, label : GUIContent) : Rect
Parameters
Name | Description |
totalPosition |
Rectangle on the screen to use in total for both the label and the control.
|
id |
The unique ID of the control.
|
label |
Label to show in front of the control.
|
Returns
Rect - Rectangle on the screen to use just for the control itself.
Description
Make a label in front of some control.
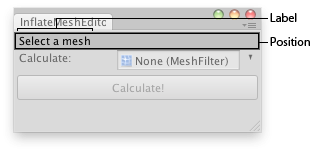
Prefix Label in an Editor Window.
class InflateMeshEditor
extends EditorWindow {
var object :
MeshFilter;
@
MenuItem(
"Examples/Inflate Mesh")
static function Init() {
var window = GetWindow(InflateMeshEditor);
window.Show();
}
function OnGUI() {
EditorGUI.PrefixLabel(
Rect(3,3,position.width-6, 15),
0,
GUIContent(
"Select a mesh"));
object =
EditorGUI.ObjectField(
Rect(3,20,position.width-6,20),
"Calculate:",
object,
MeshFilter);
GUI.enabled = object? true :
false;
if(
GUI.Button(
Rect(3,45,position.width-6,25),
"Calculate!"))
Calculate();
}
function Calculate() {
var finalNormals =
new Vector3[0];
var mesh = object.sharedMesh;
var vertices = mesh.vertices;
var normals = mesh.normals;
var vertexIDs =
new int[vertices.length];
var counter :
int = 0;
for (
var i = 0; i < vertices.length; i++) {
for (
var j = 0; j < vertices.length; j++) {
if(vertexIDs[i] == 0) {
counter++;
vertexIDs[i] = counter;
}
if(i != j)
if(vertices[i] == vertices[j] && vertices[i] != 0)
vertexIDs[j] = vertexIDs[i];
}
}
finalNormals = normals;
calculated = 0.5;
for(
var k = 1; k <= counter; k++) {
var curAvgNormal :
Vector3 =
Vector3.zero;
for(
var l = 0; l < vertexIDs.length; l++)
if(vertexIDs[l] == k) {
curAvgNormal += normals[l];
}
curAvgNormal.Normalize();
for(
var m = 0; m < vertexIDs.length; m++)
if(vertexIDs[m] == k)
finalNormals[m] = curAvgNormal;
}
object.gameObject.AddComponent(
"InflateMesh").fNormals = finalNormals;
Debug.Log(
"Done Adding Component, press play and see your mesh being inflated!");
}
}
And the script attached to the editor script:
private var mesh :
Mesh;
private var vertices =
new Vector3[0];
private var normals =
new Vector3[0];
var fNormals =
new Vector3[0];
var increaseRatio = 0.005;
function Start () {
mesh = GetComponent(
MeshFilter).mesh;
vertices = mesh.vertices;
normals = mesh.normals;
}
function Update () {
for (
var i = 0; i < vertices.length; i++) {
vertices[i] += fNormals[i] *
Time.deltaTime * increaseRatio;
}
mesh.vertices = vertices;
}