static function Label (position : Vector3, content : GUIContent, style : GUIStyle) : void
Parameters
Name | Description |
position |
Position in 3D space as seen from the current handle camera.
|
text |
Text to display on the label.
|
image |
Texture to display on the label.
|
content |
Text, image and tooltip for this label.
|
style |
The style to use. If left out, the label style from the current GUISkin is used. Note: Use HandleUtility.GetHandleSize where you might want to have constant screen-sized handles.
|
Description
Make a text label positioned in 3D space.
Labels have no user interaction, do not catch mouse clicks and are always rendered in normal style.
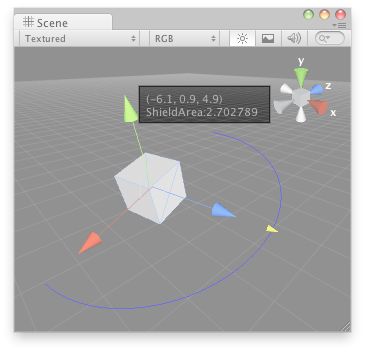
Label in the Scene View.
@
CustomEditor (DummyLabelScript)
class LabelHandle
extends Editor {
function OnSceneGUI () {
Handles.color =
Color.blue;
Handles.Label(target.transform.position +
Vector3.up*2,
target.transform.position.ToString() +
"\nShieldArea: " +
target.shieldArea.ToString());
Handles.BeginGUI(
Rect(
Screen.width - 100,
Screen.height - 80, 90,50));
Handles.DrawWireArc(target.transform.position,
target.transform.up,
-target.transform.right,
180,
target.shieldArea);
target.shieldArea =
Handles.ScaleValueHandle(target.shieldArea,
target.transform.position + target.transform.forward*target.shieldArea,
target.transform.rotation,
1,
Handles.ConeCap,
1);
}
}
And the script attached to this Handle:
var shieldArea : float = 5;