iSector Struct Reference
[Crystal Space 3D Engine]
The iSector interface is used to work with "sectors".
More...
#include <iengine/sector.h>
Inheritance diagram for iSector:
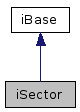
Public Member Functions | |
virtual iShaderVariableContext * | GetSVContext ()=0 |
Get the shader variable context for this sector. | |
virtual iObject * | QueryObject ()=0 |
Get the iObject for this sector. | |
Light culling | |
virtual void | AddLightVisibleCallback (iLightVisibleCallback *cb)=0 |
Add a callback that is called whenever a light is visible. | |
virtual bool | IsLightCullingEnabled () const =0 |
Return true if light culling objects are enabled. | |
virtual void | RemoveLightVisibleCallback (iLightVisibleCallback *cb)=0 |
Remove a light visible callback. | |
virtual void | SetLightCulling (bool enable)=0 |
Set/reset culling objects for all lights in the sector. | |
Mesh handling | |
virtual void | AddSectorMeshCallback (iSectorMeshCallback *cb)=0 |
Add a mesh callback. | |
virtual iMeshList * | GetMeshes ()=0 |
Get the list of meshes in this sector. | |
virtual const csSet< csPtrKey< iMeshWrapper > > & | GetPortalMeshes () const =0 |
Get the set of meshes containing portals that leave from this sector. | |
virtual csRenderMeshList * | GetVisibleMeshes (iRenderView *)=0 |
Get a set of visible meshes for given camera. | |
virtual void | RemoveSectorMeshCallback (iSectorMeshCallback *cb)=0 |
Remove a mesh callback. | |
virtual void | UnlinkObjects ()=0 |
Unlink all mesh objects from this sector. | |
Visculling | |
virtual void | CalculateSectorBBox (csBox3 &bbox, bool do_meshes) const =0 |
Calculate the bounding box of all objects in this sector. | |
virtual void | CheckFrustum (iFrustumView *lview)=0 |
Check visibility in a frustum way for all things and polygons in this sector and possibly traverse through portals to other sectors. | |
virtual iSector * | FollowSegment (csReversibleTransform &t, csVector3 &new_position, bool &mirror, bool only_portals=false)=0 |
Follow a segment starting at this sector. | |
virtual iVisibilityCuller * | GetVisibilityCuller ()=0 |
Get the visibility culler that is used for this sector. | |
virtual csSectorHitBeamResult | HitBeam (const csVector3 &start, const csVector3 &end, bool accurate=false)=0 |
Follow a beam from start to end and return the first object that is hit. | |
virtual iMeshWrapper * | HitBeam (const csVector3 &start, const csVector3 &end, csVector3 &intersect, int *polygon_idx, bool accurate=false)=0 |
Follow a beam from start to end and return the first object that is hit. | |
virtual csSectorHitBeamResult | HitBeamPortals (const csVector3 &start, const csVector3 &end)=0 |
Follow a beam from start to end and return the first polygon that is hit. | |
virtual iMeshWrapper * | HitBeamPortals (const csVector3 &start, const csVector3 &end, csVector3 &isect, int *polygon_idx, iSector **final_sector=0)=0 |
Follow a beam from start to end and return the first polygon that is hit. | |
virtual bool | SetVisibilityCullerPlugin (const char *name, iDocumentNode *culler_params=0)=0 |
Use the specified plugin as the visibility culler for this sector. | |
Mesh generator handling | |
virtual iMeshGenerator * | CreateMeshGenerator (const char *name)=0 |
Create a mesh generator. | |
virtual iMeshGenerator * | GetMeshGenerator (size_t idx)=0 |
Get the specific mesh generator. | |
virtual iMeshGenerator * | GetMeshGeneratorByName (const char *name)=0 |
Get the specific mesh generator by name. | |
virtual size_t | GetMeshGeneratorCount () const =0 |
Get the number of mesh generators. | |
virtual void | RemoveMeshGenerator (size_t idx)=0 |
Remove a mesh generator. | |
virtual void | RemoveMeshGenerators ()=0 |
Remove all mesh generators. | |
Drawing related | |
virtual void | DecRecLevel ()=0 |
Remove one draw recursion level. | |
virtual void | Draw (iRenderView *rview)=0 |
Draw the sector with the given render view. | |
virtual int | GetRecLevel () const =0 |
Get the current draw recursion level. | |
virtual iRenderLoop * | GetRenderLoop ()=0 |
Get the renderloop for this sector. | |
virtual void | IncRecLevel ()=0 |
Add one draw recursion level. | |
virtual void | PrepareDraw (iRenderView *rview)=0 |
Prepare the sector to draw. | |
virtual void | SetRenderLoop (iRenderLoop *rl)=0 |
Set the renderloop to use for this sector. | |
Fog handling | |
virtual void | DisableFog ()=0 |
Disable fog in this sector. | |
virtual const csFog & | GetFog () const =0 |
Return the fog structure (even if fog is disabled). | |
virtual bool | HasFog () const =0 |
Has this sector fog? | |
virtual void | SetFog (const csFog &fog)=0 |
Set a fog structure directly. | |
virtual void | SetFog (float density, const csColor &color)=0 |
Fill the fog structure with the given values. | |
Light handling | |
virtual csColor | GetDynamicAmbientLight () const =0 |
Get the last set dynamic ambient light for this sector. | |
virtual uint | GetDynamicAmbientVersion () const =0 |
Get the version number of the dynamic ambient color. | |
virtual iLightList * | GetLights ()=0 |
Get the list of static and pseudo-dynamic lights in this sector. | |
virtual void | SetDynamicAmbientLight (const csColor &color)=0 |
Sets dynamic ambient light this sector. | |
virtual void | ShineLights (iMeshWrapper *)=0 |
Version of ShineLights() which only affects one mesh object. | |
virtual void | ShineLights ()=0 |
Calculate lighting for all objects in this sector. | |
Sector callbacks | |
virtual iSectorCallback * | GetSectorCallback (int idx) const =0 |
Get the specified sector callback. | |
virtual int | GetSectorCallbackCount () const =0 |
Get the number of sector callbacks. | |
virtual void | RemoveSectorCallback (iSectorCallback *cb)=0 |
Remove a sector callback. | |
virtual void | SetSectorCallback (iSectorCallback *cb)=0 |
Set the sector callback. |
Detailed Description
The iSector interface is used to work with "sectors".A "sector" is an empty region of space that can contain other objects (mesh objects). A sector itself does not represent geometry but only contains other geometry. A sector does contain lights though. The sector is the basic building block for any Crystal Space level. A level can be made from one or more sectors. Using the thing mesh object one can use portals to connect multiple sectors.
Main creators of instances implementing this interface:
Main ways to get pointers to this interface:
- iEngine::FindSector()
- iSectorList::Get()
- iSectorList::FindByName()
- iLoaderContext::FindSector()
- iPortal::GetSector()
- iCamera::GetSector()
Main users of this interface:
Definition at line 187 of file sector.h.
Member Function Documentation
virtual void iSector::AddLightVisibleCallback | ( | iLightVisibleCallback * | cb | ) | [pure virtual] |
Add a callback that is called whenever a light is visible.
This only works if SetLightCulling() is enabled.
virtual void iSector::AddSectorMeshCallback | ( | iSectorMeshCallback * | cb | ) | [pure virtual] |
virtual void iSector::CalculateSectorBBox | ( | csBox3 & | bbox, | |
bool | do_meshes | |||
) | const [pure virtual] |
Calculate the bounding box of all objects in this sector.
This function is not very efficient as it will traverse all objects in the sector one by one and compute a bounding box from that.
virtual void iSector::CheckFrustum | ( | iFrustumView * | lview | ) | [pure virtual] |
Check visibility in a frustum way for all things and polygons in this sector and possibly traverse through portals to other sectors.
virtual iMeshGenerator* iSector::CreateMeshGenerator | ( | const char * | name | ) | [pure virtual] |
Create a mesh generator.
virtual void iSector::DecRecLevel | ( | ) | [pure virtual] |
Remove one draw recursion level.
virtual void iSector::DisableFog | ( | ) | [pure virtual] |
Disable fog in this sector.
virtual void iSector::Draw | ( | iRenderView * | rview | ) | [pure virtual] |
Draw the sector with the given render view.
virtual iSector* iSector::FollowSegment | ( | csReversibleTransform & | t, | |
csVector3 & | new_position, | |||
bool & | mirror, | |||
bool | only_portals = false | |||
) | [pure virtual] |
Follow a segment starting at this sector.
If the segment intersects with a polygon it will stop there unless the polygon is a portal in which case it will recursively go to that sector (possibly applying warping transformations) and continue there.
This routine will modify all the given parameters to reflect space warping. These should be used as the new camera transformation when you decide to really go to the new position.
This function returns the resulting sector and new_position will be set to the last position that you can go to before hitting a wall.
If only_portals is true then only portals will be checked. This means that intersection with normal polygons is not checked. This is a lot faster but it does mean that you need to use another collision detection system to test with walls.
virtual csColor iSector::GetDynamicAmbientLight | ( | ) | const [pure virtual] |
Get the last set dynamic ambient light for this sector.
virtual uint iSector::GetDynamicAmbientVersion | ( | ) | const [pure virtual] |
Get the version number of the dynamic ambient color.
This number is increased whenever dynamic ambient changes.
virtual const csFog& iSector::GetFog | ( | ) | const [pure virtual] |
Return the fog structure (even if fog is disabled).
virtual iLightList* iSector::GetLights | ( | ) | [pure virtual] |
Get the list of static and pseudo-dynamic lights in this sector.
virtual iMeshList* iSector::GetMeshes | ( | ) | [pure virtual] |
Get the list of meshes in this sector.
virtual iMeshGenerator* iSector::GetMeshGenerator | ( | size_t | idx | ) | [pure virtual] |
Get the specific mesh generator.
virtual iMeshGenerator* iSector::GetMeshGeneratorByName | ( | const char * | name | ) | [pure virtual] |
Get the specific mesh generator by name.
virtual size_t iSector::GetMeshGeneratorCount | ( | ) | const [pure virtual] |
Get the number of mesh generators.
virtual const csSet<csPtrKey<iMeshWrapper> >& iSector::GetPortalMeshes | ( | ) | const [pure virtual] |
Get the set of meshes containing portals that leave from this sector.
Note that portals are uni-directional. The portals represented by this list are portals that are on some mesh object that is actually located in this sector.
virtual int iSector::GetRecLevel | ( | ) | const [pure virtual] |
Get the current draw recursion level.
virtual iRenderLoop* iSector::GetRenderLoop | ( | ) | [pure virtual] |
Get the renderloop for this sector.
If this returns 0 then it means there is no specific renderloop for this sector. In that case the default renderloop in the engine will be used.
virtual iSectorCallback* iSector::GetSectorCallback | ( | int | idx | ) | const [pure virtual] |
Get the specified sector callback.
virtual int iSector::GetSectorCallbackCount | ( | ) | const [pure virtual] |
Get the number of sector callbacks.
virtual iShaderVariableContext* iSector::GetSVContext | ( | ) | [pure virtual] |
Get the shader variable context for this sector.
virtual iVisibilityCuller* iSector::GetVisibilityCuller | ( | ) | [pure virtual] |
Get the visibility culler that is used for this sector.
If there is no culler yet a culler of type 'crystalspace.culling.frustvis' will be created and used for this sector.
virtual csRenderMeshList* iSector::GetVisibleMeshes | ( | iRenderView * | ) | [pure virtual] |
Get a set of visible meshes for given camera.
These will be cached for a given frame and camera, but if the cached result isn't enough it will be reculled. The returned pointer is valid as long as the sector exists (the sector will delete it)
virtual bool iSector::HasFog | ( | ) | const [pure virtual] |
Has this sector fog?
virtual csSectorHitBeamResult iSector::HitBeam | ( | const csVector3 & | start, | |
const csVector3 & | end, | |||
bool | accurate = false | |||
) | [pure virtual] |
Follow a beam from start to end and return the first object that is hit.
In case it is a thing the polygon_idx field will be filled with the indices of the polygon that was hit. If polygon_idx is null then the polygon will not be filled in. This function doesn't support portals.
- See also:
- csSectorHitBeamResult
virtual iMeshWrapper* iSector::HitBeam | ( | const csVector3 & | start, | |
const csVector3 & | end, | |||
csVector3 & | intersect, | |||
int * | polygon_idx, | |||
bool | accurate = false | |||
) | [pure virtual] |
Follow a beam from start to end and return the first object that is hit.
In case it is a thing the polygon_idx field will be filled with the indices of the polygon that was hit. If polygon_idx is null then the polygon will not be filled in. This function doesn't support portals.
- Deprecated:
- Use the csSectorHitBeamResult version instead
virtual csSectorHitBeamResult iSector::HitBeamPortals | ( | const csVector3 & | start, | |
const csVector3 & | end | |||
) | [pure virtual] |
Follow a beam from start to end and return the first polygon that is hit.
This function correctly traverse portals and space warping portals. Normally the sector you call this on should be the sector containing the 'start' point. 'isect' will be the intersection point if a polygon is returned. This function returns -1 if no polygon was hit or the polygon index otherwise.
- See also:
- csSectorHitBeamResult
virtual iMeshWrapper* iSector::HitBeamPortals | ( | const csVector3 & | start, | |
const csVector3 & | end, | |||
csVector3 & | isect, | |||
int * | polygon_idx, | |||
iSector ** | final_sector = 0 | |||
) | [pure virtual] |
Follow a beam from start to end and return the first polygon that is hit.
This function correctly traverse portals and space warping portals. Normally the sector you call this on should be the sector containing the 'start' point. 'isect' will be the intersection point if a polygon is returned. This function returns -1 if no polygon was hit or the polygon index otherwise.
- Deprecated:
- Use the csSectorHitBeamResult version instead
virtual void iSector::IncRecLevel | ( | ) | [pure virtual] |
Add one draw recursion level.
virtual bool iSector::IsLightCullingEnabled | ( | ) | const [pure virtual] |
Return true if light culling objects are enabled.
virtual void iSector::PrepareDraw | ( | iRenderView * | rview | ) | [pure virtual] |
Prepare the sector to draw.
Must be called before any rendermesh is requested.
virtual void iSector::RemoveLightVisibleCallback | ( | iLightVisibleCallback * | cb | ) | [pure virtual] |
Remove a light visible callback.
virtual void iSector::RemoveMeshGenerator | ( | size_t | idx | ) | [pure virtual] |
Remove a mesh generator.
virtual void iSector::RemoveMeshGenerators | ( | ) | [pure virtual] |
Remove all mesh generators.
virtual void iSector::RemoveSectorCallback | ( | iSectorCallback * | cb | ) | [pure virtual] |
Remove a sector callback.
virtual void iSector::RemoveSectorMeshCallback | ( | iSectorMeshCallback * | cb | ) | [pure virtual] |
Remove a mesh callback.
virtual void iSector::SetDynamicAmbientLight | ( | const csColor & | color | ) | [pure virtual] |
Sets dynamic ambient light this sector.
This works in addition to the dynamic light you can specify for every object.
virtual void iSector::SetFog | ( | const csFog & | fog | ) | [pure virtual] |
Set a fog structure directly.
virtual void iSector::SetFog | ( | float | density, | |
const csColor & | color | |||
) | [pure virtual] |
Fill the fog structure with the given values.
virtual void iSector::SetLightCulling | ( | bool | enable | ) | [pure virtual] |
Set/reset culling objects for all lights in the sector.
This can be used for hardware accelerated lighting techniques that want to know what lights (influence object) are visible for camera. With this enabled every light will be registered to the culler of this sector and a callback (see AddLightVisibleCallback) will be called.
virtual void iSector::SetRenderLoop | ( | iRenderLoop * | rl | ) | [pure virtual] |
Set the renderloop to use for this sector.
If this is not set then the default engine renderloop will be used.
virtual void iSector::SetSectorCallback | ( | iSectorCallback * | cb | ) | [pure virtual] |
virtual bool iSector::SetVisibilityCullerPlugin | ( | const char * | name, | |
iDocumentNode * | culler_params = 0 | |||
) | [pure virtual] |
Use the specified plugin as the visibility culler for this sector.
Returns false if the culler could not be loaded for some reason. The optional culler parameters will be given to the new visibility culler.
virtual void iSector::ShineLights | ( | iMeshWrapper * | ) | [pure virtual] |
Version of ShineLights() which only affects one mesh object.
virtual void iSector::ShineLights | ( | ) | [pure virtual] |
Calculate lighting for all objects in this sector.
virtual void iSector::UnlinkObjects | ( | ) | [pure virtual] |
Unlink all mesh objects from this sector.
This will not remove the mesh objects but simply unlink them so that they are no longer part of this sector. This happens automatically when the sector is removed but you can force it by calling this function here.
The documentation for this struct was generated from the following file:
- iengine/sector.h
Generated for Crystal Space by doxygen 1.4.7