static function EnumPopup (position : Rect, label : GUIContent, selected : System.Enum, style : GUIStyle = EditorStyles.popup) : System.Enum
Parameters
Name | Description |
position |
Rectangle on the screen to use for the field.
|
label |
Optional label in front of the field.
|
selected |
The enum option the field shows.
|
style |
Optional GUIStyle.
|
Returns
System.Enum - The enum option that has been selected by the user.
Description
Make an enum popup selection field.
Takes the currently selected enum value as a parameter and returns the enum value selected by the user.
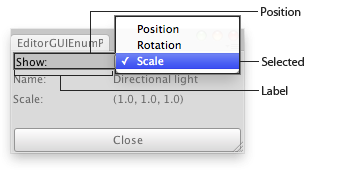
Enum Popup in an Editor Window.
enum OPTIONS {
Position = 0,
Rotation = 1,
Scale = 2,
}
class EditorGUIEnumPopup
extends EditorWindow {
var display : OPTIONS = OPTIONS.Position;
@
MenuItem(
"Examples/Editor GUI Enum Popup usage")
static function Init() {
var window = GetWindow(EditorGUIEnumPopup);
window.position =
Rect(0, 0, 220, 80);
window.Show();
}
function OnGUI() {
var selectedObj :
Transform =
Selection.activeTransform;
display =
EditorGUI.EnumPopup(
Rect(3,3,position.width - 6, 15),
"Show:",
display);
EditorGUI.LabelField(
Rect(0, 20, position.width,15),
"Name:",
selectedObj ? selectedObj.name :
"Select an Object");
if(selectedObj) {
switch(display) {
case OPTIONS.Position:
EditorGUI.LabelField(
Rect(0, 40, position.width,15),
"Position:",
selectedObj.position.ToString());
break;
case OPTIONS.Rotation:
EditorGUI.LabelField(
Rect(0, 40, position.width,15),
"Rotation:",
selectedObj.rotation.ToString());
break;
case OPTIONS.Scale:
EditorGUI.LabelField(
Rect(0, 40, position.width,15),
"Scale:",
selectedObj.localScale.ToString());
break;
default:
Debug.LogError(
"Unrecognized Option");
break;
}
}
if(
GUI.Button(
Rect(3,position.height - 25, position.width - 6, 24),
"Close"))
this.Close();
}
}