iSyntaxService Struct Reference
[Loading & Saving support]
This component provides services for other loaders to easily parse properties of standard CS world syntax.
More...
#include <imap/services.h>
Inheritance diagram for iSyntaxService:
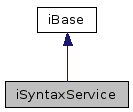
Public Member Functions | |
virtual bool | HandlePortalParameter (iDocumentNode *child, iLoaderContext *ldr_context, uint32 &flags, bool &mirror, bool &warp, int &msv, csMatrix3 &m, csVector3 &before, csVector3 &after, iString *destSector, bool &handled, bool &autoresolve)=0 |
Handles a common portal parameter. | |
virtual bool | ParseAlphaMode (iDocumentNode *node, iStringSet *strings, csAlphaMode &alphaMode, bool allowAutoMode=true)=0 |
Parse an alphamode description. | |
virtual bool | ParseBool (iDocumentNode *node, bool &result, bool def_result)=0 |
Parse the value of this node and return a boolean depending on this value. | |
virtual bool | ParseBoolAttribute (iDocumentNode *node, const char *attrname, bool &result, bool def_result, bool required)=0 |
Parse the value of an attribute of this node and return a boolean depending on this value. | |
virtual bool | ParseBox (iDocumentNode *node, csOBB &b)=0 |
Parse a box description. | |
virtual bool | ParseBox (iDocumentNode *node, csBox3 &v)=0 |
Parse a box description. | |
virtual bool | ParseColor (iDocumentNode *node, csColor4 &c)=0 |
Parse a color description. | |
virtual bool | ParseColor (iDocumentNode *node, csColor &c)=0 |
Parse a color description. | |
virtual bool | ParseGradient (iDocumentNode *node, iGradient *gradient)=0 |
Parse a color gradient. | |
virtual bool | ParseKey (iDocumentNode *node, iKeyValuePair *&keyvalue)=0 |
Parse a key definition. | |
virtual bool | ParseMatrix (iDocumentNode *node, csMatrix3 &m)=0 |
Parse a matrix description. | |
virtual bool | ParseMixmode (iDocumentNode *node, uint &mixmode, bool allowFxMesh=false)=0 |
Parse a mixmode description. | |
virtual bool | ParsePlane (iDocumentNode *node, csPlane3 &p)=0 |
Parse a plane description. | |
virtual csRef< iRenderBuffer > | ParseRenderBuffer (iDocumentNode *node)=0 |
Parse a user render buffer. | |
virtual csRef< iShader > | ParseShaderRef (iLoaderContext *ldr_context, iDocumentNode *node)=0 |
Parse a node that is a reference to a shader. | |
virtual bool | ParseShaderVar (iLoaderContext *ldr_context, iDocumentNode *node, csShaderVariable &var)=0 |
Parse a shader variable declaration. | |
virtual csRef< iShaderVariableAccessor > | ParseShaderVarExpr (iDocumentNode *node)=0 |
Parse a shader variable expression. | |
virtual bool | ParseVector (iDocumentNode *node, csVector2 &v)=0 |
Parse a vector description. | |
virtual bool | ParseVector (iDocumentNode *node, csVector3 &v)=0 |
Parse a vector description. | |
virtual bool | ParseZMode (iDocumentNode *node, csZBufMode &zmode, bool allowZmesh=false)=0 |
Attempt to parse a zmode from node. | |
virtual bool | WriteAlphaMode (iDocumentNode *node, iStringSet *strings, const csAlphaMode &alphaMode)=0 |
Write an alphamode description. | |
bool | WriteBool (iDocumentNode *node, const char *name, bool value, bool default_value) |
Write a node representing the value of the boolean, if it differs from a provided default value. | |
virtual bool | WriteBool (iDocumentNode *node, const char *name, bool value)=0 |
Write a node representing the value of the boolean. | |
virtual bool | WriteBox (iDocumentNode *node, const csOBB &b)=0 |
Write a box description. | |
virtual bool | WriteBox (iDocumentNode *node, const csBox3 &v)=0 |
Write a box description. | |
virtual bool | WriteColor (iDocumentNode *node, const csColor4 &c)=0 |
Write a color description. | |
virtual bool | WriteColor (iDocumentNode *node, const csColor &c)=0 |
Write a color description. | |
virtual bool | WriteGradient (iDocumentNode *node, iGradient *gradient)=0 |
Write a color gradient. | |
virtual bool | WriteKey (iDocumentNode *node, iKeyValuePair *keyvalue)=0 |
Write a key definition and add the key to the given object, Returns true if successful. | |
virtual bool | WriteMatrix (iDocumentNode *node, const csMatrix3 &m)=0 |
Write a matrix description. | |
virtual bool | WriteMixmode (iDocumentNode *node, uint mixmode, bool allowFxMesh)=0 |
Write a mixmode description. | |
virtual bool | WritePlane (iDocumentNode *node, const csPlane3 &p)=0 |
Write a plane description. | |
virtual bool | WriteRenderBuffer (iDocumentNode *node, iRenderBuffer *buffer)=0 |
Write a render buffer. | |
virtual bool | WriteShaderVar (iDocumentNode *node, csShaderVariable &var)=0 |
Write a shader variable declaration. | |
virtual bool | WriteVector (iDocumentNode *node, const csVector2 &v)=0 |
Write a vector description. | |
virtual bool | WriteVector (iDocumentNode *node, const csVector3 &v)=0 |
Write a vector description. | |
virtual bool | WriteZMode (iDocumentNode *node, csZBufMode zmode, bool allowZmesh)=0 |
Write a ZMode description. | |
Parse reporting helpers | |
virtual void | Report (const char *msgid, int severity, iDocumentNode *errornode, const char *msg,...)=0 |
Report something, also gives a path in the XML tree. | |
virtual void | ReportBadToken (iDocumentNode *badtokennode)=0 |
Report a bad token. | |
virtual void | ReportError (const char *msgid, iDocumentNode *errornode, const char *msg,...)=0 |
Report an error and also gives a path in the XML tree. |
Detailed Description
This component provides services for other loaders to easily parse properties of standard CS world syntax.
Definition at line 73 of file services.h.
Member Function Documentation
virtual bool iSyntaxService::HandlePortalParameter | ( | iDocumentNode * | child, | |
iLoaderContext * | ldr_context, | |||
uint32 & | flags, | |||
bool & | mirror, | |||
bool & | warp, | |||
int & | msv, | |||
csMatrix3 & | m, | |||
csVector3 & | before, | |||
csVector3 & | after, | |||
iString * | destSector, | |||
bool & | handled, | |||
bool & | autoresolve | |||
) | [pure virtual] |
Handles a common portal parameter.
flags: contains all flags found in the description. Returns false on failure. Returns false in 'handled' if it couldn't understand the token.
virtual bool iSyntaxService::ParseAlphaMode | ( | iDocumentNode * | node, | |
iStringSet * | strings, | |||
csAlphaMode & | alphaMode, | |||
bool | allowAutoMode = true | |||
) | [pure virtual] |
Parse an alphamode description.
Returns true if successful.
virtual bool iSyntaxService::ParseBool | ( | iDocumentNode * | node, | |
bool & | result, | |||
bool | def_result | |||
) | [pure virtual] |
Parse the value of this node and return a boolean depending on this value.
The following mapping happens (case insensitive):
- 1 -> true
- 0 -> false
- yes -> true
- no -> false
- true -> true
- false -> false
- on -> true
- off -> false
- (empty value) -> (def_result)
- (everyting else) -> error
virtual bool iSyntaxService::ParseBoolAttribute | ( | iDocumentNode * | node, | |
const char * | attrname, | |||
bool & | result, | |||
bool | def_result, | |||
bool | required | |||
) | [pure virtual] |
Parse the value of an attribute of this node and return a boolean depending on this value.
The following mapping happens (case insensitive):
- 1 -> true
- 0 -> false
- yes -> true
- no -> false
- true -> true
- false -> false
- on -> true
- off -> false
- (empty value) -> (def_result)
- (everyting else) -> error
- Parameters:
-
node Document node with the attribute to parse. attrname Name of the attribute. result Returns the result. def_result Default result value. required if this is true then not having the attribute will result in an error. If this is false then not having the attribute will result in the default value.
- Returns:
- Whether the parsing was successful.
false
if an error occured.
virtual bool iSyntaxService::ParseBox | ( | iDocumentNode * | node, | |
csOBB & | b | |||
) | [pure virtual] |
Parse a box description.
Returns true if successful.
virtual bool iSyntaxService::ParseBox | ( | iDocumentNode * | node, | |
csBox3 & | v | |||
) | [pure virtual] |
Parse a box description.
Returns true if successful.
virtual bool iSyntaxService::ParseColor | ( | iDocumentNode * | node, | |
csColor4 & | c | |||
) | [pure virtual] |
Parse a color description.
Returns true if successful.
virtual bool iSyntaxService::ParseColor | ( | iDocumentNode * | node, | |
csColor & | c | |||
) | [pure virtual] |
Parse a color description.
Returns true if successful.
virtual bool iSyntaxService::ParseGradient | ( | iDocumentNode * | node, | |
iGradient * | gradient | |||
) | [pure virtual] |
Parse a color gradient.
- Parameters:
-
node Document node containing the gradient data. gradient Valid pointer to a gradient interface which is filled with the data from the document node.
virtual bool iSyntaxService::ParseKey | ( | iDocumentNode * | node, | |
iKeyValuePair *& | keyvalue | |||
) | [pure virtual] |
Parse a key definition.
A iKeyValuePair instance is return in "keyvalue", with refcount 1 Returns true if successful.
virtual bool iSyntaxService::ParseMatrix | ( | iDocumentNode * | node, | |
csMatrix3 & | m | |||
) | [pure virtual] |
Parse a matrix description.
Returns true if successful.
virtual bool iSyntaxService::ParseMixmode | ( | iDocumentNode * | node, | |
uint & | mixmode, | |||
bool | allowFxMesh = false | |||
) | [pure virtual] |
Parse a mixmode description.
Returns true if successful.
virtual bool iSyntaxService::ParsePlane | ( | iDocumentNode * | node, | |
csPlane3 & | p | |||
) | [pure virtual] |
Parse a plane description.
Returns true if successful.
virtual csRef<iRenderBuffer> iSyntaxService::ParseRenderBuffer | ( | iDocumentNode * | node | ) | [pure virtual] |
Parse a user render buffer.
virtual csRef<iShader> iSyntaxService::ParseShaderRef | ( | iLoaderContext * | ldr_context, | |
iDocumentNode * | node | |||
) | [pure virtual] |
Parse a node that is a reference to a shader.
Those nodes look like <nodename name="shadername" file="/path/to/shader.xml" />
. First, the shader manager is queried for a shader of the name specified in the name
attribute. If this failed, the shader is attempted to be loaded from the file
specified. Note that if the name appearing in the shader file and the name of the name
attribute mismatches, this method fails (and the loaded shader is not registered with the shader manager),
virtual bool iSyntaxService::ParseShaderVar | ( | iLoaderContext * | ldr_context, | |
iDocumentNode * | node, | |||
csShaderVariable & | var | |||
) | [pure virtual] |
Parse a shader variable declaration.
virtual csRef<iShaderVariableAccessor> iSyntaxService::ParseShaderVarExpr | ( | iDocumentNode * | node | ) | [pure virtual] |
Parse a shader variable expression.
Returns an acessor that can be set on a shader variable. The accessor subsequently evaluates the expression.
virtual bool iSyntaxService::ParseVector | ( | iDocumentNode * | node, | |
csVector2 & | v | |||
) | [pure virtual] |
Parse a vector description.
Returns true if successful.
virtual bool iSyntaxService::ParseVector | ( | iDocumentNode * | node, | |
csVector3 & | v | |||
) | [pure virtual] |
Parse a vector description.
Returns true if successful.
virtual bool iSyntaxService::ParseZMode | ( | iDocumentNode * | node, | |
csZBufMode & | zmode, | |||
bool | allowZmesh = false | |||
) | [pure virtual] |
Attempt to parse a zmode from node.
allowZmesh specifies whether ZMESH and ZMESH2 zmodes should be saved to zmode or rejected, causing the method to fail and return 'false'.
- Remarks:
- As z modes usually appear "in between" other document nodes, this function does not report an error if the token isn't recognized, but only returns 'false'.
virtual void iSyntaxService::Report | ( | const char * | msgid, | |
int | severity, | |||
iDocumentNode * | errornode, | |||
const char * | msg, | |||
... | ||||
) | [pure virtual] |
Report something, also gives a path in the XML tree.
virtual void iSyntaxService::ReportBadToken | ( | iDocumentNode * | badtokennode | ) | [pure virtual] |
Report a bad token.
This is a convenience function which will eventually call ReportError().
virtual void iSyntaxService::ReportError | ( | const char * | msgid, | |
iDocumentNode * | errornode, | |||
const char * | msg, | |||
... | ||||
) | [pure virtual] |
Report an error and also gives a path in the XML tree.
virtual bool iSyntaxService::WriteAlphaMode | ( | iDocumentNode * | node, | |
iStringSet * | strings, | |||
const csAlphaMode & | alphaMode | |||
) | [pure virtual] |
Write an alphamode description.
Returns true if successful.
bool iSyntaxService::WriteBool | ( | iDocumentNode * | node, | |
const char * | name, | |||
bool | value, | |||
bool | default_value | |||
) | [inline] |
Write a node representing the value of the boolean, if it differs from a provided default value.
Definition at line 152 of file services.h.
References WriteBool().
virtual bool iSyntaxService::WriteBool | ( | iDocumentNode * | node, | |
const char * | name, | |||
bool | value | |||
) | [pure virtual] |
virtual bool iSyntaxService::WriteBox | ( | iDocumentNode * | node, | |
const csOBB & | b | |||
) | [pure virtual] |
Write a box description.
Returns true if successful.
virtual bool iSyntaxService::WriteBox | ( | iDocumentNode * | node, | |
const csBox3 & | v | |||
) | [pure virtual] |
Write a box description.
Returns true if successful.
virtual bool iSyntaxService::WriteColor | ( | iDocumentNode * | node, | |
const csColor4 & | c | |||
) | [pure virtual] |
Write a color description.
Returns true if successful.
virtual bool iSyntaxService::WriteColor | ( | iDocumentNode * | node, | |
const csColor & | c | |||
) | [pure virtual] |
Write a color description.
Returns true if successful.
virtual bool iSyntaxService::WriteGradient | ( | iDocumentNode * | node, | |
iGradient * | gradient | |||
) | [pure virtual] |
Write a color gradient.
virtual bool iSyntaxService::WriteKey | ( | iDocumentNode * | node, | |
iKeyValuePair * | keyvalue | |||
) | [pure virtual] |
Write a key definition and add the key to the given object, Returns true if successful.
virtual bool iSyntaxService::WriteMatrix | ( | iDocumentNode * | node, | |
const csMatrix3 & | m | |||
) | [pure virtual] |
Write a matrix description.
Returns true if successful.
virtual bool iSyntaxService::WriteMixmode | ( | iDocumentNode * | node, | |
uint | mixmode, | |||
bool | allowFxMesh | |||
) | [pure virtual] |
Write a mixmode description.
Returns true if successful.
virtual bool iSyntaxService::WritePlane | ( | iDocumentNode * | node, | |
const csPlane3 & | p | |||
) | [pure virtual] |
Write a plane description.
Returns true if successful.
virtual bool iSyntaxService::WriteRenderBuffer | ( | iDocumentNode * | node, | |
iRenderBuffer * | buffer | |||
) | [pure virtual] |
Write a render buffer.
virtual bool iSyntaxService::WriteShaderVar | ( | iDocumentNode * | node, | |
csShaderVariable & | var | |||
) | [pure virtual] |
Write a shader variable declaration.
virtual bool iSyntaxService::WriteVector | ( | iDocumentNode * | node, | |
const csVector2 & | v | |||
) | [pure virtual] |
Write a vector description.
Returns true if successful.
virtual bool iSyntaxService::WriteVector | ( | iDocumentNode * | node, | |
const csVector3 & | v | |||
) | [pure virtual] |
Write a vector description.
Returns true if successful.
virtual bool iSyntaxService::WriteZMode | ( | iDocumentNode * | node, | |
csZBufMode | zmode, | |||
bool | allowZmesh | |||
) | [pure virtual] |
Write a ZMode description.
Returns true if successful.
The documentation for this struct was generated from the following file:
- imap/services.h
Generated for Crystal Space by doxygen 1.4.7