Parameters
Name | Description |
position |
Rectangle on the screen to use for the field.
|
label |
Optional label to display in front of the field.
|
value |
The curve to edit.
|
color |
The color to show the curve with.
|
ranges |
Optional rectangle that the curve is restrained within.
|
Returns
AnimationCurve - The curve edited by the user.
Description
Make a field for editing an AnimationCurve.
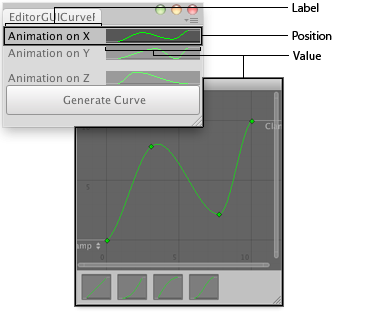
Curve field in an Editor Window.
class EditorGUICurveField
extends EditorWindow {
var curveX :
AnimationCurve =
AnimationCurve.Linear(0,0,10,10);
var curveY :
AnimationCurve =
AnimationCurve.Linear(0,0,10,10);
var curveZ :
AnimationCurve =
AnimationCurve.Linear(0,0,10,10);
@
MenuItem(
"Examples/Create Curve For Object")
static function Init() {
var window = GetWindow(EditorGUICurveField);
window.position =
Rect(0,0,200,100);
window.Show();
}
function OnGUI() {
curveX =
EditorGUI.CurveField(
Rect(3,3,position.width-6,15),
"Animation on X", curveX);
curveY =
EditorGUI.CurveField(
Rect(3,20,position.width-6,15),
"Animation on Y", curveY);
curveZ =
EditorGUI.CurveField(
Rect(3,45,position.width-6,15),
"Animation on Z", curveZ);
if(
GUI.Button(
Rect(3,60,position.width-6,30),
"Generate Curve"))
AddCurveToSelectedGameObject();
}
function AddCurveToSelectedGameObject() {
if(
Selection.activeGameObject) {
var comp : FollowAnimationCurve =
Selection.activeGameObject.AddComponent(FollowAnimationCurve);
comp.SetCurves(curveX, curveY, curveZ);
}
else {
Debug.LogError(
"No Game Object selected for adding an animation curve");
}
}
}
And the script attached to this editor script: