static var depth : int
Description
The sorting depth of the currently executing GUI behaviour.
Set this to determine ordering when you have different scripts running simultaneously.
Note:To see this example working, you will need to create 2
scripts. Remember to name the scripts with the same name as the class
names, else it will not work.
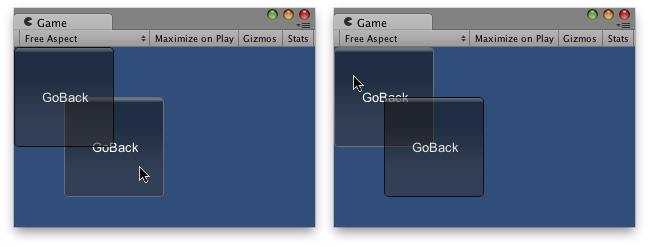
One Button behind the other.
using UnityEngine;
using System.Collections;
public class example1 :
MonoBehaviour {
public static int guiDepth = 0;
void OnGUI() {
GUI.depth = guiDepth;
if (
GUI.RepeatButton(
new Rect(0, 0, 100, 100),
"GoBack")) {
guiDepth = 1;
example2.guiDepth = 0;
}
}
}
public class example :
MonoBehaviour {
}
And copy this other example to another script:
using UnityEngine;
using System.Collections;
public class example2 :
MonoBehaviour {
public static int guiDepth = 1;
void OnGUI() {
GUI.depth = guiDepth;
if (
GUI.RepeatButton(
new Rect(50, 50, 100, 100),
"GoBack")) {
guiDepth = 1;
example1.guiDepth = 0;
}
}
}
public class example :
MonoBehaviour {
}